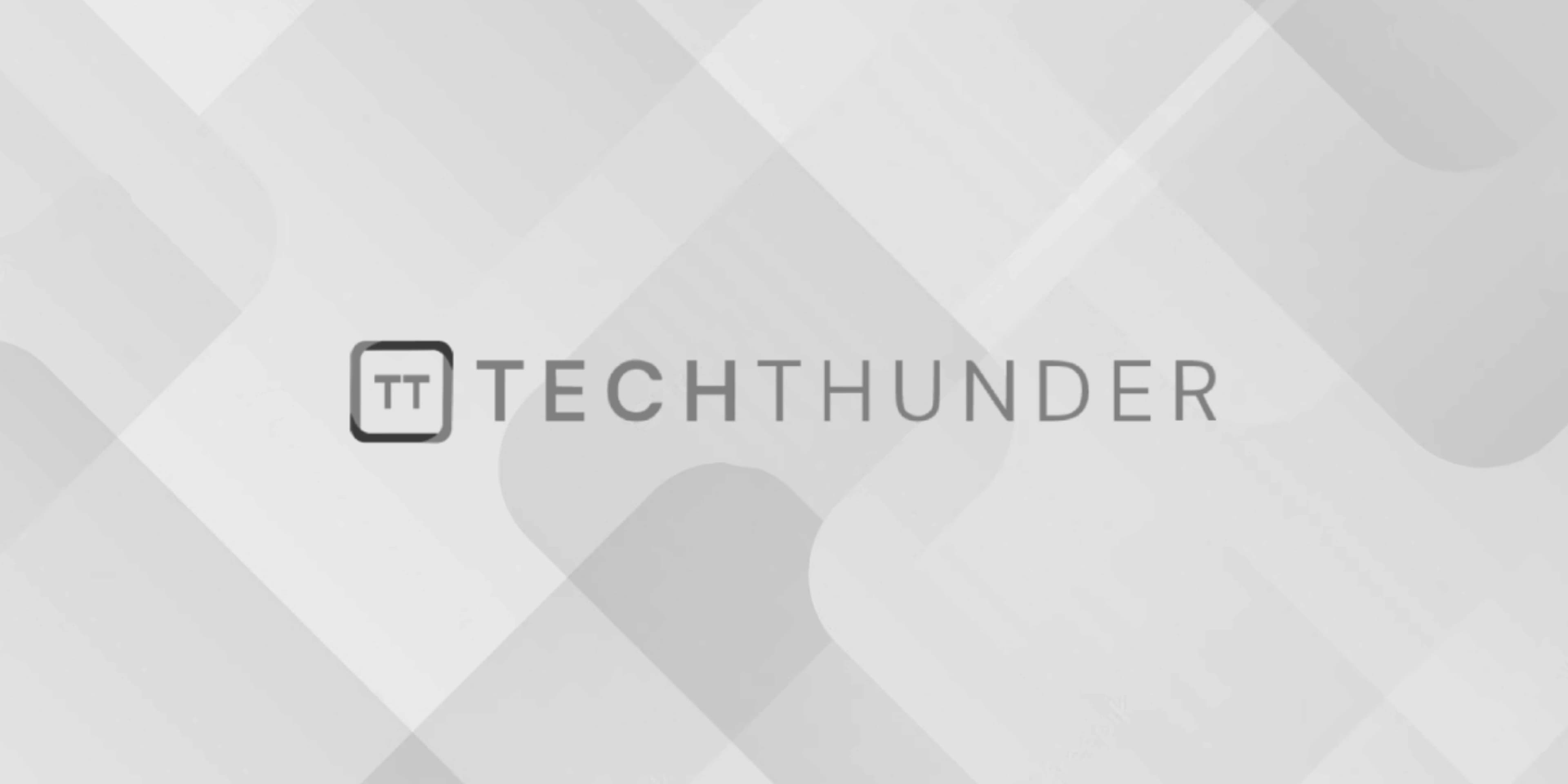
174 views
Java super keyword
The Java super
keyword is used to refer to the superclass or parent class. It has several important uses, especially in the context of inheritance. The super
keyword allows you to access and call the members (fields, methods, and constructors) of the superclass from within a subclass. Here are the main uses of the super
keyword:
- Accessing Superclass Members:
- You can use
super
to access fields and methods of the superclass. This is particularly useful when a subclass has overridden a method or when a subclass wants to reference a hidden superclass member.
class Superclass {
int x = 10;
void display() {
System.out.println("Superclass: " + x);
}
}
class Subclass extends Superclass {
int x = 20;
void display() {
System.out.println("Subclass: " + x);
System.out.println("Superclass: " + super.x);
super.display();
}
}
- Invoking Superclass Constructors:
- When you create an instance of a subclass, the constructor of the superclass is implicitly called. You can use
super
to explicitly call a specific constructor of the superclass. This is useful for initializing the superclass’s state.
class Superclass {
int x;
Superclass(int x) {
this.x = x;
}
}
class Subclass extends Superclass {
int y;
Subclass(int x, int y) {
super(x); // Call the superclass constructor to initialize the 'x' field.
this.y = y;
}
}
- Superclass Method Overriding:
- When a subclass overrides a method from the superclass, it can use
super
to invoke the overridden method from the superclass. This is useful when the subclass wants to extend or modify the behavior of the superclass method.
class Superclass {
void printMessage() {
System.out.println("Superclass message");
}
}
class Subclass extends Superclass {
@Override
void printMessage() {
super.printMessage(); // Calls the overridden method in the superclass.
System.out.println("Subclass message");
}
}
The super
keyword is essential in situations where a subclass needs to work with the members of the superclass while still extending or customizing the behavior. It helps ensure that the subclass and superclass cooperate effectively in the context of inheritance.