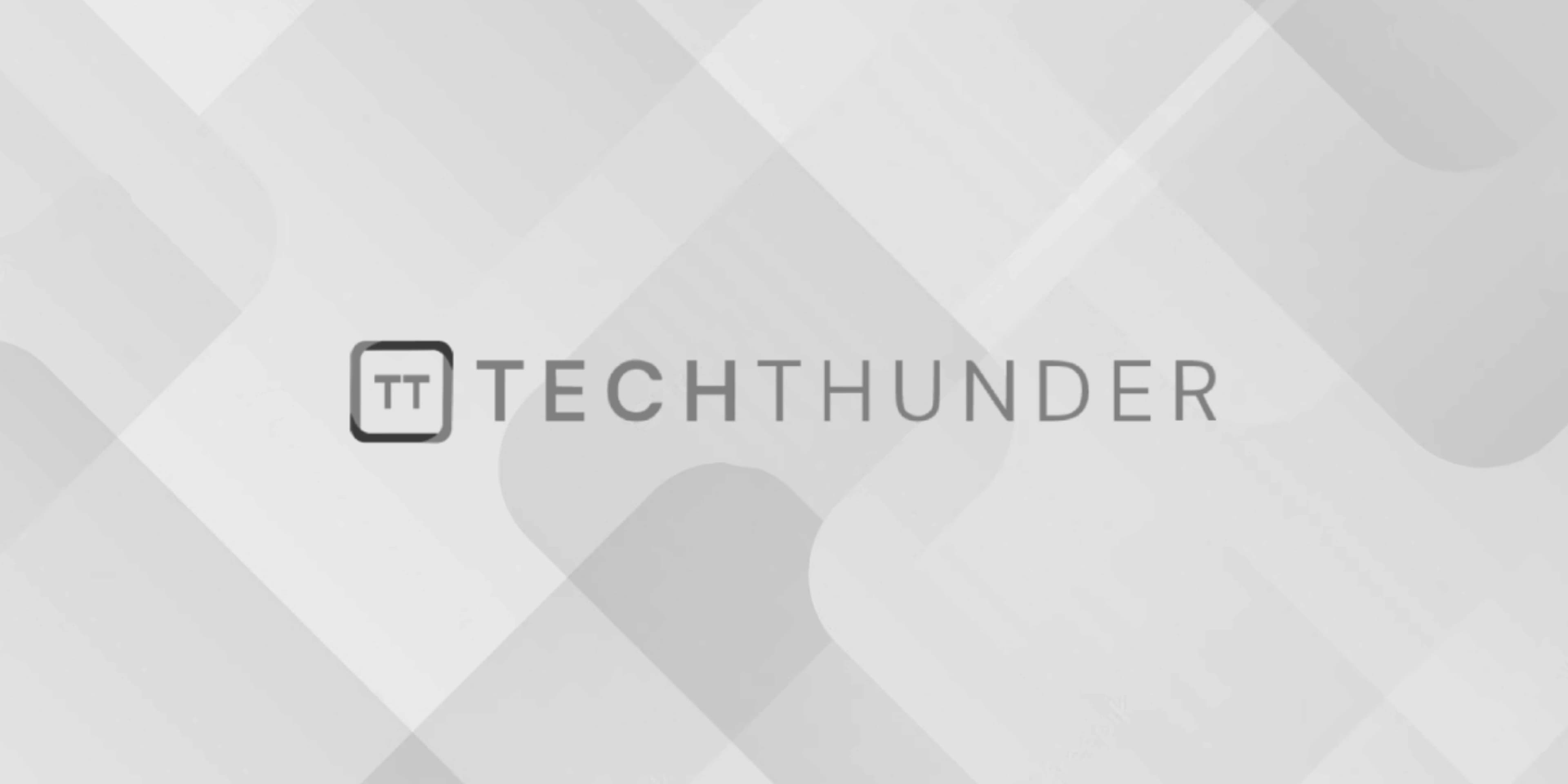
Java Object class
The Java Object
class is the root class for all other classes. It is part of the java.lang
package, and every class in Java is either directly or indirectly derived from the Object
class. This means that all classes inherit several methods from the Object
class, and these methods can be overridden and used in any Java class.
The Object
class provides several methods that can be overridden in derived classes to provide custom behavior. Some of the most commonly used methods in the Object
class are:
equals(Object obj)
:
- This method is used to compare the current object with the specified object for equality.
- By default, the
equals
method compares object references, but it’s often overridden in custom classes to provide meaningful comparison based on the object’s content.
hashCode()
:
- This method returns a hash code value for the object.
- The
hashCode
method is commonly overridden when theequals
method is overridden to ensure that equal objects have the same hash code.
toString()
:
- This method returns a string representation of the object.
- The
toString
method is frequently overridden to provide a human-readable representation of the object’s state.
getClass()
:
- This method returns the
Class
object for the current instance. - The
getClass
method can be used to retrieve information about the class of the object.
clone()
:
- This method is used to create a copy of the object. However, to use it, a class must implement the
Cloneable
interface and override theclone
method.
finalize()
:
- This method is called by the garbage collector before the object is reclaimed by the memory management system.
- It can be overridden to provide custom cleanup behavior, but it’s generally not recommended due to its unpredictable nature.
The Object
class also includes other methods for working with threads and synchronization, such as wait
, notify
, and notifyAll
, which are used for inter-thread communication.
Here’s an example of how to override the equals
and toString
methods in a custom class:
public class MyObject {
private int value;
public MyObject(int value) {
this.value = value;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
MyObject other = (MyObject) obj;
return value == other.value;
}
@Override
public int hashCode() {
return Objects.hash(value);
}
@Override
public String toString() {
return "MyObject{" +
"value=" + value +
'}';
}
}
By overriding these methods, you can provide customized behavior and representations for your objects. These are essential practices when creating your own classes to ensure meaningful comparisons and human-readable outputs.