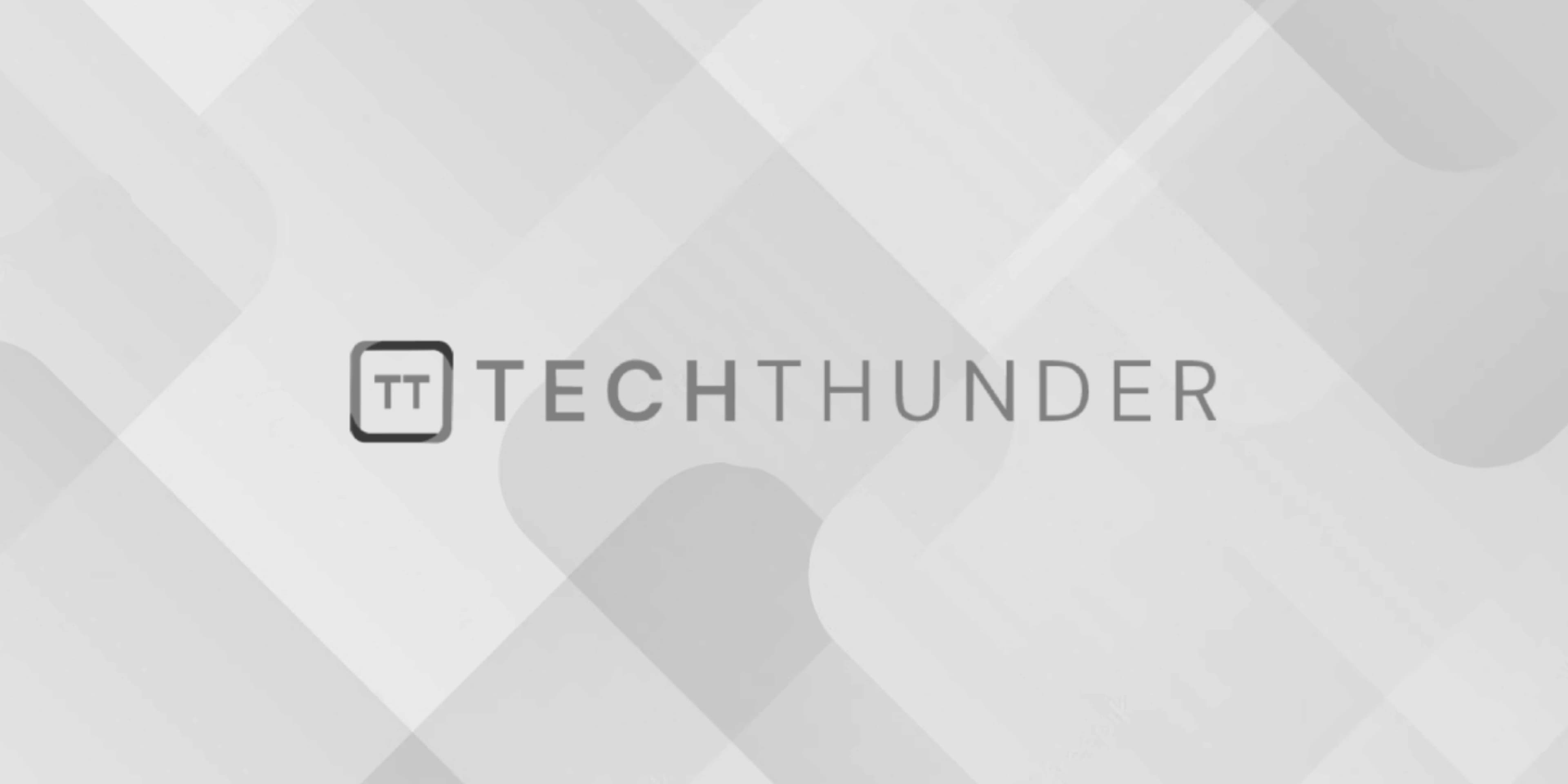
284 views
Java Reflection
Java Reflection is a powerful feature that allows you to examine and manipulate the structure, behavior, and attributes of classes, interfaces, fields, methods, and constructors at runtime. Reflection provides the ability to inspect and interact with Java classes, objects, and their members without knowing their details at compile time. It’s commonly used for debugging, testing frameworks, serialization, and frameworks like Spring and Hibernate.
Key aspects of Java Reflection:
Class
Class: TheClass
class is the starting point for Reflection. It provides methods to obtain information about a class’s name, fields, methods, constructors, superclasses, interfaces, and more.- Accessing Members: Reflection allows you to access fields, methods, and constructors of a class, even if they are private or hidden.
- Dynamic Instantiation: You can create instances of classes at runtime using Reflection’s
newInstance()
method. - Method Invocation: Reflection allows you to invoke methods on objects at runtime using the
Method
class. - Annotations: You can inspect annotations applied to classes, methods, fields, and other program elements using Reflection.
- Dynamic Proxy: Reflection enables the creation of dynamic proxy objects, useful in implementing proxy patterns and aspect-oriented programming.
Here’s a simple example demonstrating Java Reflection:
import java.lang.reflect.*;
public class ReflectionExample {
public static void main(String[] args) throws ClassNotFoundException {
// Get the Class object for a given class name
Class<?> clazz = Class.forName("java.lang.String");
// Get class name
System.out.println("Class Name: " + clazz.getName());
// Get declared methods
Method[] methods = clazz.getDeclaredMethods();
System.out.println("Declared Methods:");
for (Method method : methods) {
System.out.println(" - " + method.getName());
}
// Get declared fields
Field[] fields = clazz.getDeclaredFields();
System.out.println("Declared Fields:");
for (Field field : fields) {
System.out.println(" - " + field.getName());
}
}
}
Keep in mind:
- Reflection comes with some performance overhead due to its dynamic nature.
- It can bypass some access controls and should be used carefully.
- Reflection is more suitable for frameworks and tools than for regular application code.
Java Reflection is a complex topic with many applications. It’s recommended to refer to the official Java documentation and resources for more in-depth understanding and practical usage.