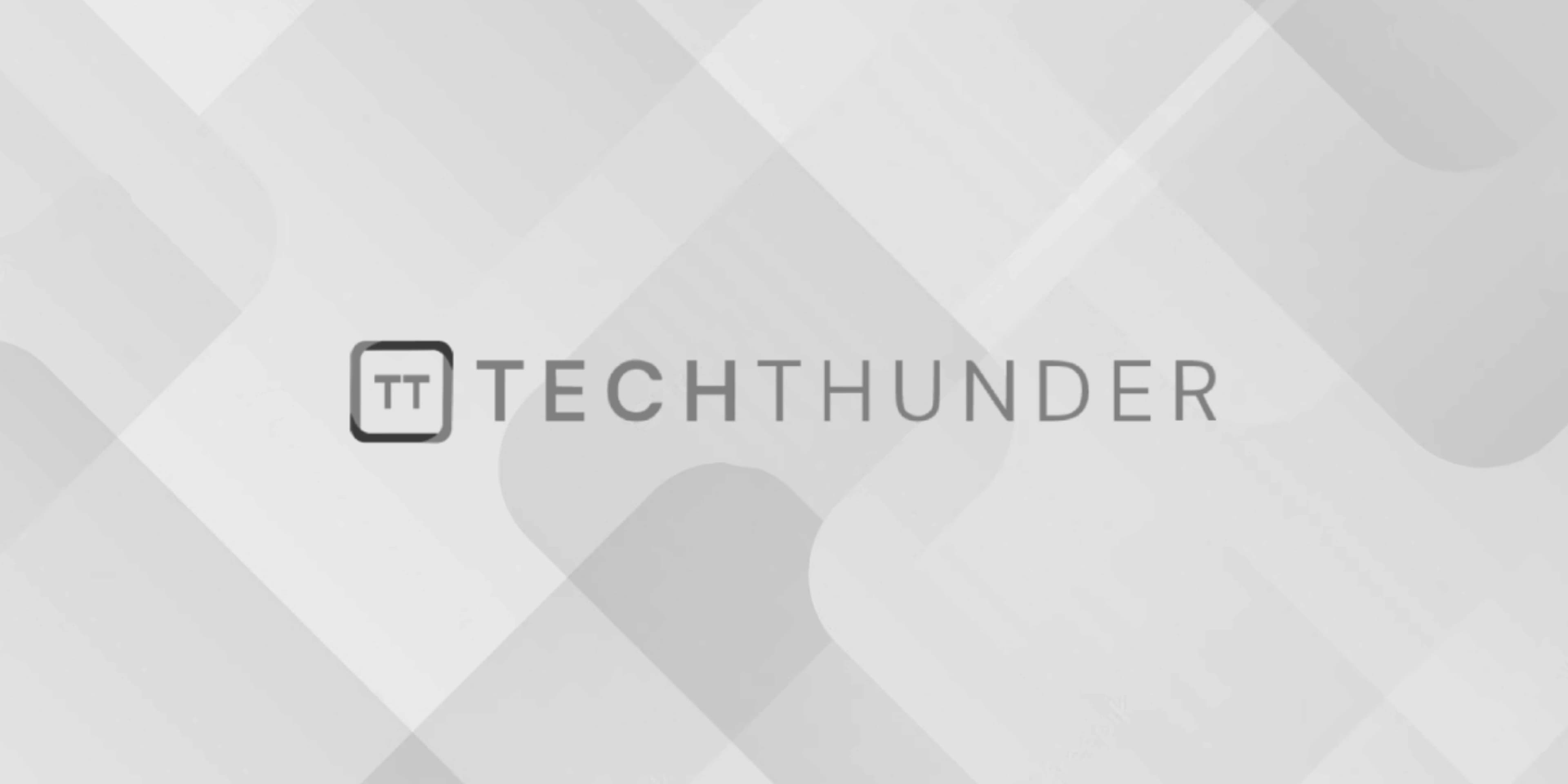
Java Object Cloning
The Java object cloning is the process of creating a duplicate copy of an existing object. Cloning is useful in situations where you need to create a copy of an object with the same data but distinct references. To perform cloning in Java, you can use the clone()
method, provided that the class of the object implements the Cloneable
interface.
Here are the steps to clone an object in Java:
- Ensure that the class of the object to be cloned implements the
Cloneable
interface. TheCloneable
interface is a marker interface, which means it doesn’t define any methods. It’s used to indicate that a class supports cloning. - Override the
clone()
method in the class. Theclone()
method is declared in theObject
class but protected, so you must override it with a public method in your class. - In the
clone()
method, use thesuper.clone()
method to create a shallow copy of the object. This creates a new object with the same field values as the original object. However, the references within the object still point to the same objects (shallow copy). - If necessary, make adjustments to the cloned object to create a deep copy. In the case of reference types, you might need to clone those objects as well.
Here’s an example of cloning an object:
class MyObject implements Cloneable {
private int value;
public MyObject(int value) {
this.value = value;
}
public void setValue(int value) {
this.value = value;
}
public int getValue() {
return value;
}
@Override
public MyObject clone() {
try {
return (MyObject) super.clone();
} catch (CloneNotSupportedException e) {
throw new InternalError(); // This should never happen.
}
}
}
public class Main {
public static void main(String[] args) {
MyObject originalObject = new MyObject(42);
// Clone the object
MyObject clonedObject = originalObject.clone();
// Modify the original object
originalObject.setValue(99);
// Check if the clone is a distinct object
System.out.println("Original value: " + originalObject.getValue());
System.out.println("Cloned value: " + clonedObject.getValue());
}
}
In this example, we create a custom class MyObject
that implements the Cloneable
interface and overrides the clone()
method to create a shallow copy of the object. After cloning, changes to the original object do not affect the cloned object because they are separate instances with the same initial values.
It’s important to note that object cloning creates a shallow copy by default. If the class contains reference types, you might need to perform deep cloning if you want the entire object graph to be copied, rather than just copying references.