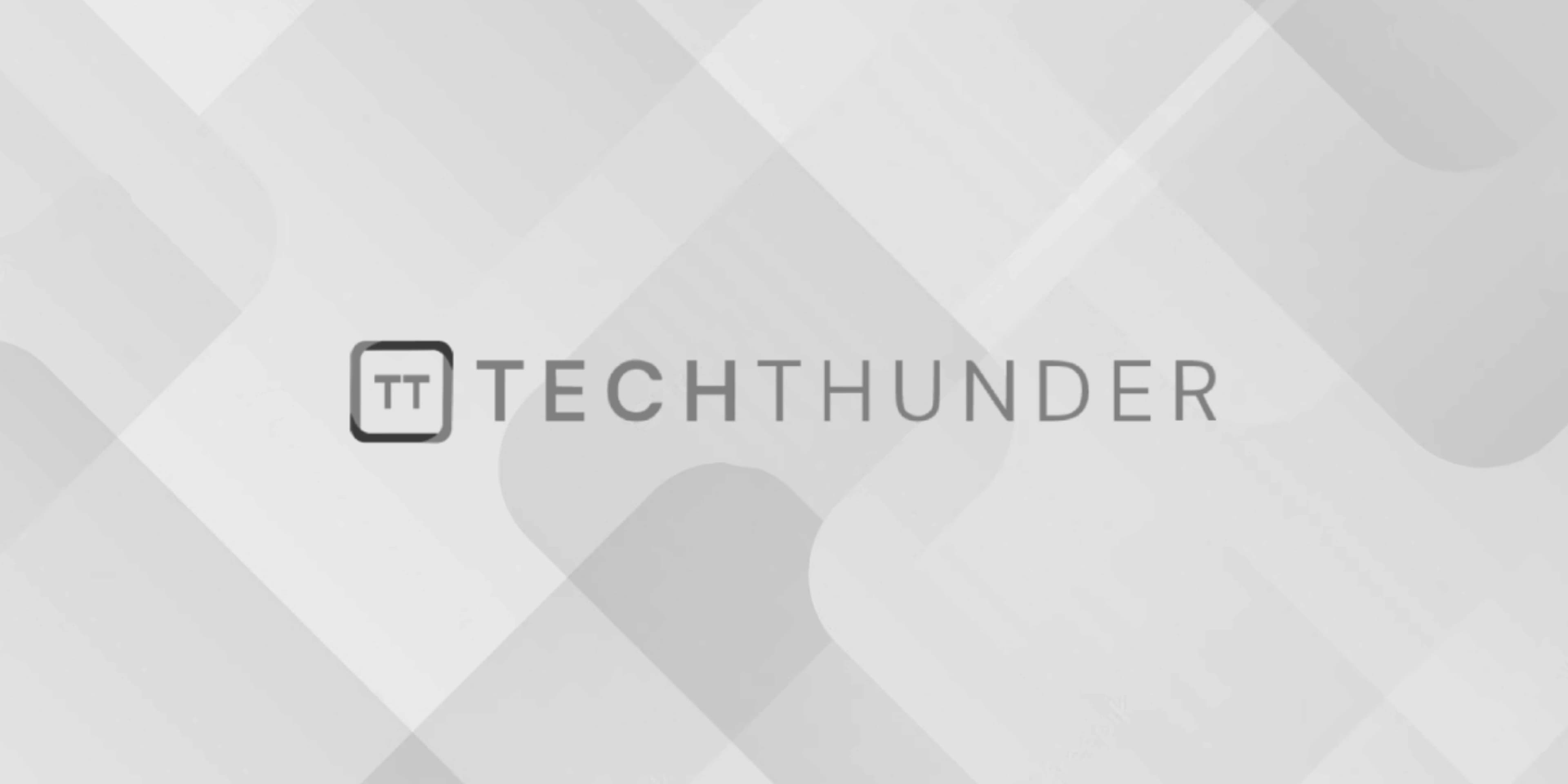
286 views
Java Constructor
The Java constructor is a special type of method that is used to initialize objects when they are created. Constructors have the same name as the class they belong to and do not have a return type, not even void
. They are called automatically when an object is instantiated and are used to set initial values and perform other setup tasks. Here are some key points about constructors in Java:
- Constructor Signature:
- A constructor has the same name as the class it belongs to.
- Constructors do not have a return type, not even
void
. - Multiple constructors can exist in a class, as long as they have different parameter lists. This is known as constructor overloading.
public class MyClass {
// Default constructor (no parameters)
public MyClass() {
// Constructor code here
}
// Parameterized constructor
public MyClass(int value) {
// Constructor code here
}
}
- Default Constructor:
- If you don’t provide any constructors in your class, Java automatically provides a default constructor with no parameters. This default constructor initializes the object but doesn’t perform any specific actions.
- You can also explicitly define a default constructor if needed.
public class MyClass {
// Default constructor (explicitly defined)
public MyClass() {
// Constructor code here
}
}
- Parameterized Constructor:
- A parameterized constructor accepts one or more parameters. It is used to initialize the object with values provided during object creation.
public class Employee {
private String name;
private int employeeId;
public Employee(String name, int employeeId) {
this.name = name;
this.employeeId = employeeId;
}
}
this
Keyword:
- Inside a constructor, the
this
keyword refers to the current object being created. It is used to distinguish between instance variables and constructor parameters when they have the same names.
public class Person {
private String name;
public Person(String name) {
this.name = name; // "this.name" refers to the instance variable, while "name" refers to the parameter
}
}
- Chaining Constructors (Constructor Overloading):
- You can have multiple constructors in a class with different parameter lists. This allows you to provide flexibility in object creation.
public class Student {
private String name;
private int age;
public Student(String name) {
this.name = name;
}
public Student(String name, int age) {
this(name); // Calls the single-argument constructor
this.age = age;
}
}
- Constructor Invocation:
- Constructors can invoke other constructors within the same class using the
this
keyword. This is known as constructor chaining.
public class MyClass {
private int value;
public MyClass() {
this(0); // Calls the parameterized constructor
}
public MyClass(int value) {
this.value = value;
}
}
Constructors are essential for initializing object state and setting up objects with the required values. They play a crucial role in Java’s object-oriented programming model.