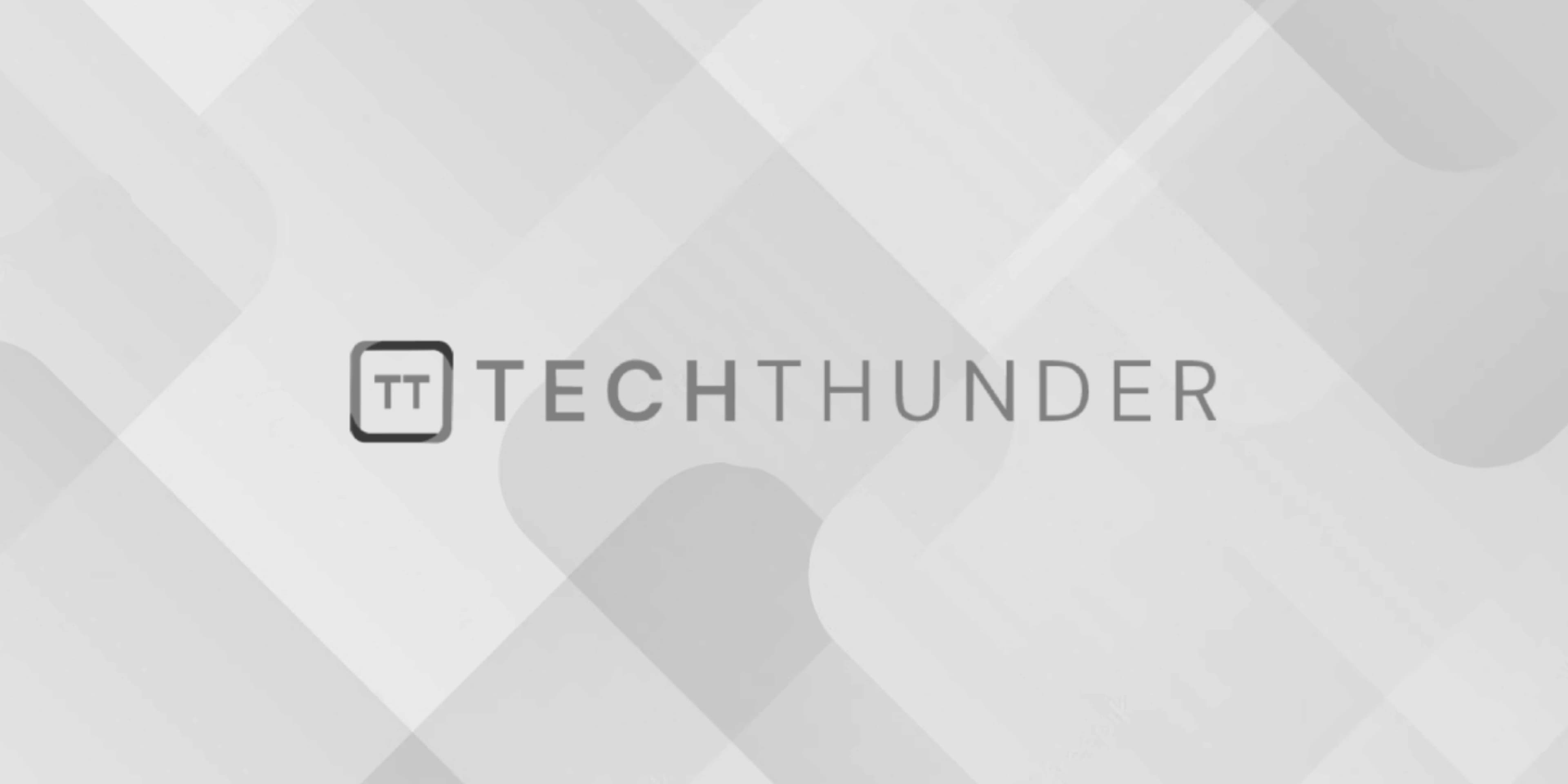
Spring Security Tutorial
The step-by-step tutorial for implementing Spring Security in a Spring Boot application. This tutorial will cover basic security configuration, user authentication, authorization, and a simple login/logout feature.
Step 1: Create a Spring Boot Project
Start by creating a new Spring Boot project using Spring Initializr or your preferred development environment.
Step 2: Configure Spring Security
Create a security configuration class that extends WebSecurityConfigurerAdapter
.
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/", "/home").permitAll()
.anyRequest().authenticated()
.and()
.formLogin()
.loginPage("/login")
.permitAll()
.and()
.logout()
.permitAll();
}
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER")
.and()
.withUser("admin").password(passwordEncoder().encode("adminpassword")).roles("ADMIN");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
Step 3: Create Login and Logout Pages
Create Thymeleaf templates for the login and logout pages.
src/main/resources/templates/login.html
src/main/resources/templates/logout.html
Step 4: Create Controllers
Create controllers for the login and logout pages.
@Controller
public class LoginController {
@GetMapping("/login")
public String login() {
return "login";
}
}
@Controller
public class LogoutController {
@GetMapping("/logout")
public String logout() {
return "logout";
}
}
Step 5: Create Home Page
Create a simple home page template.
src/main/resources/templates/home.html
Step 6: Run the Application
Run your Spring Boot application and navigate to http://localhost:8080/home
. You can access the login page by going to http://localhost:8080/login
.
This tutorial provides a basic introduction to setting up Spring Security in a Spring Boot application. Keep in mind that for a production environment, you should consider more advanced security features such as user authentication against a database, role-based access control, securing APIs, and more. The above code should be a starting point for understanding the setup and how different components interact in Spring Security.