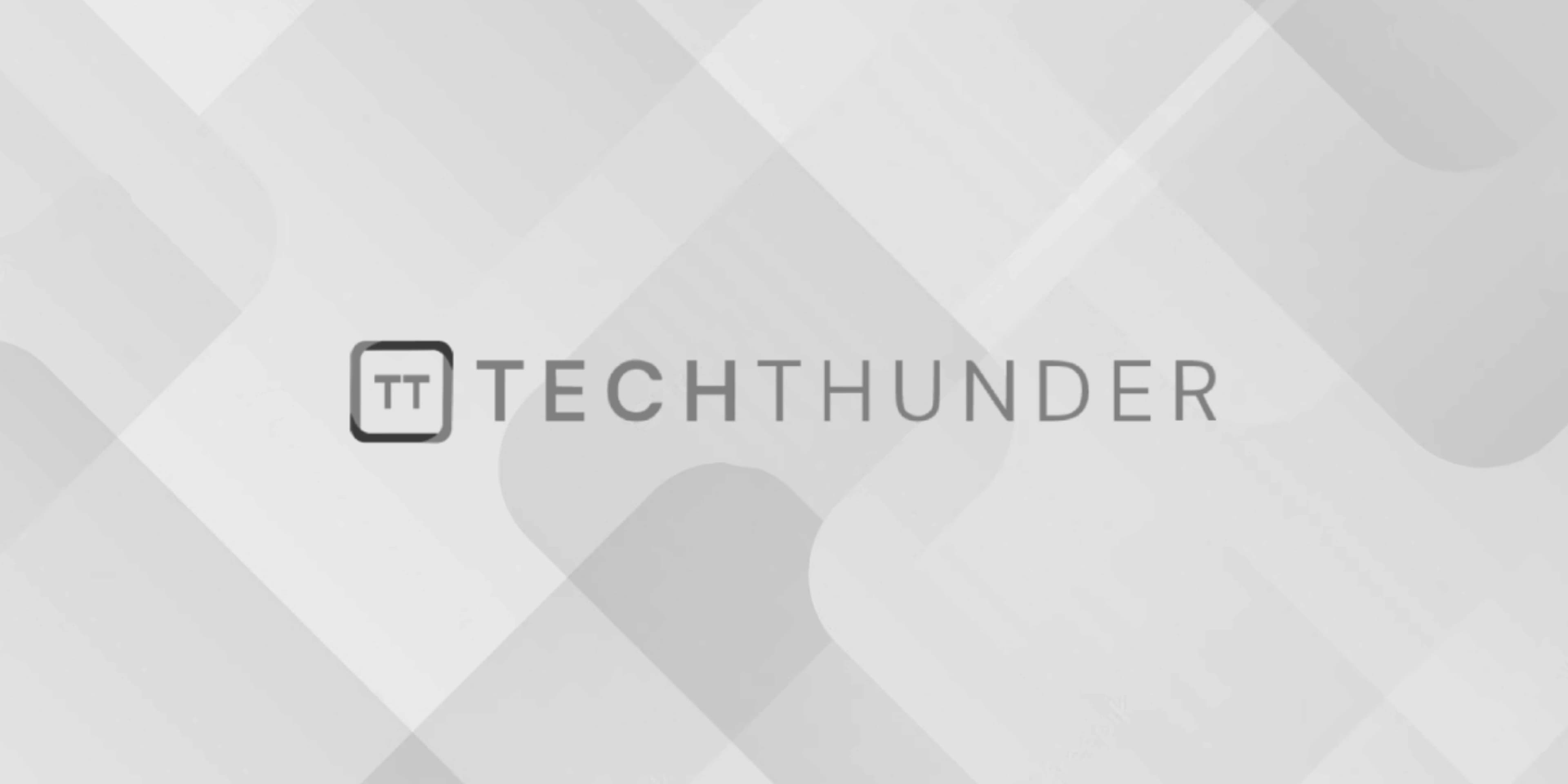
Java Abstract class
The Java abstract class is a class that cannot be instantiated on its own and is meant to be subclassed by other classes. Abstract classes are used to define a common interface and provide a partial implementation for their subclasses. They are particularly useful when you want to create a blueprint for a group of related classes.
Here are the key characteristics and rules associated with abstract classes in Java:
- Declaring an Abstract Class:
- You define an abstract class using the
abstract
keyword in its class declaration. - An abstract class can have fields (variables) and methods, just like a regular class.
- Abstract Methods:
- Abstract classes can include abstract methods. These are methods that are declared without providing an implementation (i.e., no method body).
- Abstract methods are marked with the
abstract
keyword, and they end with a semicolon instead of a method body. - Subclasses of an abstract class must provide concrete (i.e., non-abstract) implementations for all abstract methods defined in the abstract class.
- Concrete Methods:
- Abstract classes can also include concrete (i.e., fully implemented) methods.
- Subclasses can inherit these concrete methods, and they can also override or extend them as needed.
- Cannot Be Instantiated:
- You cannot create instances of an abstract class using the
new
keyword. An abstract class is meant to be subclassed, and objects are created from its concrete subclasses.
- Inheritance:
- Subclasses of an abstract class must implement all abstract methods or be declared as abstract classes themselves.
Here’s an example of an abstract class in Java:
abstract class Shape {
int x, y; // Fields for coordinates
// Abstract method to calculate the area (to be implemented by subclasses)
abstract double calculateArea();
// Concrete method to display the coordinates
void displayCoordinates() {
System.out.println("Coordinates: (" + x + ", " + y + ")");
}
}
class Circle extends Shape {
double radius;
Circle(int x, int y, double radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
@Override
double calculateArea() {
return Math.PI * radius * radius;
}
}
class Rectangle extends Shape {
double width, height;
Rectangle(int x, int y, double width, double height) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
}
@Override
double calculateArea() {
return width * height;
}
}
In this example, Shape
is an abstract class with one abstract method calculateArea()
and a concrete method displayCoordinates()
. Circle
and Rectangle
are concrete subclasses of Shape
that provide specific implementations for the calculateArea()
method.
Abstract classes are commonly used to define common behavior or contracts for a group of related classes, while allowing for individual customization in concrete subclasses.