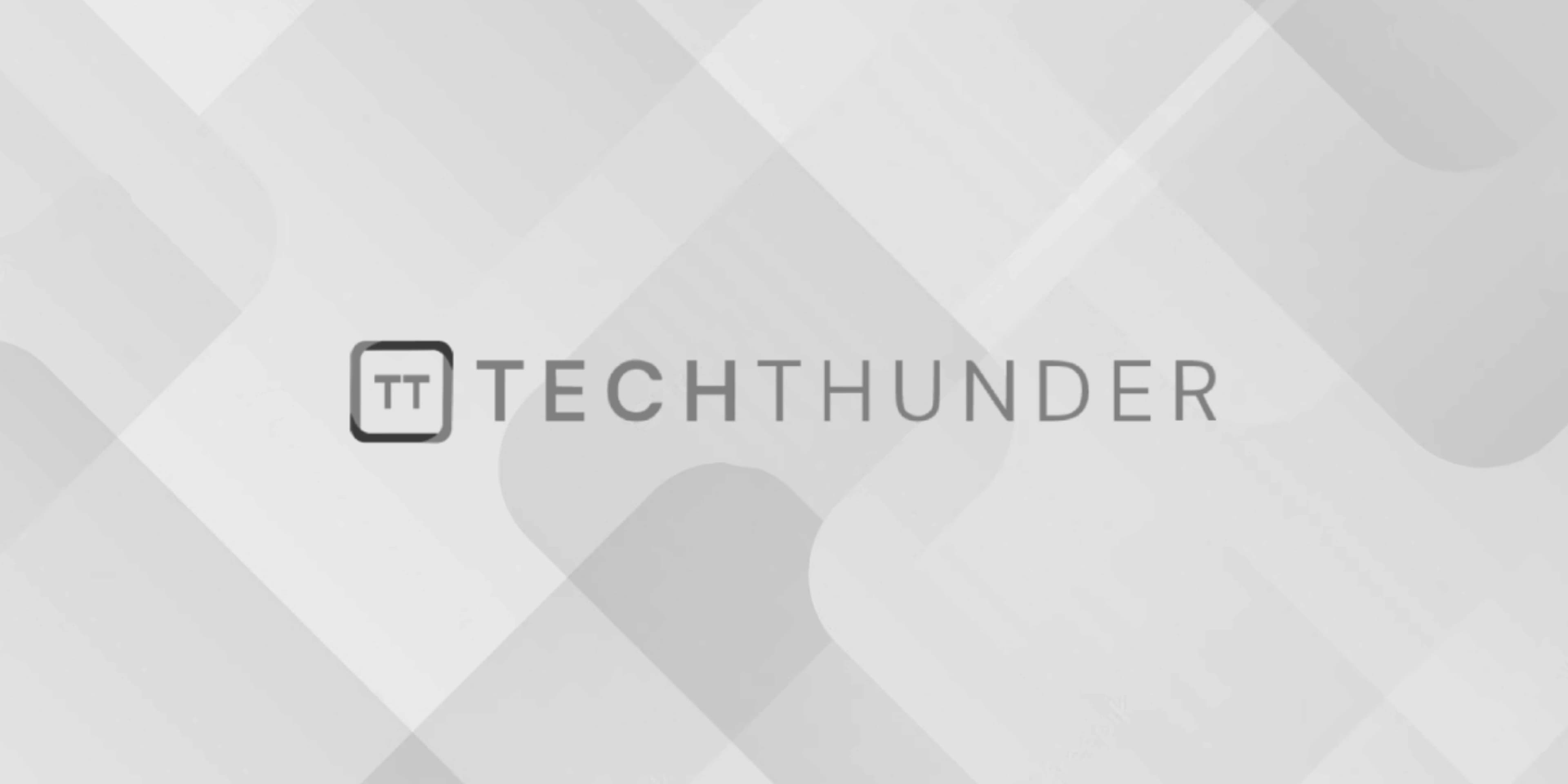
Java Internationalization
Java Internationalization (I18N) refers to the process of designing and developing applications that can adapt to various languages, regions, and cultural conventions. It involves making software products sensitive to differences in language, date and time formats, numeric formats, currency symbols, and other locale-specific attributes.
The Java platform provides comprehensive support for internationalization through its java.util
and java.text
packages. Here are some key concepts and classes related to Java Internationalization:
- Locale: A
Locale
represents a specific language and region combination. It’s used to customize the presentation of data for different locales. - Resource Bundles: A resource bundle is a collection of locale-specific resources, such as text strings, images, and other data. It allows applications to display messages in different languages.
- Message Formatting: The
MessageFormat
class allows you to create strings that include placeholders for variable data. It’s used for constructing localized messages with dynamic values. - Number and Currency Formatting: The
NumberFormat
andCurrencyFormat
classes provide ways to format numbers and currency amounts according to locale-specific rules. - Date and Time Formatting: The
DateFormat
class is used for formatting and parsing dates and times based on locale-specific formats. - Collation: The
Collator
class allows you to compare and sort strings according to the rules of a specific locale. - Time Zone Handling: The
TimeZone
class allows you to work with time zones and convert dates and times across different time zones.
Here’s a simple example of how to use resource bundles for internationalization:
Suppose you have two resource bundle files: messages_en_US.properties
and messages_fr_FR.properties
.
messages_en_US.properties
:
greeting=Hello!
messages_fr_FR.properties
:
greeting=Bonjour !
Java code:
import java.util.Locale;
import java.util.ResourceBundle;
public class InternationalizationExample {
public static void main(String[] args) {
Locale enLocale = new Locale("en", "US");
Locale frLocale = new Locale("fr", "FR");
ResourceBundle enBundle = ResourceBundle.getBundle("messages", enLocale);
ResourceBundle frBundle = ResourceBundle.getBundle("messages", frLocale);
System.out.println("English Greeting: " + enBundle.getString("greeting"));
System.out.println("French Greeting: " + frBundle.getString("greeting"));
}
}
Internationalization is essential for creating software that can be easily localized and adapted to various cultures and languages, making it more accessible to a global audience.