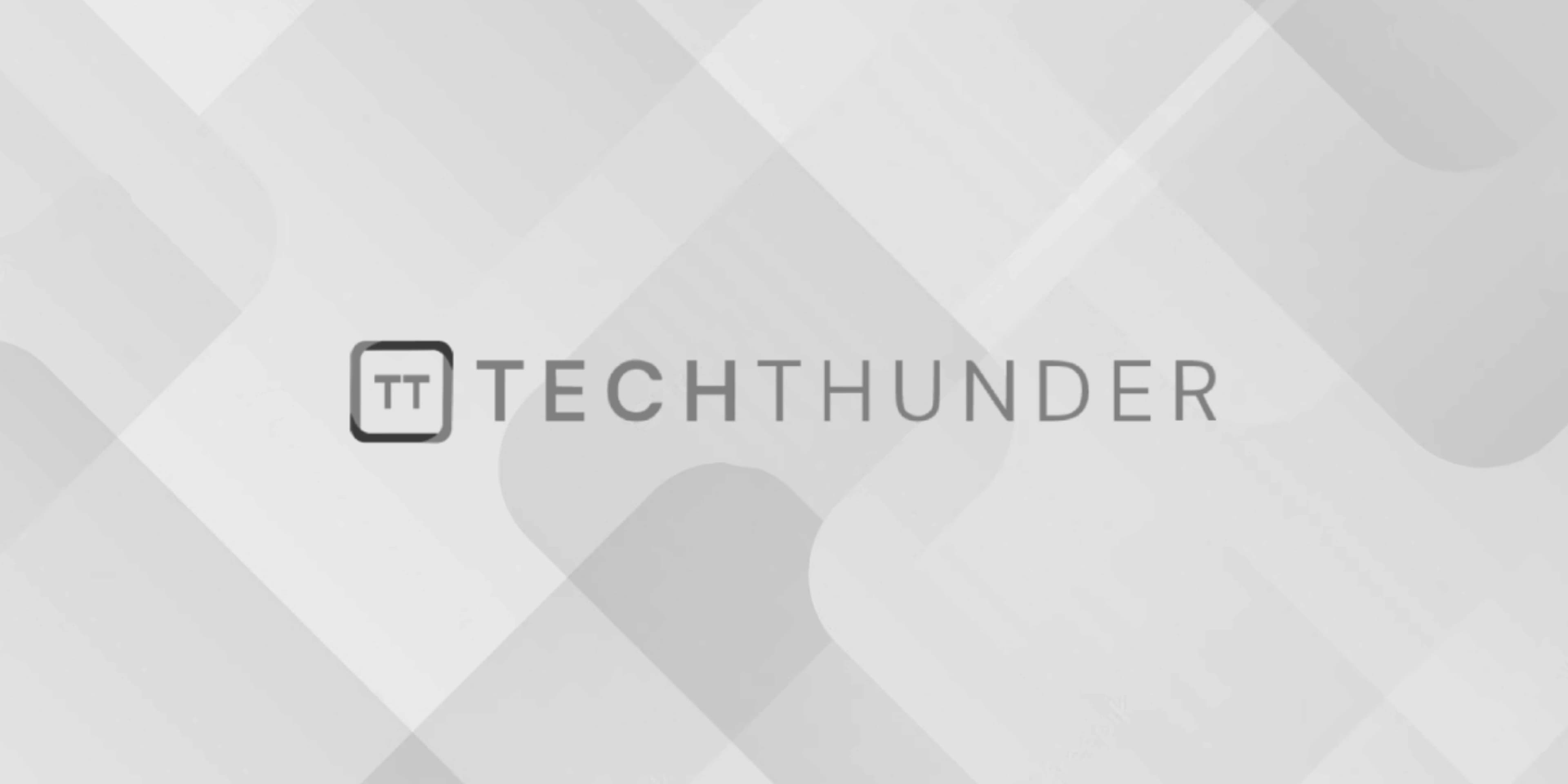
Java Switch
The switch
statement in Java is used for multi-way decision-making. It provides a way to select one of many code blocks to be executed, based on the value of an expression. Here’s the basic syntax of the switch
statement in Java:
switch (expression) {
case value1:
// Code to execute if expression equals value1
break; // Optional, used to exit the switch statement
case value2:
// Code to execute if expression equals value2
break;
// More cases...
default:
// Code to execute if none of the cases match
}
- The
expression
is evaluated, and the value is compared to eachcase
value. - If a
case
value matches theexpression
, the code block for that case is executed. - The
break
statement is optional but is used to exit theswitch
statement. Withoutbreak
, the execution would “fall through” to the nextcase
. - The
default
case is optional and is executed if none of thecase
values match theexpression
.
Here’s an example of using the switch
statement in Java to determine the day of the week based on an integer:
public class Main {
public static void main(String[] args) {
int day = 2;
switch (day) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday");
break;
case 3:
System.out.println("Tuesday");
break;
case 4:
System.out.println("Wednesday");
break;
case 5:
System.out.println("Thursday");
break;
case 6:
System.out.println("Friday");
break;
case 7:
System.out.println("Saturday");
break;
default:
System.out.println("Invalid day");
}
}
}
In this example, the value of the day
variable is compared to the case
values, and the corresponding day of the week is printed.
It’s important to use break
to exit the switch
statement when the desired case is found. Without break
, the execution will continue to the next case, which may lead to unexpected behavior.
The switch
statement is a useful tool for situations where you have a single expression to evaluate against multiple possible values. It provides a more structured and efficient alternative to using multiple if-else
statements.