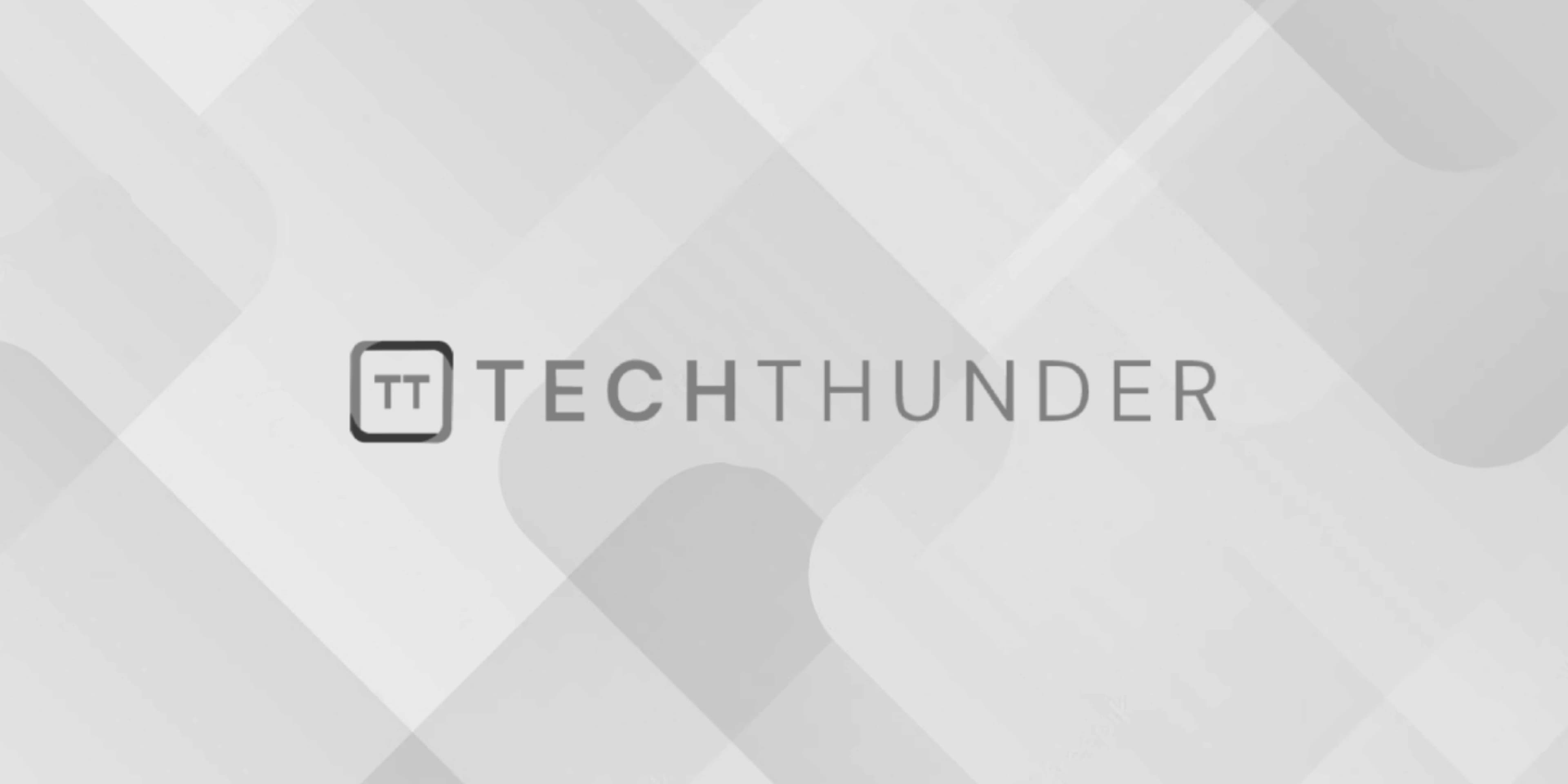
276 views
Java Abstract vs Interface
The Java has both abstract classes and interfaces are used to achieve abstraction and define contracts for classes, but they serve different purposes and have distinct characteristics. Here’s a comparison between abstract classes and interfaces in Java:
Abstract Classes:
- Method Definitions: Abstract classes can have abstract (unimplemented) methods as well as concrete (implemented) methods. Abstract methods are declared using the
abstract
keyword. - Fields: Abstract classes can have fields, including instance variables and constants.
- Constructors: Abstract classes can have constructors, and they are called when an instance of a concrete subclass is created.
- Inheritance: A class can extend only one abstract class. Abstract classes support single inheritance in Java.
- Method Overriding: Abstract methods in abstract classes must be overridden in concrete subclasses.
- Code Reusability: Abstract classes are suitable for sharing code and providing a common base for related classes.
- Default Method: Starting from Java 8, abstract classes can have default methods, which provide a default implementation that can be optionally overridden by subclasses.
Interfaces:
- Method Definitions: Interfaces can only have abstract method signatures. They do not contain method implementations. All methods in an interface are implicitly
public
andabstract
. - Fields: Interfaces can only contain constant (static final) fields, which are implicitly
public
,static
, andfinal
. - Constructors: Interfaces cannot have constructors because they cannot be instantiated directly.
- Inheritance: A class can implement multiple interfaces, allowing it to inherit abstract method signatures and constants from multiple sources. This supports multiple inheritance of types in Java.
- Method Overriding: Classes that implement interfaces must provide concrete implementations for all methods defined in the interface.
- Code Reusability: Interfaces are suitable for defining a contract that multiple, unrelated classes can adhere to.
- Default Method: Starting from Java 8, interfaces can have default methods, which provide a default implementation that can be optionally overridden by implementing classes.
When to Use Abstract Classes:
- Use abstract classes when you have a common base class that provides a shared implementation and you want to enforce some common functionality among related classes.
- Use abstract classes when you need to provide fields, constructors, or default method implementations.
- Use abstract classes when you have a single inheritance relationship, and multiple inheritance is not required.
When to Use Interfaces:
- Use interfaces when you want to define a contract for multiple unrelated classes to adhere to. Interfaces enable polymorphism and allow different classes to implement the same interface.
- Use interfaces when you need multiple inheritance of types, as a class can implement multiple interfaces.
- Use interfaces when you want to provide a common set of method signatures that classes can implement differently.
The common to use a combination of abstract classes and interfaces to design a flexible and extensible class hierarchy in Java. The choice between abstract classes and interfaces depends on the specific design goals of your application.