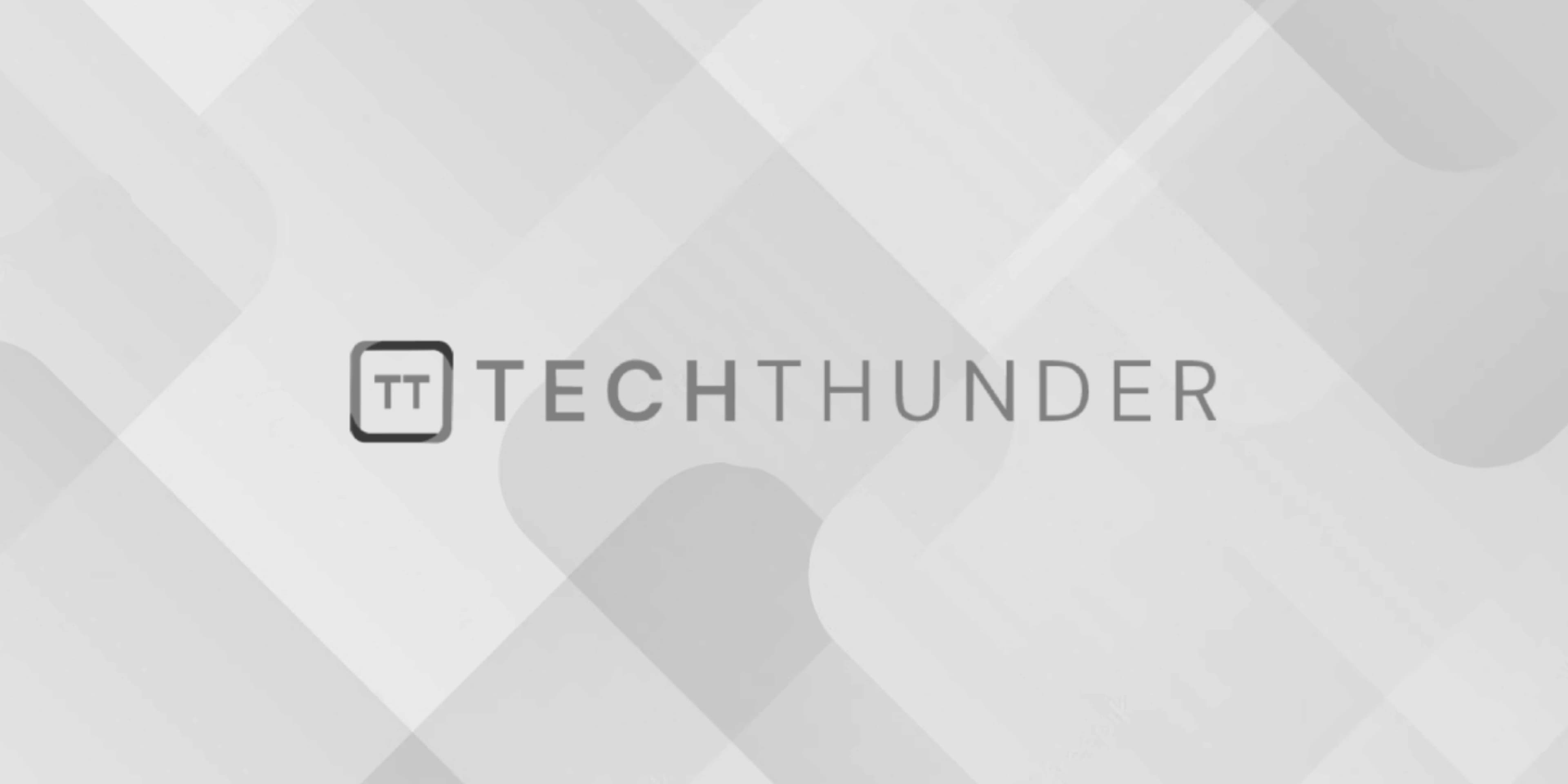
Java Multithreading
Multithreading in Java refers to the concurrent execution of multiple threads within a single Java application. A thread is a lightweight unit of execution that can run independently and concurrently with other threads. Multithreading allows you to perform multiple tasks simultaneously, taking advantage of modern processors with multiple cores.
Java provides a rich set of classes and APIs for multithreading, making it relatively easier to work with threads. Here are the key concepts and components related to multithreading in Java:
- Thread Class: The
Thread
class is the core class used for creating and managing threads. You can extend theThread
class or implement theRunnable
interface to define the code that will run in the thread. - Runnable Interface: The
Runnable
interface defines a single method,run()
, which contains the code that will be executed when the thread starts. - Creating Threads:
- Extending
Thread
class:java class MyThread extends Thread { public void run() { // Thread code here } }
- Implementing
Runnable
interface:java class MyRunnable implements Runnable { public void run() { // Thread code here } }
- Starting Threads: Call the
start()
method on aThread
object to begin its execution. Therun()
method will be executed in a separate thread. - Thread States: Threads have different states such as
NEW
,RUNNABLE
,BLOCKED
,WAITING
,TIMED_WAITING
, andTERMINATED
. - Thread Synchronization: When multiple threads access shared resources, synchronization mechanisms like
synchronized
blocks and methods are used to prevent data corruption and ensure thread safety. - Thread Joining: The
join()
method allows a calling thread to wait for the completion of another thread before proceeding. - Thread Priority: Threads can be assigned priorities using the
setPriority()
method. Higher-priority threads are given preference, but it’s not a guaranteed order of execution. - Thread Pooling: Java provides thread pooling through the
Executor
framework, allowing efficient management of thread resources. - Thread Intercommunication: Threads can communicate and synchronize with each other using methods like
wait()
,notify()
, andnotifyAll()
.
Here’s a basic example of creating and starting threads in Java:
class MyThread extends Thread {
public void run() {
for (int i = 1; i <= 5; i++) {
System.out.println("Thread " + Thread.currentThread().getId() + ": " + i);
}
}
}
public class ThreadExample {
public static void main(String[] args) {
MyThread thread1 = new MyThread();
MyThread thread2 = new MyThread();
thread1.start();
thread2.start();
}
}
Keep in mind that multithreading introduces challenges like synchronization and thread safety. It’s important to understand these challenges and use proper synchronization techniques to avoid issues like race conditions and data inconsistencies.