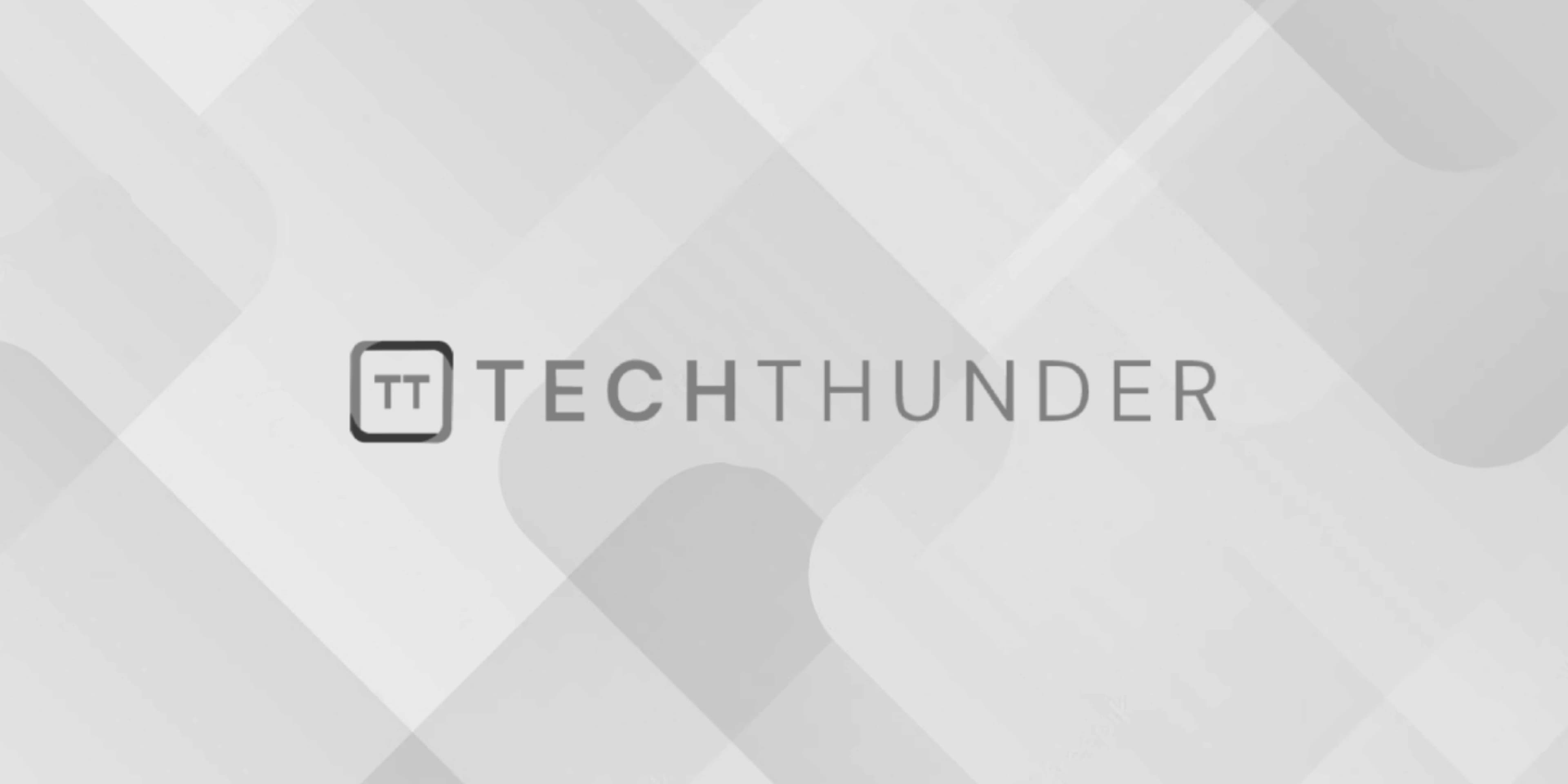
269 views
Java Array
The Java array is a data structure used to store a fixed-size, ordered collection of elements of the same data type. Arrays are fundamental to programming and are commonly used to store and manipulate data. Here are some key points about Java arrays:
- Declaration and Initialization:
- To declare an array, you specify the data type of its elements, followed by square brackets
[]
. For example,int[] numbers;
declares an array of integers. - To initialize an array, you allocate memory and specify its size. For example,
numbers = new int[5];
creates an array of integers with space for five elements.
- Array Indexing:
- Elements in an array are accessed by their index, starting from 0 for the first element and incrementing by 1 for each subsequent element.
- Example:
int firstNumber = numbers[0];
- Array Length:
- You can get the length of an array using the
length
property, which returns the number of elements in the array. - Example:
int arrayLength = numbers.length;
- Array Initialization with Values:
- You can initialize an array with values at the time of declaration using an array initializer. For example:
java int[] numbers = {1, 2, 3, 4, 5};
- Multidimensional Arrays:
- Java supports multidimensional arrays, such as two-dimensional arrays. They are essentially arrays of arrays.
- Example:
java int[][] matrix = { {1, 2, 3}, {4, 5, 6}, {7, 8, 9} };
- Array Elements:
- Array elements can be of any data type, including primitive types and reference types. You can have arrays of integers, strings, objects, and more.
- Dynamic Array:
- Arrays in Java have a fixed size determined at the time of creation. Once an array is created, its size cannot be changed.
- If you need a dynamic array that can grow or shrink, consider using Java’s
ArrayList
class from thejava.util
package.
- Common Array Operations:
- Iterating through array elements using loops (e.g.,
for
orforeach
). - Sorting and searching arrays using various algorithms.
- Modifying and updating elements using array indexing.
- Combining and splitting arrays.
- Working with arrays of objects, such as strings or custom classes.
Here’s an example of creating, initializing, and accessing elements in a simple array:
public class ArrayExample {
public static void main(String[] args) {
// Declare and initialize an array of integers
int[] numbers = {1, 2, 3, 4, 5};
// Access and print array elements
for (int i = 0; i < numbers.length; i++) {
System.out.println("Element at index " + i + ": " + numbers[i]);
}
}
}
Arrays are a fundamental building block in Java and are used in a wide range of applications, from simple data storage to more complex data structures and algorithms.