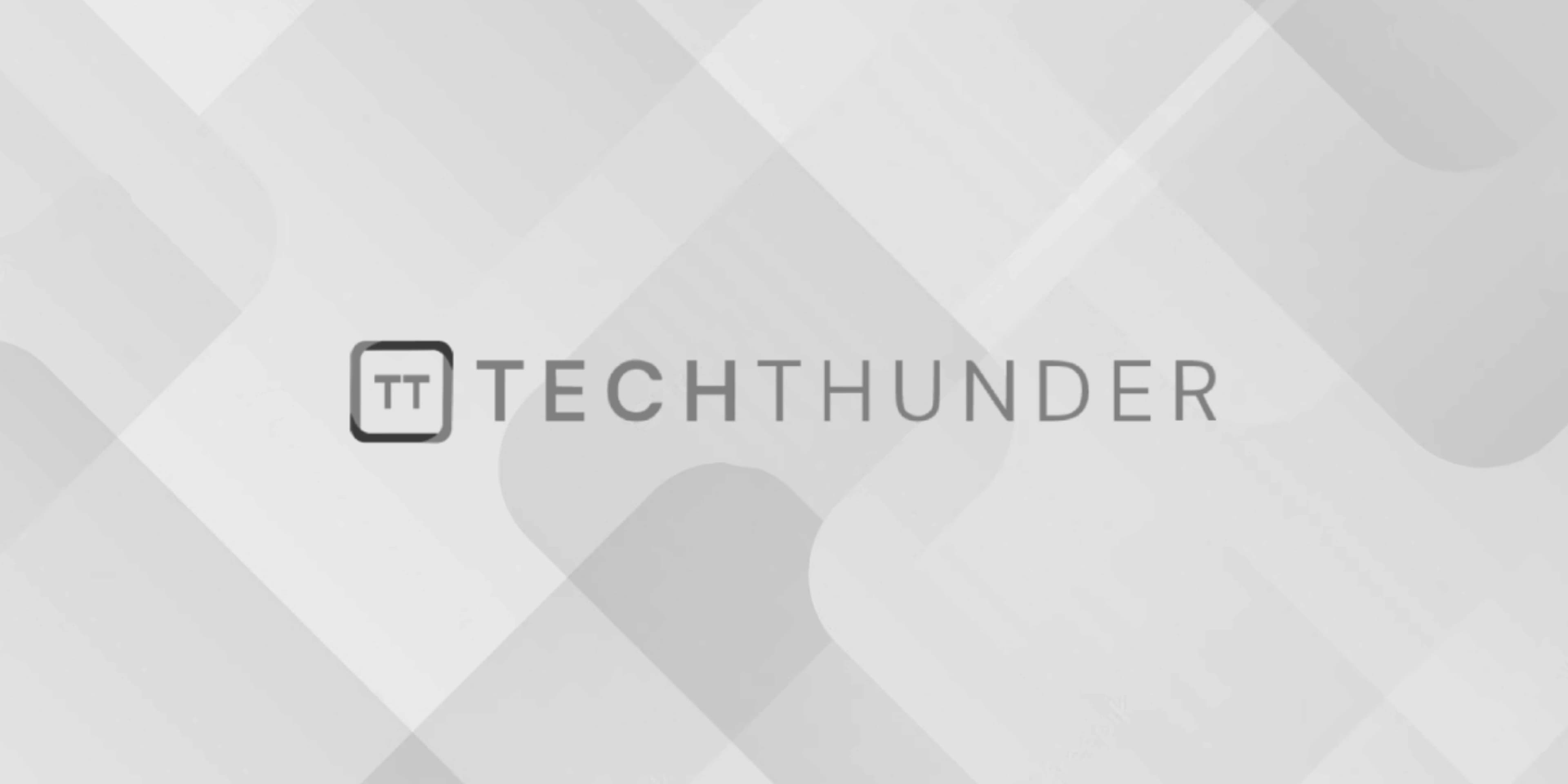
194 views
Java final keyword
The Java final
keyword is a modifier that can be applied to various elements, including classes, methods, variables, and parameters. The use of the final
keyword indicates that the marked element is unchangeable, immutable, or cannot be overridden, depending on where it’s used. Here’s an overview of how the final
keyword works in Java:
- Final Variables:
- When
final
is applied to a variable, it means the variable is a constant, and its value cannot be changed once it is assigned. This is often used for constants, which are typically declared in uppercase.
final int MAX_VALUE = 100;
- Final Methods:
- When a method is declared as
final
, it cannot be overridden by subclasses. This is often used to prevent the modification of a method’s behavior in a subclass.
class Parent {
final void someMethod() {
// Method implementation
}
}
- Final Classes:
- When a class is declared as
final
, it means it cannot be extended by other classes. Final classes are typically used for classes that should not have subclasses, such as utility classes or classes with a fixed implementation.
final class MyFinalClass {
// Class members and methods
}
- Final Parameters:
- When a parameter of a method is declared as
final
, it means that the parameter cannot be modified within the method. This is often used for method parameters to ensure their values are not changed accidentally.
void someMethod(final int value) {
// The 'value' parameter is final and cannot be modified here
}
- Final Fields in Objects:
- When an instance variable is declared as
final
within a class, it means that each instance of the class will have a constant, unchangeable field.
class ImmutableClass {
final int value;
ImmutableClass(int value) {
this.value = value;
}
}
- Final for Threads:
- In a multi-threaded context, the
final
keyword can be used to ensure safe publication of objects. When a reference to an object is marked asfinal
, it guarantees that the reference itself will not change, even though the object it points to might change.
class ThreadSafeExample {
final SomeObject sharedObject = new SomeObject();
}
The final
keyword is essential for ensuring immutability, providing security, and guaranteeing that certain elements in your code remain unaltered. It can also lead to better code optimization by the Java compiler and better performance in some cases. It’s a powerful tool for design and code quality.