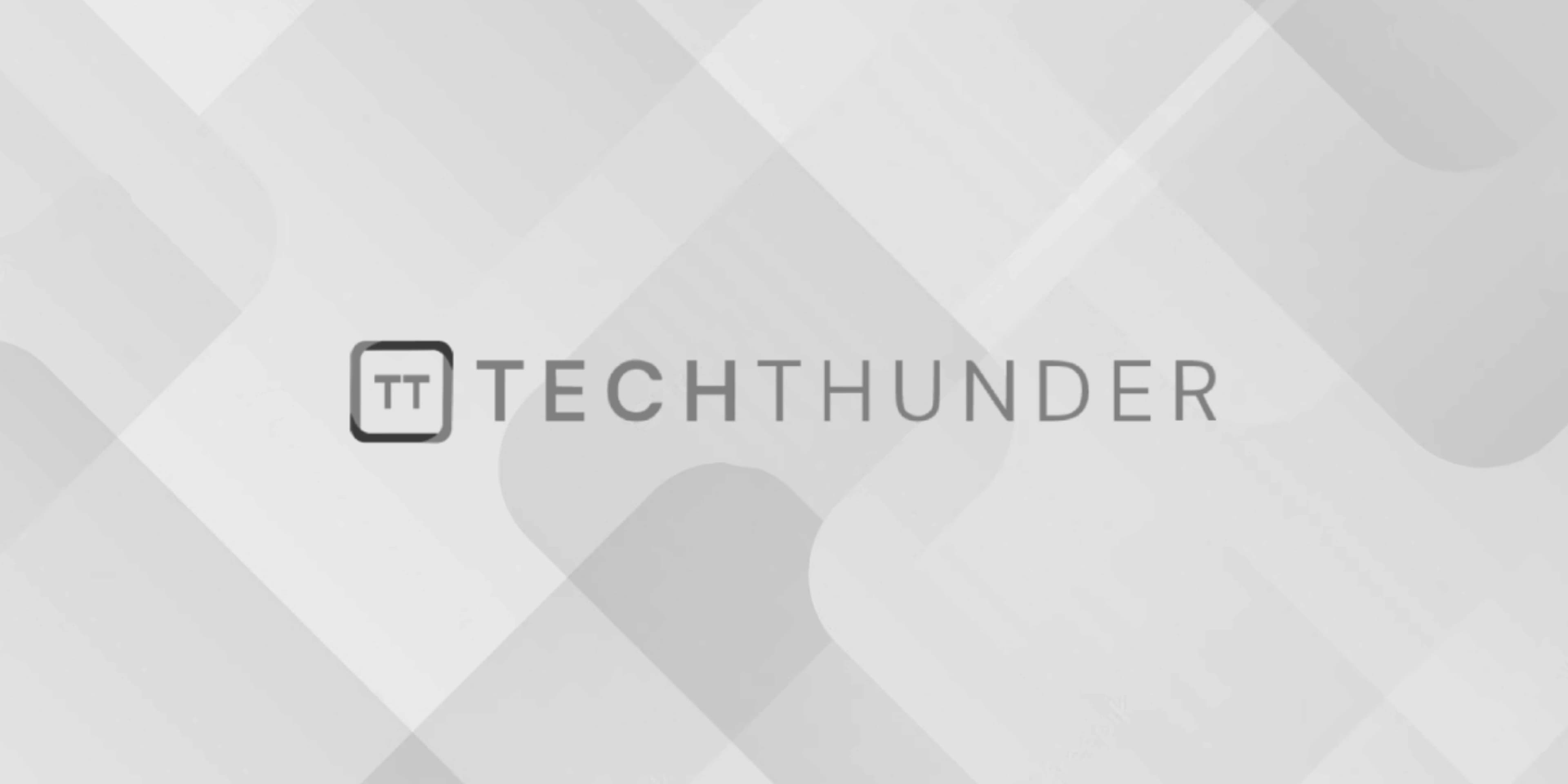
164 views
Java this keyword
The Java this
keyword is a reference to the current instance of a class, and it is used to access or refer to the instance’s fields, methods, and constructors. It is particularly useful when there is a need to disambiguate between instance variables and parameters or to distinguish between instance and local variables with the same name. Here’s how the this
keyword is used:
- Accessing Instance Variables:
- You can use
this
to refer to instance variables within a class, especially when there is a local variable with the same name, creating a naming conflict.
public class Person {
String name; // Instance variable
public void setName(String name) {
this.name = name; // Using 'this' to refer to the instance variable
}
}
- Invoking Constructors:
- When one constructor of a class wants to call another constructor of the same class,
this()
is used to achieve constructor chaining.
public class MyClass {
int value;
public MyClass() {
this(0); // Calls the parameterized constructor
}
public MyClass(int value) {
this.value = value;
}
}
- Passing
this
as an Argument:
- You can pass the current instance (
this
) as an argument to another method or constructor. This is often seen when implementing builder patterns or callback mechanisms.
public class Button {
public void onClick(ClickListener listener) {
listener.onClick(this); // Pass the current instance to the listener
}
}
- Returning
this
:
- A method can return the current instance (
this
) to allow method chaining. This is often seen in fluent APIs.
public class StringBuilder {
private StringBuilder buffer = new StringBuilder();
public StringBuilder append(String s) {
buffer.append(s);
return this; // Return 'this' to enable method chaining
}
}
- Method References:
- In Java 8 and later versions, you can use the
this
keyword in method references to refer to the current instance.
public class MyClass {
public void myMethod() {
Runnable runnable = this::anotherMethod; // Method reference to 'anotherMethod'
}
public void anotherMethod() {
// Method implementation
}
}
The this
keyword is a fundamental concept in Java, especially in the context of instance variables and constructors. It allows you to work with the current instance of a class and manage potential naming conflicts.