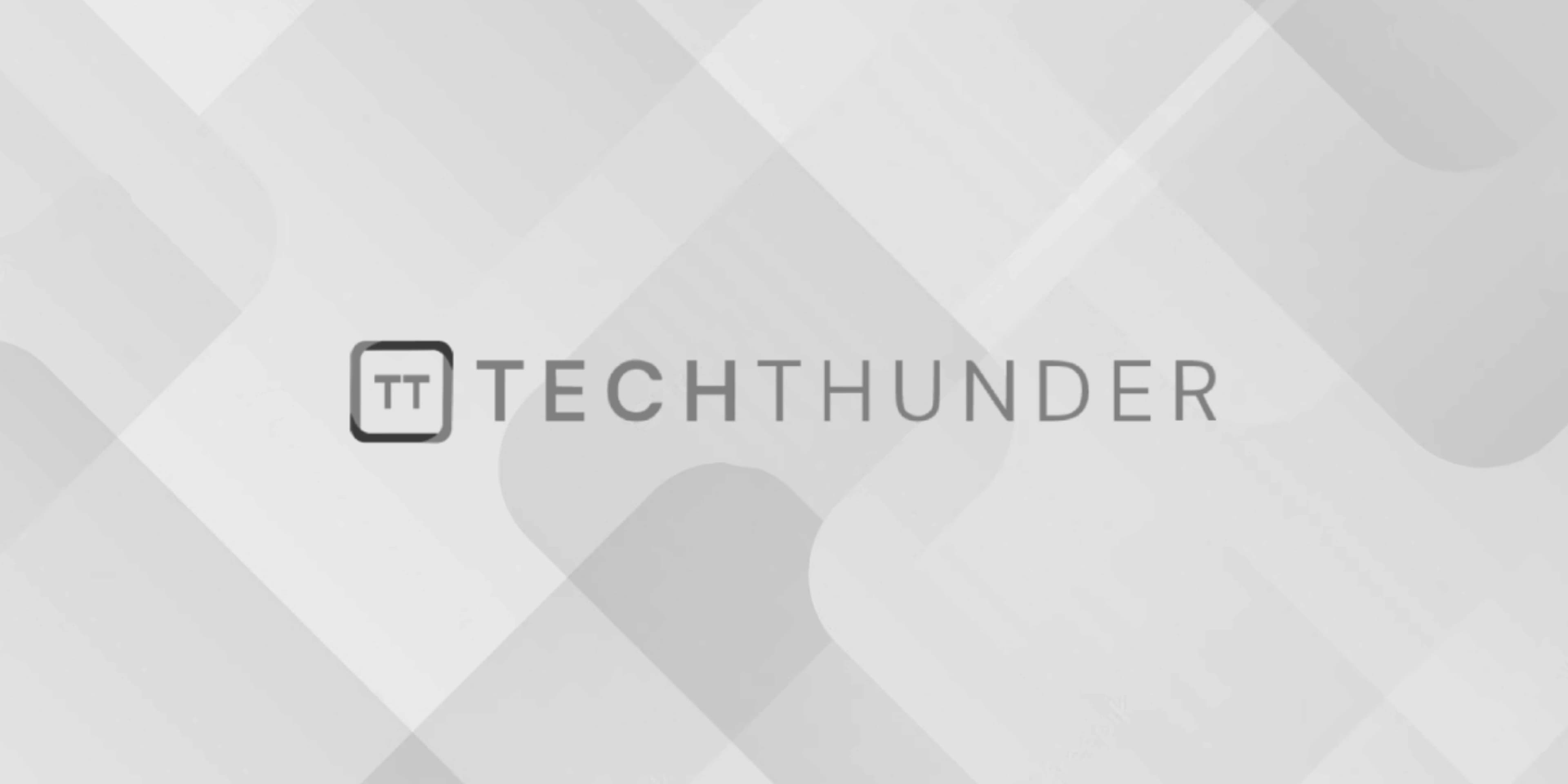
Java strictfp keyword
The strictfp
keyword is used in Java to ensure that floating-point calculations are performed consistently across different platforms and compilers. It stands for “strict floating-point” and is a modifier that can be applied to classes, interfaces, and methods. When a class, interface, or method is marked as strictfp
, it guarantees strict adherence to the IEEE 754 standard for floating-point arithmetic.
Here are the key points to understand about the strictfp
keyword:
- IEEE 754 Standard: The IEEE 754 standard defines the format and rules for representing and performing arithmetic with floating-point numbers. It helps ensure consistency in how floating-point calculations are executed, especially when dealing with different hardware platforms and compilers.
- Cross-Platform Consistency: When you mark a class, interface, or method as
strictfp
, you are telling the Java compiler to generate code that strictly adheres to the IEEE 754 standard. This ensures that floating-point calculations produce the same results, regardless of the underlying platform. - Use Cases: While strict adherence to the IEEE 754 standard is important in some applications, it may not be necessary for all programs. You typically use the
strictfp
keyword when you want to guarantee consistent results in floating-point calculations. This is often relevant in scientific or financial applications where precision and consistency are critical. - Applying
strictfp
: You can apply thestrictfp
keyword to the following:
- Entire classes and interfaces: When applied to a class or interface, it ensures that all methods in that class/interface are
strictfp
. - Individual methods: You can apply
strictfp
to specific methods within a class, making only those methods adhere to the IEEE 754 standard.
Here’s an example of applying strictfp
to a method:
public class MathOperations {
public strictfp double calculateResult(double a, double b) {
return a / b;
}
}
In this example, the calculateResult
method is marked as strictfp
, ensuring that the floating-point division operation strictly adheres to the IEEE 754 standard, producing consistent results.
It’s important to note that, in practice, you may not need to use strictfp
in most applications. Modern Java platforms and compilers typically provide consistent floating-point behavior by default. However, when you require strict consistency for certain applications, the strictfp
keyword is available as a tool to achieve that goal.