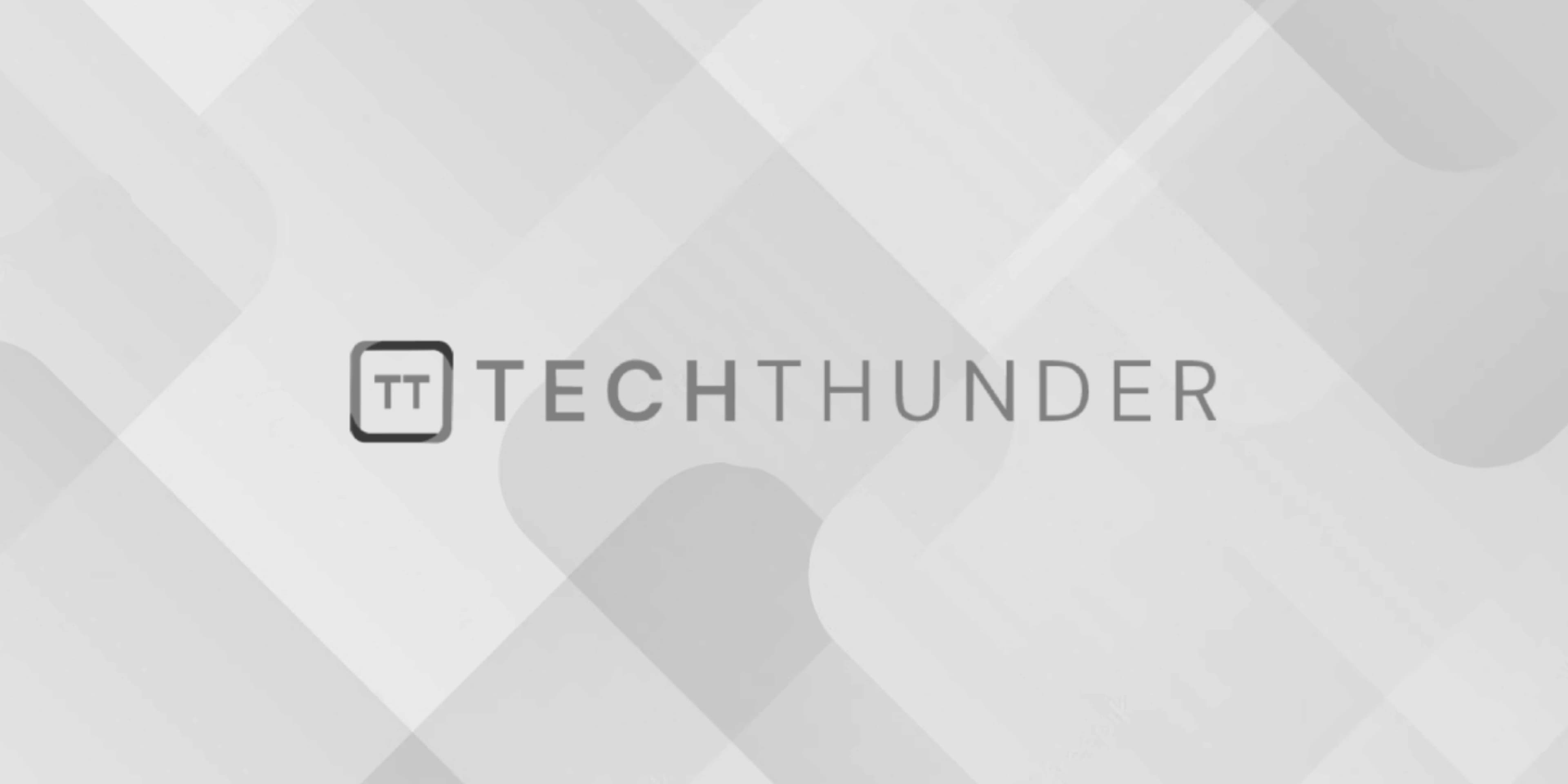
174 views
Java String
The Java String
class is a fundamental class for working with text. It is part of the Java Standard Library and provides methods and features for creating, manipulating, and managing strings. Here are some key points about the String
class:
- Immutable: Strings in Java are immutable, which means that their content cannot be changed after they are created. When you perform operations on a
String
, such as concatenation or substring extraction, it creates a newString
object rather than modifying the original one. This immutability ensures data integrity. - String Literals: You can create strings in Java by using string literals enclosed in double quotes. For example:
"Hello, World!"
. - Concatenation: Strings can be concatenated using the
+
operator. This operator is overloaded for string concatenation. For example:
String greeting = "Hello";
String name = "Alice";
String message = greeting + ", " + name;
- String Pool: Java maintains a string pool (also known as the string constant pool) for storing string literals. Strings created with the same literal value are stored in the pool, which can help reduce memory usage.
- String Methods: The
String
class provides numerous methods for manipulating strings, such as:
length()
: Returns the length (number of characters) of the string.charAt(int index)
: Returns the character at the specified index.substring(int beginIndex)
: Returns a substring starting from thebeginIndex
to the end of the string.equals(Object obj)
: Compares the string to another object for equality.equalsIgnoreCase(String anotherString)
: Compares the string to another string while ignoring case.split(String regex)
: Splits the string into an array of substrings using a regular expression as the delimiter.- And many more for searching, replacing, and formatting strings.
- String Comparison: You can compare strings for equality using the
equals()
method. To perform case-insensitive comparisons, you can useequalsIgnoreCase()
. For lexicographical ordering, you can usecompareTo()
. - String Interning: Java provides the
intern()
method that allows a string to be added to the string pool if it is not already present. This can be useful for optimizing memory usage in specific cases. - StringBuffer and StringBuilder: If you need to perform mutable string operations, you can use the
StringBuffer
andStringBuilder
classes. These classes provide methods for modifying strings in a mutable way. - String Handling Best Practices: When dealing with strings, be aware of the immutability and the potential memory overhead of creating new strings. Choose the appropriate class for the task—
String
,StringBuffer
, or `StringBuilder—based on whether you need immutability, thread safety, or performance.
The String
class is a fundamental part of Java and is widely used in applications for text processing and manipulation. Understanding its characteristics and methods is essential for effective string handling in Java programs.