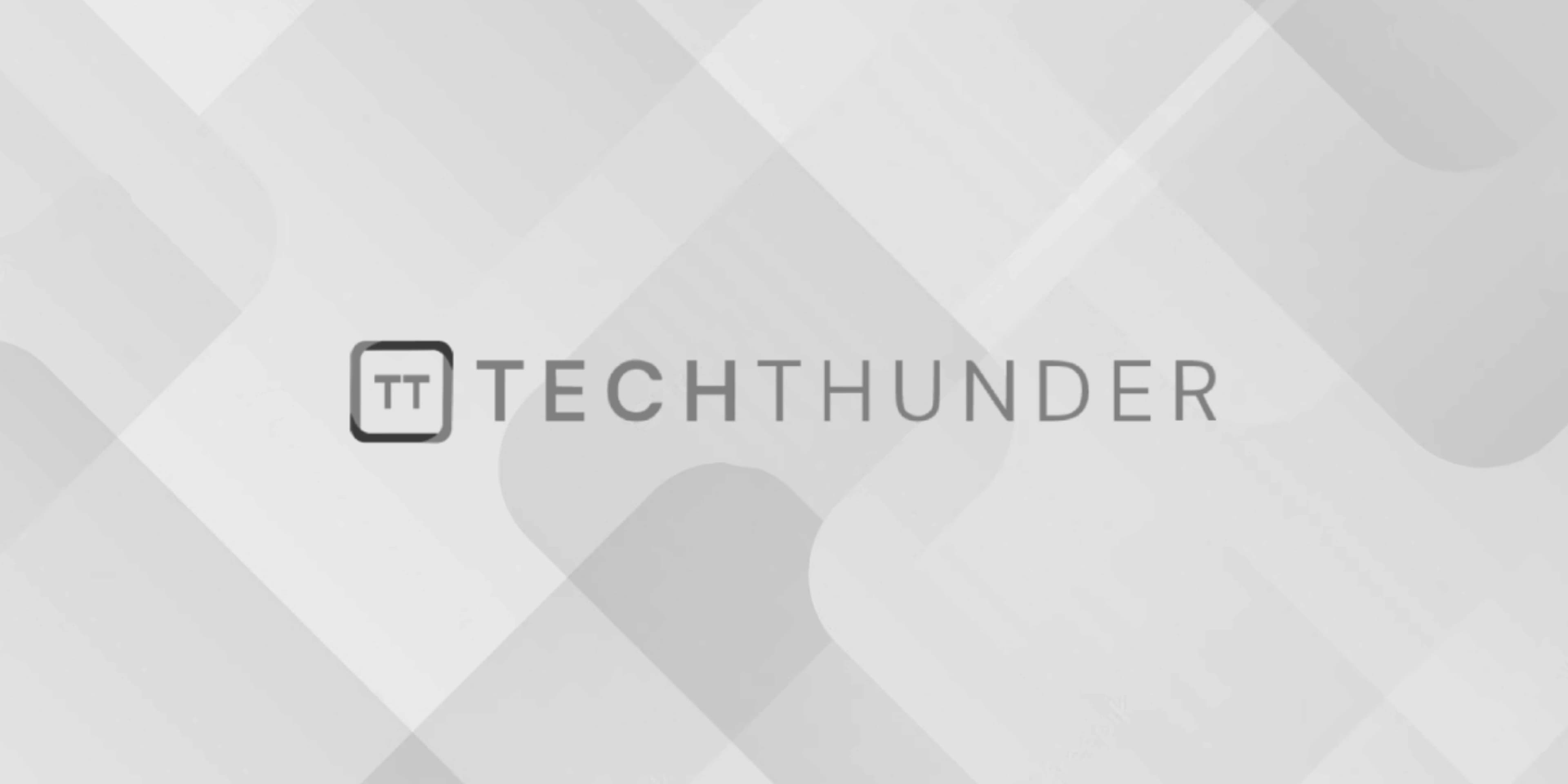
Java While Loop
The while
loop in Java is a control structure that repeatedly executes a block of code as long as a specified condition is true. It is often used when you don’t know in advance how many times the loop will need to execute. Here’s the basic syntax of the while
loop in Java:
while (condition) {
// Code to repeat
}
- The
condition
is evaluated before each iteration of the loop. If the condition istrue
, the loop continues; if it’sfalse
, the loop terminates. - The code inside the
while
loop is executed as long as the condition remainstrue
.
Here’s an example of using a while
loop to print numbers from 1 to 5:
public class Main {
public static void main(String[] args) {
int i = 1;
while (i <= 5) {
System.out.println(i);
i++; // Increment the loop control variable
}
}
}
In this example:
int i = 1
initializes the loop control variablei
to 1 before entering the loop.i <= 5
is the condition, specifying that the loop will continue as long asi
is less than or equal to 5.i++
increments the loop control variablei
by 1 after each iteration.
The loop will execute five times, printing the numbers 1 to 5.
You can use the while
loop for situations where you need to repeat a block of code until a specific condition is no longer met. It’s particularly useful when the number of iterations is not known in advance and is determined by the condition. Just be cautious to ensure that the condition will eventually become false
to avoid infinite loops.