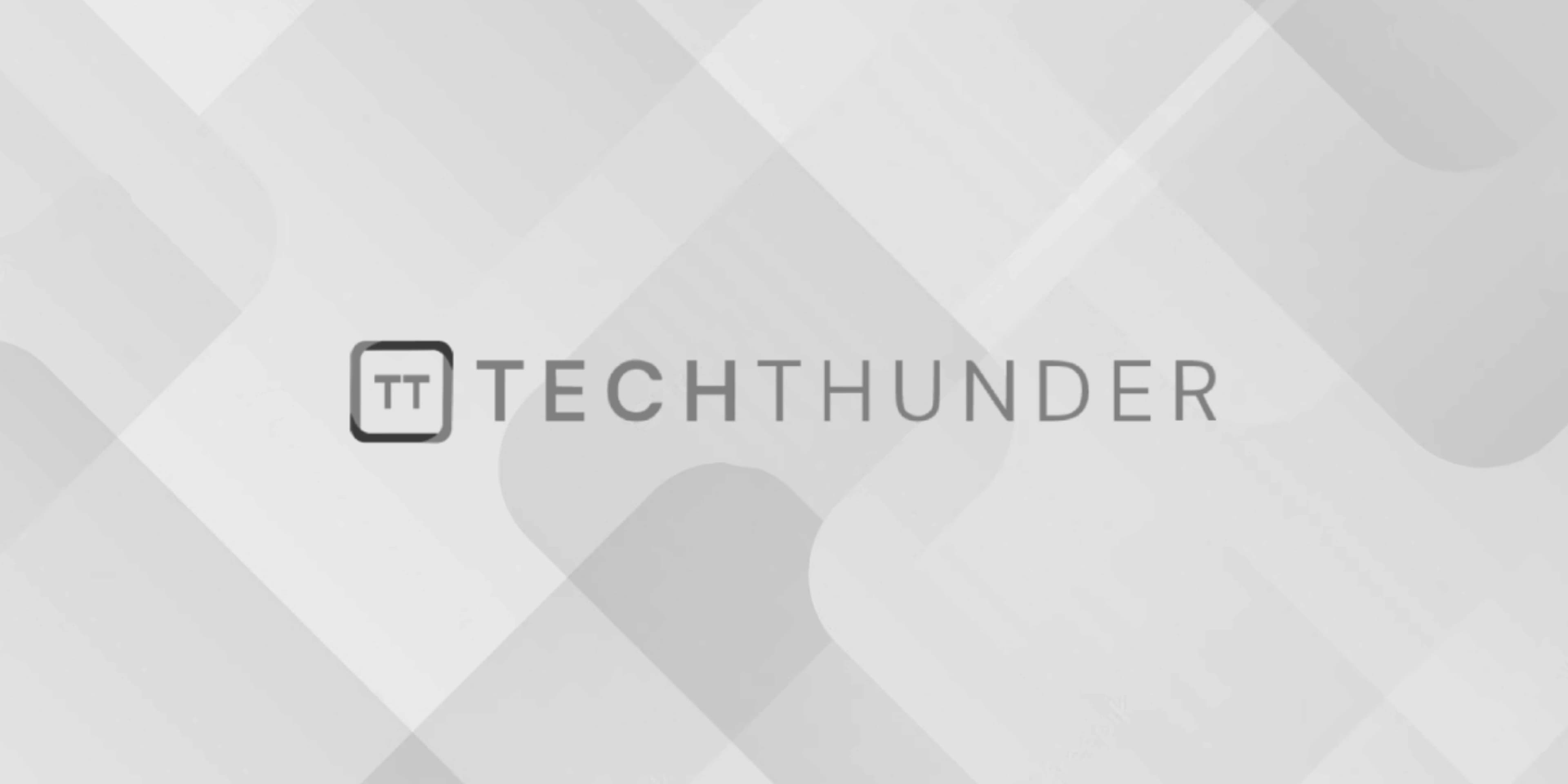
Java Date
The java.util.Date
class represents a specific point in time, including date and time information. However, the Date
class has some limitations and design issues, and as of my last knowledge update in September 2021, it’s considered somewhat outdated. The java.time
package introduced in Java 8 provides more comprehensive and flexible date and time handling through classes like LocalDate
, LocalTime
, LocalDateTime
, and more.
Here’s a brief overview of the java.util.Date
class:
- Creation and Manipulation:
You can create aDate
object to represent the current date and time usingnew Date()
. You can also provide a timestamp in milliseconds to construct aDate
object for a specific time. - Date Formatting:
To display the date in a human-readable format, you can use theSimpleDateFormat
class to format aDate
object into a string. - Arithmetic and Comparison:
TheDate
class allows for basic arithmetic and comparison operations. However, these methods are not as user-friendly as those provided by thejava.time
package. - Immutability and Mutability:
TheDate
class is mutable, which means that its state can be changed after creation. This mutability can lead to unexpected behavior in multi-threaded environments. - Time Zone Handling:
TheDate
class doesn’t handle time zones properly. It uses the system’s default time zone, which can lead to confusion and errors when dealing with different time zones.
Here’s a simple example of using the Date
class:
import java.util.Date;
public class DateExample {
public static void main(String[] args) {
Date currentDate = new Date();
System.out.println("Current date and time: " + currentDate);
long timestamp = System.currentTimeMillis();
Date specificDate = new Date(timestamp);
System.out.println("Specific date and time: " + specificDate);
}
}
For modern date and time handling, it’s recommended to use the java.time
package and its classes, which provide better support for time zones, formatting, arithmetic operations, and more. If you’re working with Java 8 or later, I suggest exploring the java.time
package for more advanced and reliable date and time handling.