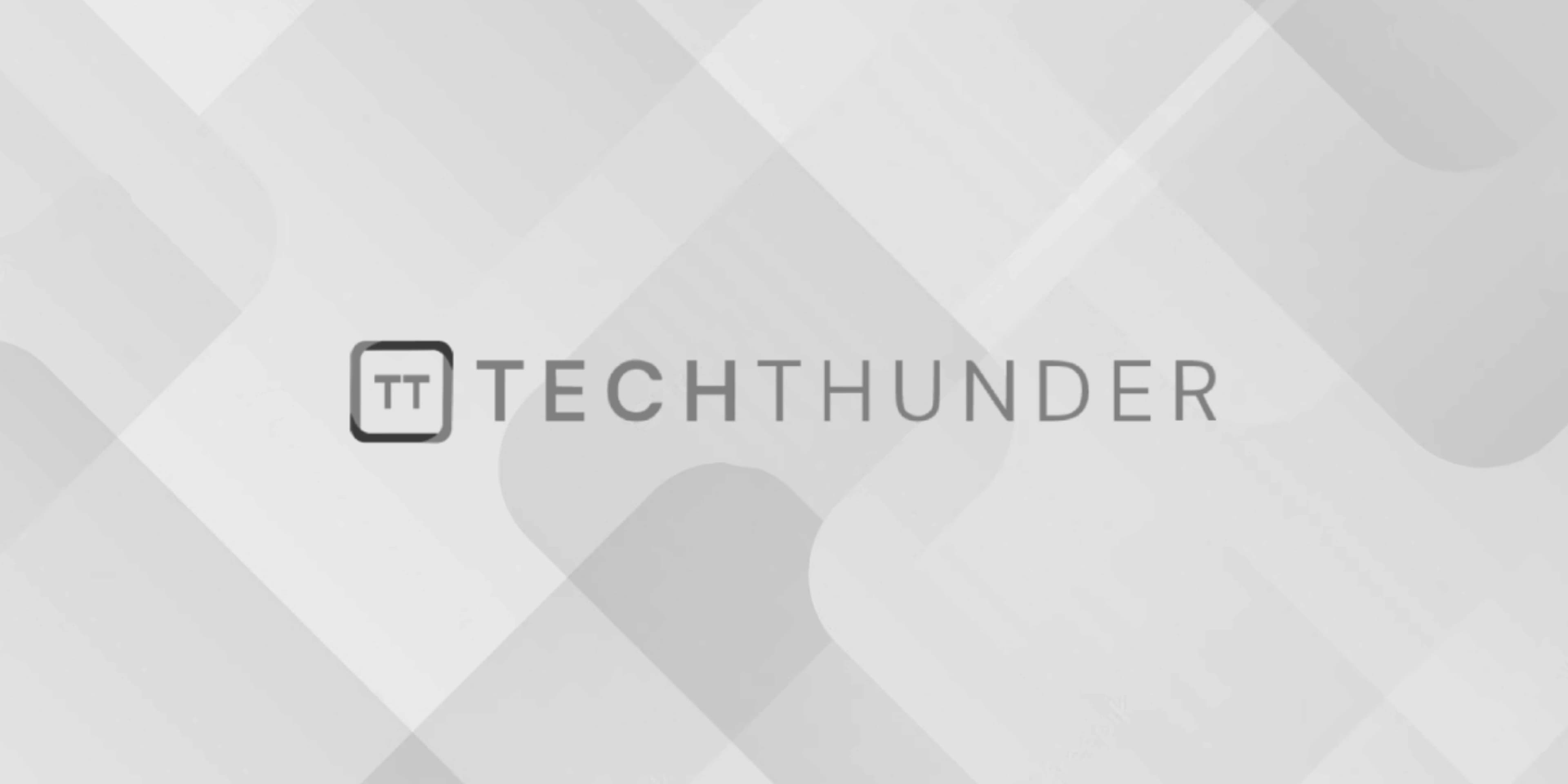
Java Overloading vs Overriding
The Java overloading and overriding are two important concepts related to methods in object-oriented programming. They involve the way methods are defined and used in classes, but they serve different purposes.
- Method Overloading:
- Method overloading is a feature that allows a class to have more than one method with the same name in the same class.
- The overloaded methods must have different method signatures, which means that the number or types of parameters should differ between the overloaded methods.
- Method overloading is often used to create variations of a method to handle different data types or a different number of parameters, making it more convenient for the caller.
- Method overloading occurs at compile-time and is resolved based on the method’s parameter list. Example of method overloading:
public class Calculator {
public int add(int a, int b) {
return a + b;
}
public double add(double a, double b) {
return a + b;
}
}
- Method Overriding:
- Method overriding is a feature that allows a subclass to provide a specific implementation of a method that is already defined in its superclass.
- The overriding method in the subclass must have the same name, return type, and parameter list as the method in the superclass. It can have a more specific (sub-type) access modifier.
- Method overriding is used to achieve polymorphism, where the appropriate method is called at runtime based on the actual object type.
- Overriding occurs when a subclass provides a specific implementation for a method defined in the superclass. Example of method overriding:
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Dog barks");
}
}
In the example above, the Dog
class overrides the makeSound
method defined in the Animal
class to provide its own specific implementation.
The method overloading is about defining multiple methods with the same name in a class, but with different parameter lists, allowing you to provide convenience and flexibility in method calls. Method overriding is about providing a specific implementation of a method in a subclass to achieve polymorphism and customize the behavior of methods inherited from the superclass. Overloading and overriding serve different purposes and occur at different times in the program’s lifecycle: overloading at compile-time and overriding at runtime.