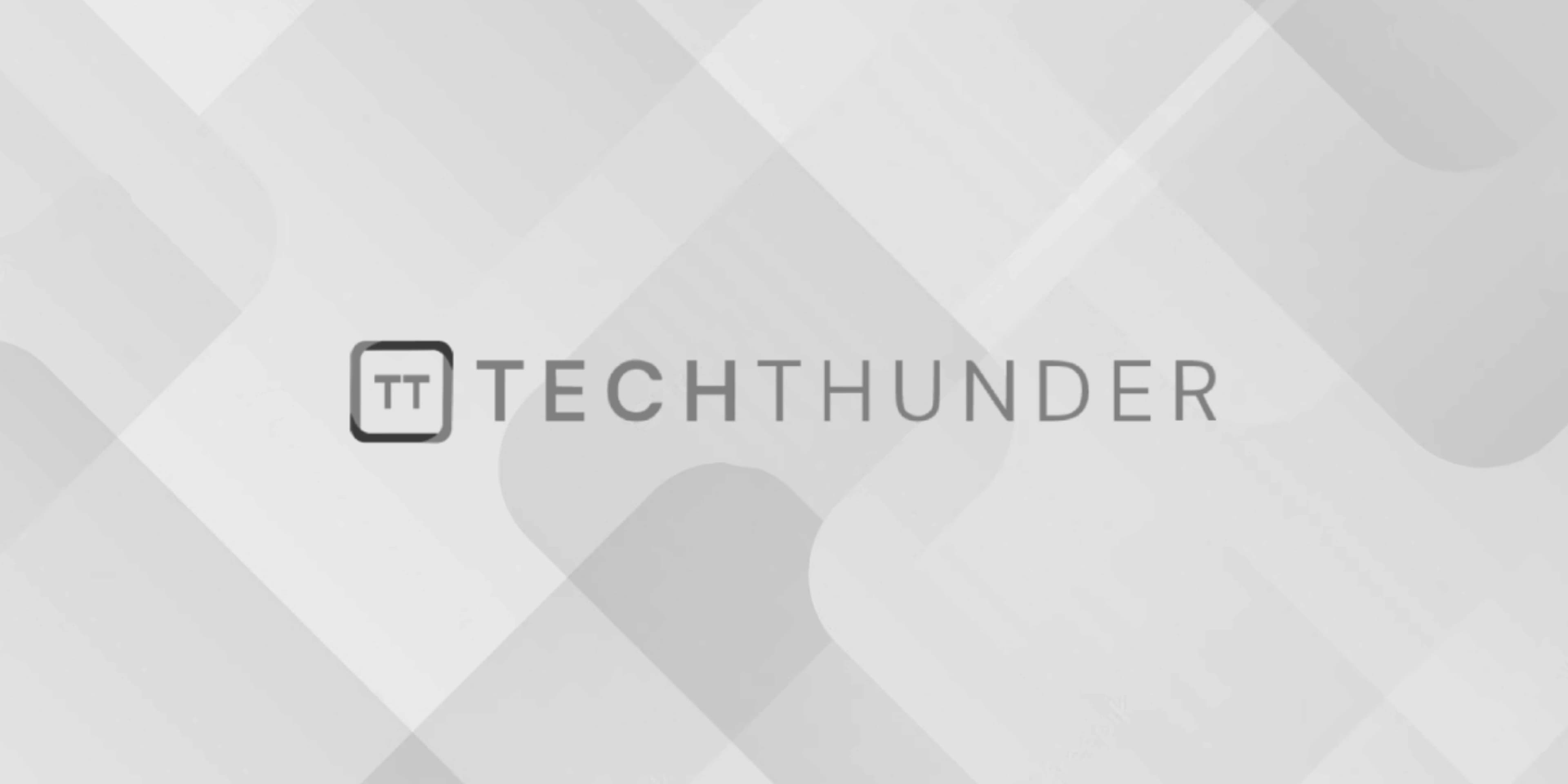
Java Dynamic Binding
Dynamic binding also known as late binding or runtime binding, is a fundamental concept in object-oriented programming and is particularly important in Java. It refers to the process by which the actual implementation of a method (the specific method code to be executed) is determined at runtime, rather than at compile time. Dynamic binding allows for polymorphism, a key feature of object-oriented programming, where the appropriate method to execute is based on the actual object’s type.
Here’s how dynamic binding works in Java:
- Inheritance and Polymorphism:
- Dynamic binding is closely tied to inheritance and polymorphism, two core principles of object-oriented programming.
- In Java, you can create a subclass that extends a superclass. The subclass inherits the methods of the superclass.
- Overriding:
- Dynamic binding comes into play when you override a method in a subclass. Method overriding means that the subclass provides its own implementation for a method that it inherits from the superclass. The overriding method must have the same method signature (name, return type, and parameter list) as the method in the superclass.
- Polymorphic Reference:
- Dynamic binding works when a reference variable of the superclass type is used to refer to an object of the subclass type. This is known as a polymorphic reference. Example:
class Animal {
void makeSound() {
System.out.println("Animal makes a sound");
}
}
class Dog extends Animal {
void makeSound() {
System.out.println("Dog barks");
}
}
public class DynamicBindingExample {
public static void main(String[] args) {
Animal myPet = new Dog(); // Polymorphic reference
myPet.makeSound(); // Calls the overridden method in Dog
}
}
In the example above, myPet
is a reference of type Animal
that actually points to an instance of the Dog
class. At runtime, when myPet.makeSound()
is called, Java determines which implementation of makeSound
to execute. Dynamic binding ensures that the makeSound
method in the Dog
class is called, demonstrating the concept of late binding.
Dynamic binding allows you to write more flexible and maintainable code, where the behavior of a method can be customized in subclasses while providing a common interface through inheritance. It enables polymorphism, which is a powerful way to write code that is more extensible and adaptable to various object types at runtime.