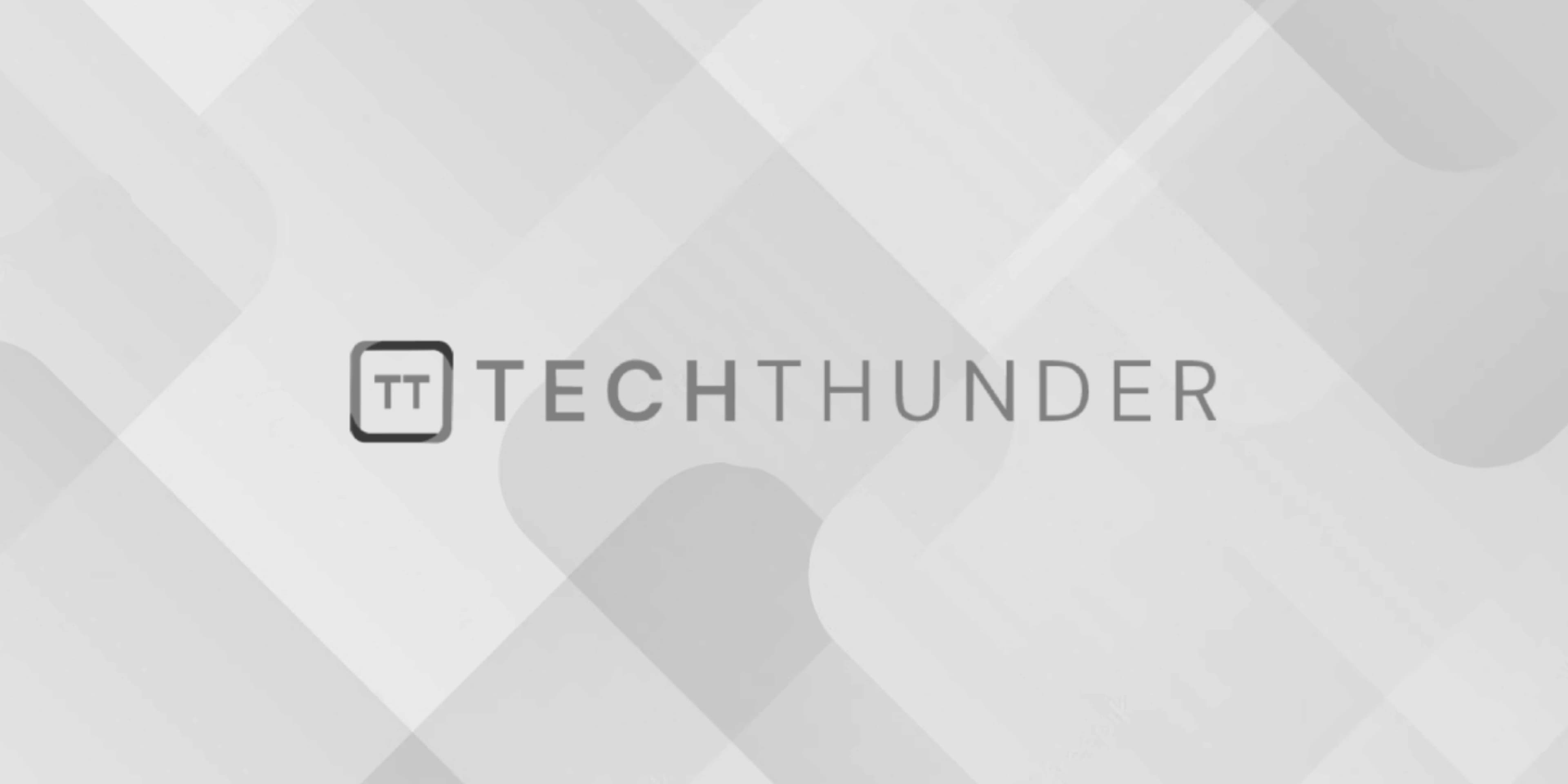
249 views
Java Program Internal
The internal structure of a Java program involves several key components and concepts. Understanding these components is essential when writing and running Java programs. Here’s an overview of the internal structure of a typical Java program:
- Package Declaration (Optional): A Java program may begin with an optional package declaration. A package is a way to organize related classes. If used, it’s specified at the very beginning of the file:
package mypackage;
- Import Statements (Optional): Import statements are used to bring classes or packages from other sources into the current program. They can be specified after the package declaration or at the beginning of the file:
import java.util.*; // Import all classes in the java.util package import java.io.File; // Import a specific class
- Class Definition: Every Java program consists of one or more classes. At least one of these classes must contain a
main
method, which serves as the entry point for the program:public class MyProgram { public static void main(String[] args) { // Program logic goes here } }
public
: Access modifier indicating that the class is accessible from outside the package.class
: Keyword indicating the beginning of a class definition.MyProgram
: The name of the class.
- Method Signature: The
main
method is where the program’s execution starts. It has the following signature:public
: Access modifier for visibility.static
: The method belongs to the class itself, not to instances of the class.void
: The return type (void means the method doesn’t return any value).main
: The name of the method.String[] args
: The method parameter, an array of strings used to pass command-line arguments to the program.
- Method Body: The method body contains the program’s logic, which is executed when the program runs. This is where you write your Java code:
public static void main(String[] args) { // Program logic goes here }
- Comments: Comments are used to provide explanations or documentation within the code. They are not executed and are for human understanding:
// This is a single-line comment /* This is a multi-line comment */
- Statements and Expressions: Within the method body, you write statements and expressions that define the program’s behavior.
- System.out.println(): Often used to output results or messages to the console. It’s a method provided by the
System
class in thejava.lang
package.System.out.println("Hello, Java!");
This is a basic structure for a Java program. The program logic goes inside the main
method, and you can include multiple classes, methods, and other components as needed to build more complex applications. Remember to compile your Java source code using the javac
command before running it with the java
command.