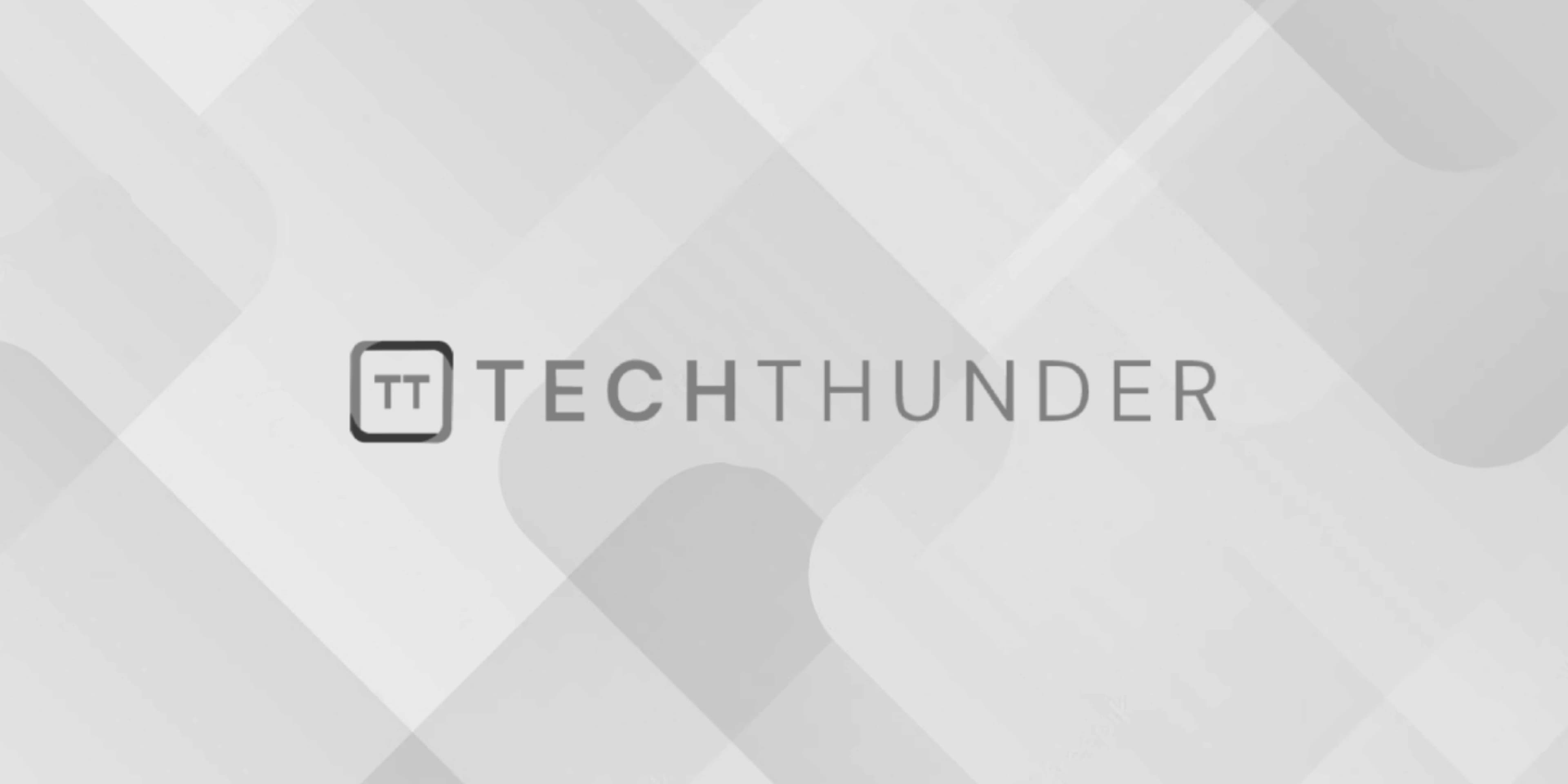
272 views
Java For Loop
The for
loop in Java is a control statement used for repetitive execution of a block of code. It’s often used when you know in advance how many times you want to execute a particular block of code. Here’s the basic syntax of a for
loop in Java:
for (initialization; condition; update) {
// Code to repeat
}
- The
initialization
statement is executed once before the loop begins. It’s typically used to initialize loop control variables. - The
condition
is evaluated before each iteration of the loop. If the condition istrue
, the loop continues; if it’sfalse
, the loop terminates. - The
update
statement is executed after each iteration of the loop. It’s usually used to update loop control variables.
Here’s a simple example that uses a for
loop to print numbers from 1 to 5:
public class Main {
public static void main(String[] args) {
for (int i = 1; i <= 5; i++) {
System.out.println(i);
}
}
}
In this example:
int i = 1
is the initialization step, where we declare and initialize the loop control variablei
to 1.i <= 5
is the condition, which specifies that the loop will continue as long asi
is less than or equal to 5.i++
is the update statement, which incrementsi
by 1 after each iteration.
The loop will execute five times, printing the numbers 1 to 5.
You can use the for
loop for various purposes, such as iterating through arrays, processing data in a list, and performing repetitive tasks with a known number of iterations. The for
loop is a powerful tool for managing repetitive tasks in your Java programs.