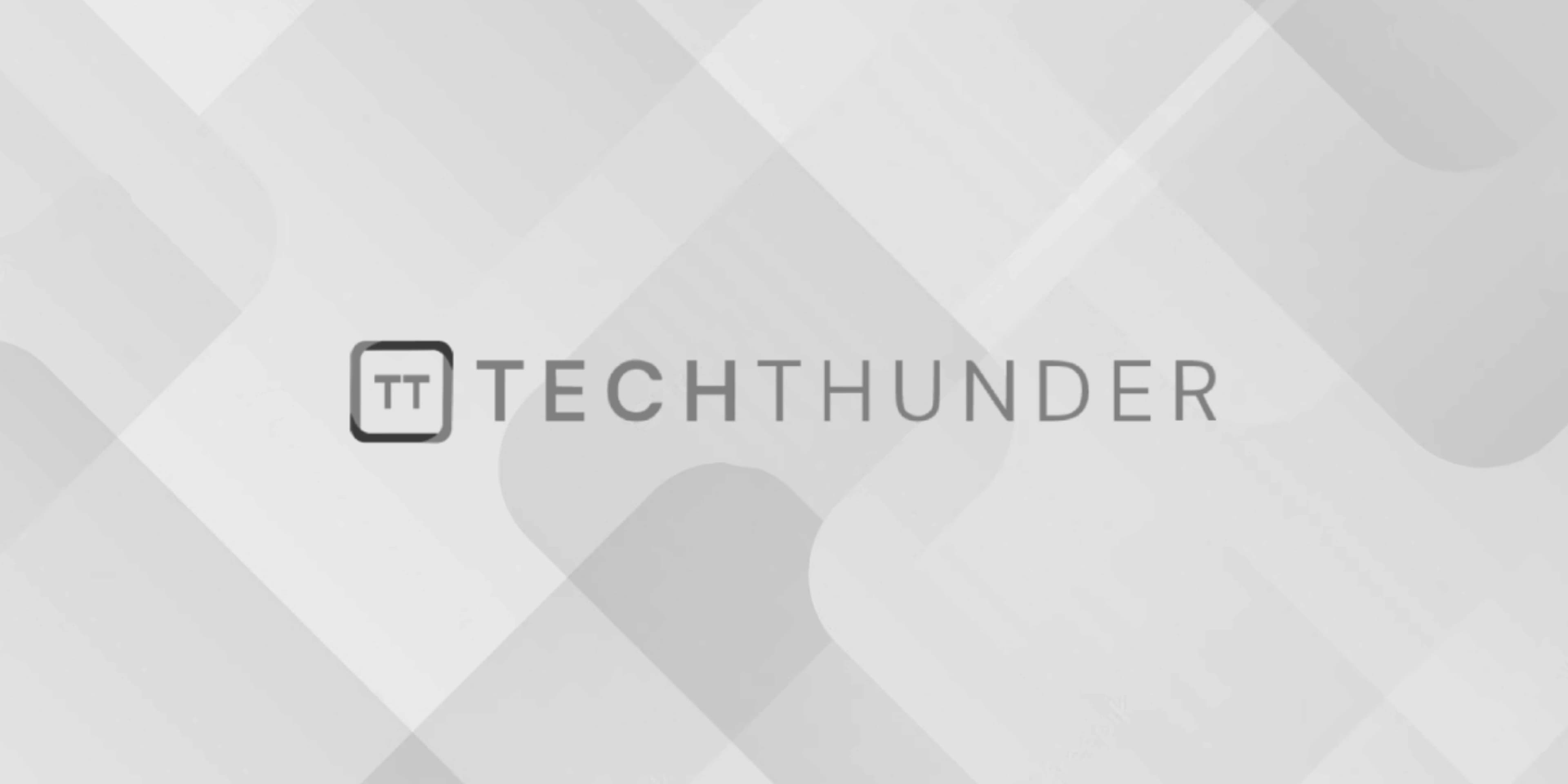
Java AWT & Events
Java AWT (Abstract Window Toolkit) is a set of classes and APIs provided by Java to create graphical user interface (GUI) components for building desktop applications. AWT provides the foundation for creating windows, dialogs, buttons, menus, and other graphical elements in Java applications. It is an older GUI framework compared to Java Swing and JavaFX.
Here are some key concepts and components related to Java AWT and events:
- Components: AWT provides a range of UI components, such as buttons, labels, text fields, checkboxes, radio buttons, lists, and more.
- Layout Managers: AWT includes layout managers that help you arrange components within containers, ensuring proper alignment and resizing across different platforms and resolutions.
- Containers: Containers are components that can hold and manage other components. Examples of containers are
Frame
,Panel
,Window
, andApplet
. - Events and Event Handling: Events are user or system actions that trigger some kind of response in a GUI application. AWT supports event-driven programming, allowing you to register event listeners to respond to events such as button clicks, mouse actions, and keyboard inputs.
- Event Listeners: Event listeners are interfaces that define callback methods to handle specific types of events. You can attach event listeners to components to respond to user interactions.
- AWT Event Hierarchy: AWT has a hierarchy of event classes that correspond to different types of user actions. Common event classes include
ActionEvent
,MouseEvent
,KeyEvent
, andWindowEvent
. - Event Dispatch Thread (EDT): AWT applications are single-threaded, with the main execution occurring on the Event Dispatch Thread. This thread is responsible for handling user interface events and updating the GUI components.
Here’s a simple example of creating a button and handling its action event:
import java.awt.*;
import java.awt.event.*;
public class AWTExample {
public static void main(String[] args) {
Frame frame = new Frame("AWT Example");
Button button = new Button("Click Me");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.out.println("Button Clicked!");
}
});
frame.add(button);
frame.setLayout(new FlowLayout());
frame.setSize(300, 200);
frame.setVisible(true);
frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
System.exit(0);
}
});
}
}
Keep in mind that AWT has been largely replaced by more modern GUI frameworks like Swing and JavaFX, which offer improved customization, look and feel, and better performance. However, understanding AWT can still be useful when working with legacy applications or when you need a lightweight GUI toolkit for simple applications.