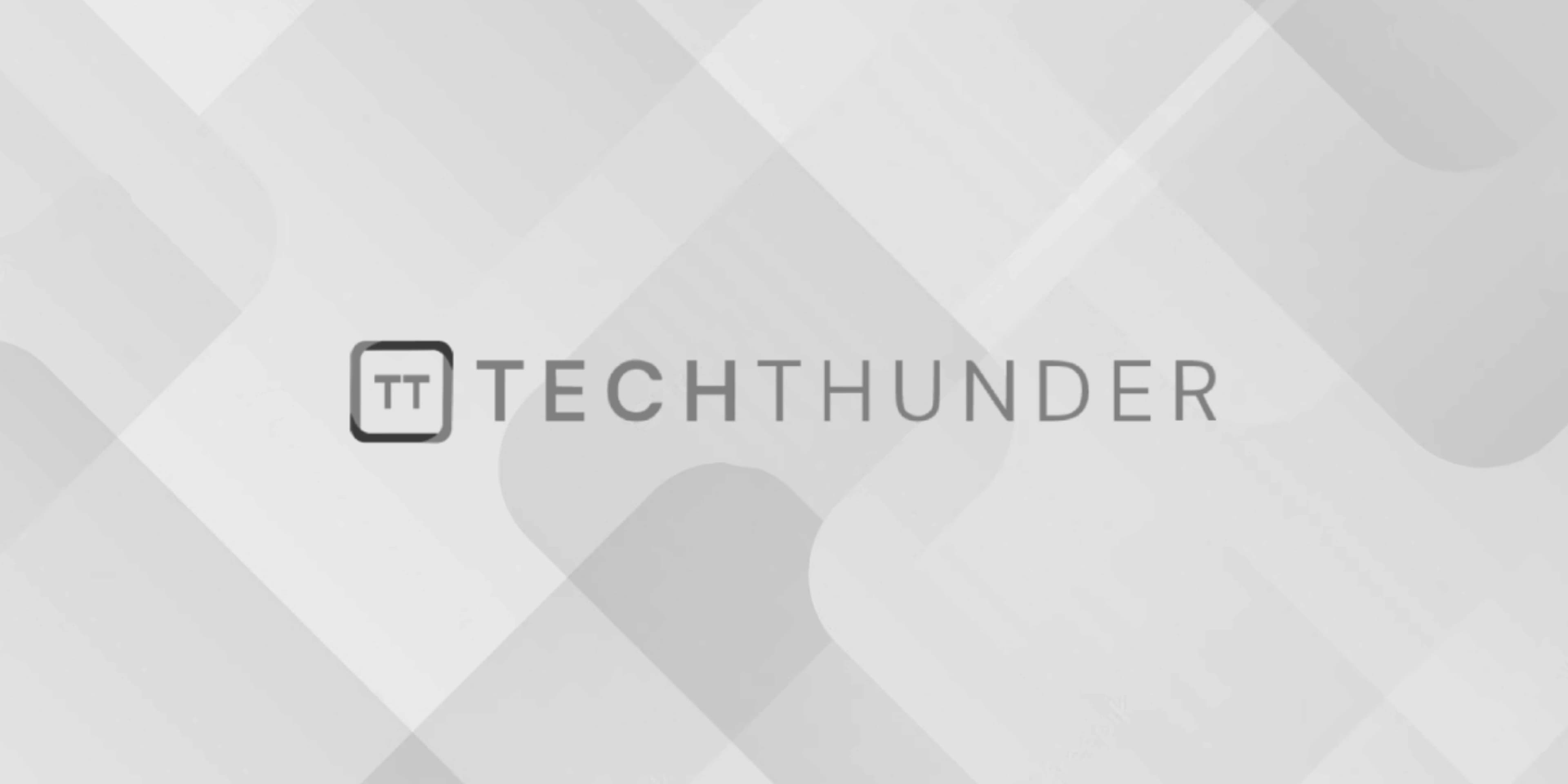
180 views
Java static keyword
The Java static
keyword is used to define a class-level (static) member. When a member, whether it’s a variable or a method, is declared as static
, it belongs to the class itself rather than to instances (objects) of the class. Here are the key aspects of using the static
keyword in Java:
- Static Variables (Class Variables):
- A static variable is shared by all instances of a class. It is created when the class is loaded into memory and destroyed when the class is unloaded.
- Static variables are often used for constants, shared data, or values that are common to all instances of a class.
- To declare a static variable, use the
static
keyword with the variable declaration.
public class MyClass {
static int count = 0; // Static variable shared by all instances
}
- Static Methods:
- A static method is associated with the class rather than with instances of the class. It can be called using the class name, without creating an object.
- Static methods are often used for utility methods, factory methods, or methods that don’t depend on the state of a particular instance.
- To declare a static method, use the
static
keyword with the method declaration.
public class MathUtils {
static int add(int a, int b) {
return a + b;
}
}
- Static Blocks:
- Static blocks are used to initialize static variables or perform one-time setup for a class. They are executed when the class is loaded into memory.
- Static blocks are declared using the
static
keyword followed by a pair of curly braces.
public class MyClass {
static {
// Code for one-time initialization
}
}
- Accessing Static Members:
- To access static members (variables or methods), you use the class name followed by the dot operator. You do not need to create an object of the class to access static members.
int x = MyClass.count; // Accessing a static variable
int result = MathUtils.add(5, 3); // Calling a static method
- Static Import:
- You can use the
static import
feature to import static members from a class directly, allowing you to use them without referencing the class name. This can make code more concise but should be used with caution to maintain clarity.
import static java.lang.Math.*;
public class Main {
public static void main(String[] args) {
double pi = PI; // Using the imported static variable
double sqrt2 = sqrt(2); // Using the imported static method
}
}
- Caution with Static Members:
- Static members should be used with care. They are shared by all instances and can lead to synchronization issues in a multi-threaded environment.
- Constants (e.g.,
public static final
) are a common use case for static variables.
The static
keyword is a fundamental feature in Java and plays a crucial role in creating utility classes, shared data, and defining behavior that is not tied to a specific instance of a class.