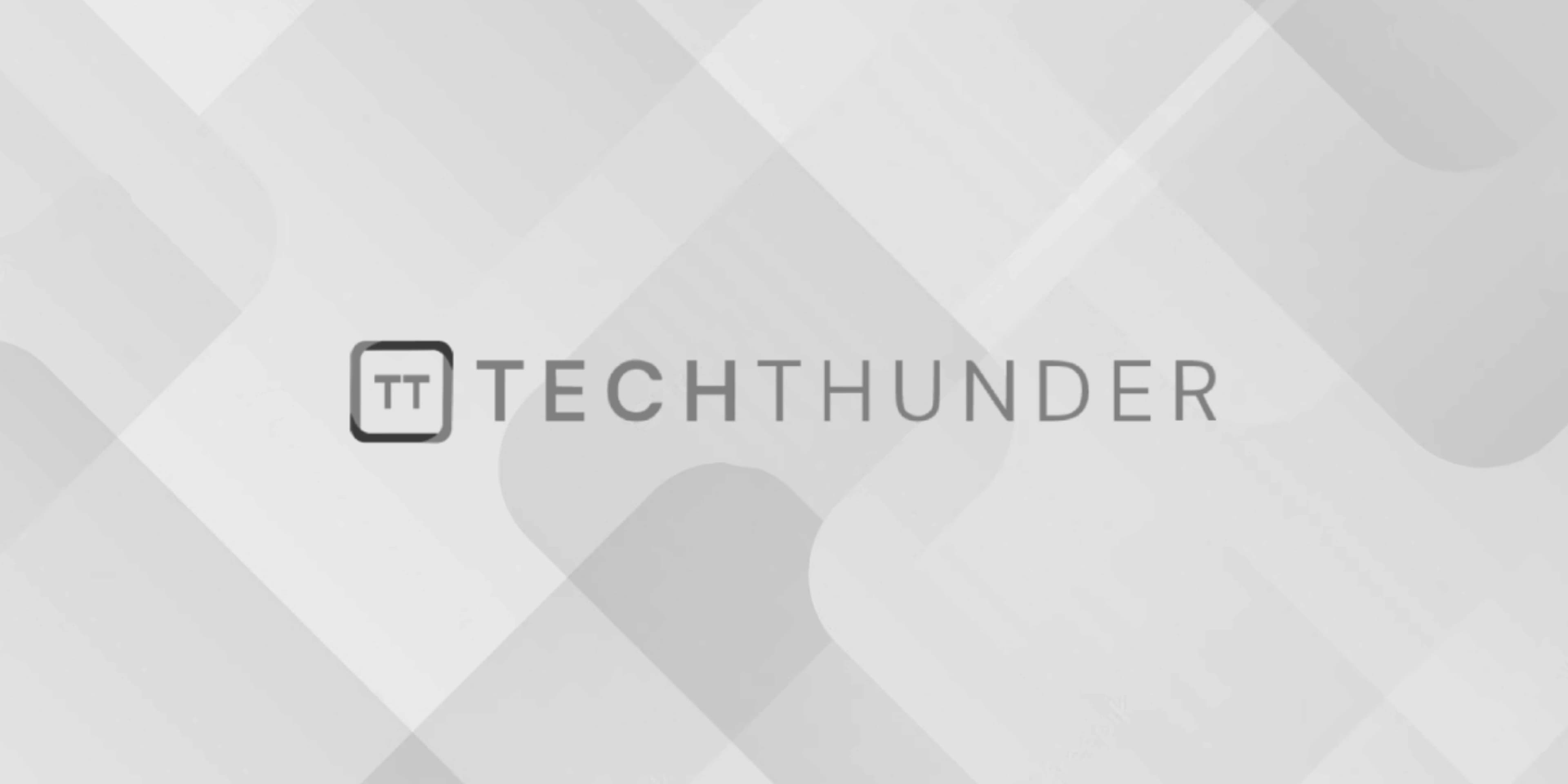
Java Covariant Return Type
Covariant return types, introduced in Java 5, allow a subclass method to return a more specific (subclass) type than the return type of the overridden method in the superclass. This feature makes it easier to work with inheritance and polymorphism, as it allows you to make the return types of overridden methods more precise without breaking compatibility with the superclass. Here’s how covariant return types work in Java:
- Method Signature Consistency:
- To use covariant return types, the overridden method in the subclass must have the same method signature (method name, parameter types, and order) as the overridden method in the superclass. The only difference is in the return type.
- Return Type Compatibility:
- The return type of the overriding method in the subclass must be a subclass of the return type of the overridden method in the superclass. This means you can return a more specific type in the subclass, but you cannot return a broader type.
@Override
Annotation:
- Using the
@Override
annotation is a good practice when working with covariant return types to help catch errors at compile time.
Here’s an example of covariant return types in Java:
class Animal {
Animal giveBirth() {
System.out.println("An animal gives birth.");
return new Animal();
}
}
class Dog extends Animal {
@Override
Dog giveBirth() {
System.out.println("A dog gives birth to a puppy.");
return new Dog();
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog();
animal.giveBirth();
}
}
In this example, the giveBirth
method is overridden in the Dog
class with a more specific return type, which is Dog
. This is a covariant return type because it returns a subclass of the return type of the overridden method in the Animal
class.
Covariant return types are particularly useful when you have a hierarchy of classes and you want to refine the return type in subclasses. This feature allows you to write more expressive and self-documenting code while still maintaining compatibility with the superclass.