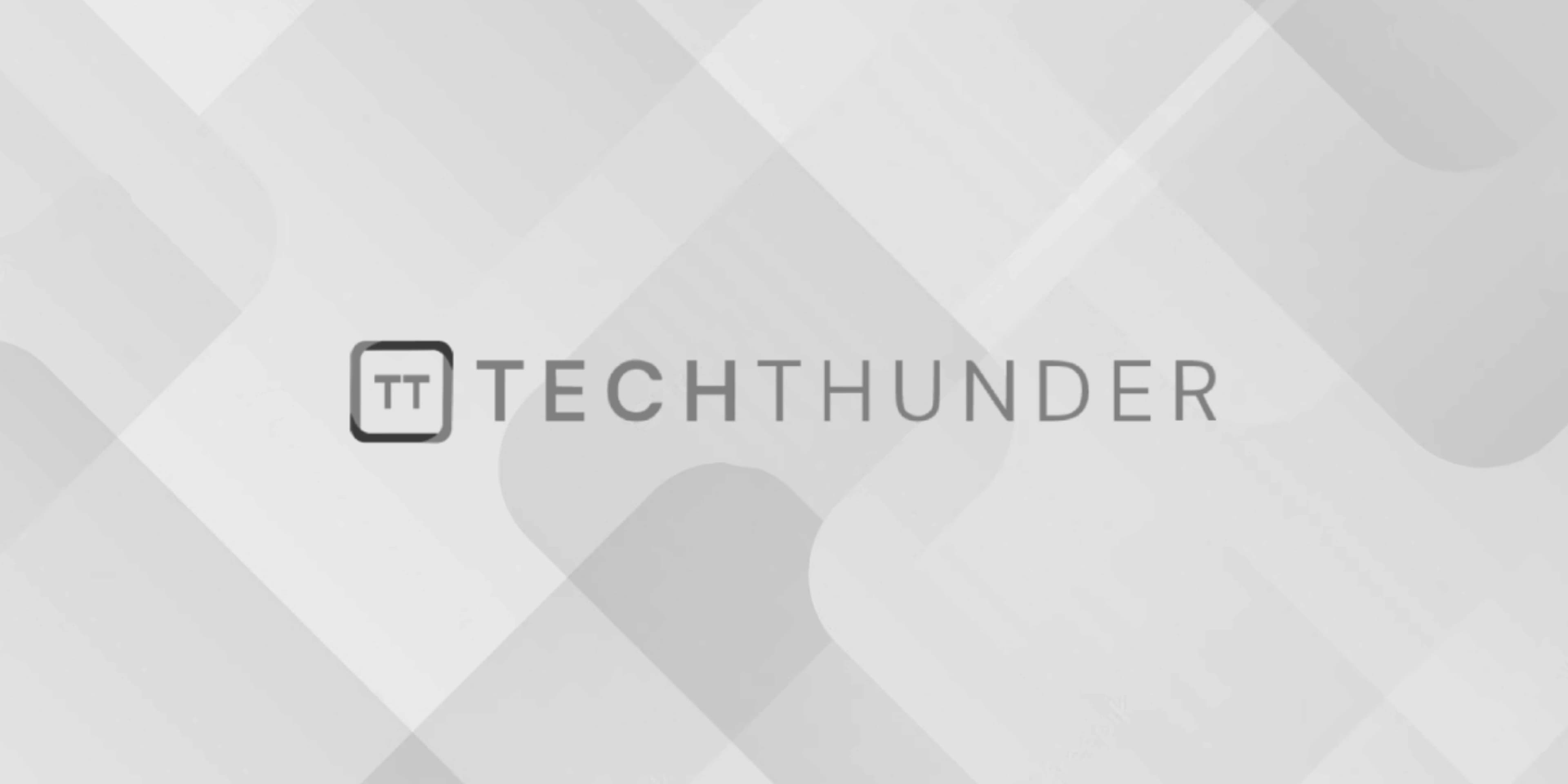
Java Recursion
Recursion is a programming technique in which a method or function calls itself to solve a problem in smaller, more manageable parts. In Java, like in many programming languages, you can implement recursive algorithms to solve problems that can be broken down into simpler, similar subproblems. Recursion is commonly used for tasks such as traversing tree structures, searching through arrays, and solving mathematical problems.
Here’s a basic outline of how recursion works in Java:
- Base Case: Every recursive algorithm must have a base case or termination condition. The base case defines the simplest scenario where the function does not call itself but returns a result directly. It’s essential to have a base case to prevent infinite recursion.
- Recursive Case: In the recursive case, the function calls itself with a modified version of the problem. This means that the problem is reduced in some way, typically by reducing the size of the input or moving closer to the base case.
- Recursive Calls: The function makes one or more recursive calls to itself, solving the same problem on a smaller scale. Each time the function is called, it brings the problem closer to the base case.
- Combining Results: The results of the recursive calls are combined to solve the original problem. This step is often necessary when the problem requires aggregating results from the smaller subproblems.
Here’s a simple example of a recursive function in Java to calculate the factorial of a number:
public class Factorial {
public static int factorial(int n) {
// Base case: factorial of 0 is 1
if (n == 0) {
return 1;
}
// Recursive case: factorial(n) = n * factorial(n-1)
return n * factorial(n - 1);
}
public static void main(String[] args) {
int number = 5;
int result = factorial(number);
System.out.println("Factorial of " + number + " is " + result);
}
}
In this example, the factorial()
function calculates the factorial of a number using recursion. The base case is when n
is 0, and the recursive case calculates the factorial as n * factorial(n - 1)
.
Recursion can be a powerful tool for solving complex problems by breaking them down into simpler subproblems. However, it’s important to use it with care, ensuring that the base case is well-defined, and that recursive calls eventually reach the base case. Otherwise, you may encounter stack overflow errors due to excessive function calls.