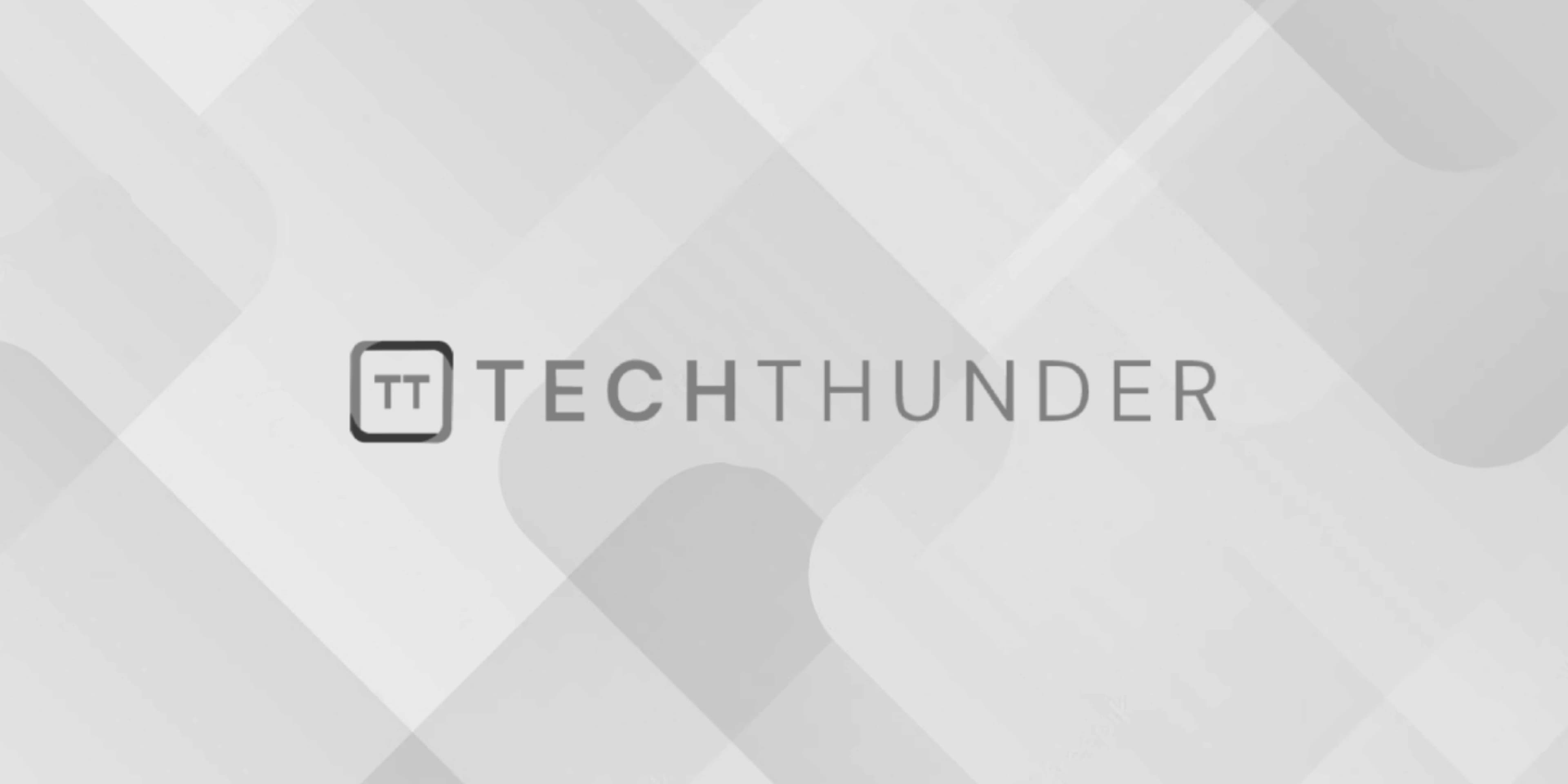
Java Do While Loop
The do-while
loop in Java is a control structure that repeatedly executes a block of code as long as a specified condition is true. It is similar to the while
loop, but the key difference is that the do-while
loop guarantees that the code block is executed at least once, even if the condition is initially false. Here’s the basic syntax of the do-while
loop in Java:
do {
// Code to repeat
} while (condition);
- The code block inside the
do
block is executed first, and then thecondition
is evaluated. - If the
condition
istrue
, the loop continues and the code block is executed again. - If the
condition
isfalse
, the loop terminates.
Here’s an example of using a do-while
loop to print numbers from 1 to 5:
public class Main {
public static void main(String[] args) {
int i = 1;
do {
System.out.println(i);
i++; // Increment the loop control variable
} while (i <= 5);
}
}
In this example:
int i = 1
initializes the loop control variablei
to 1 before entering the loop.- The code block inside the
do
block is executed first, printing the value ofi
. i++
increments the loop control variablei
by 1 after each iteration.i <= 5
is the condition, specifying that the loop will continue as long asi
is less than or equal to 5.
The loop will execute five times, printing the numbers 1 to 5, just like in the while
loop example. The key difference is that with a do-while
loop, you are guaranteed to execute the code block at least once before checking the condition.
The do-while
loop is useful when you want to ensure that a particular action is performed at least once, regardless of the initial condition.