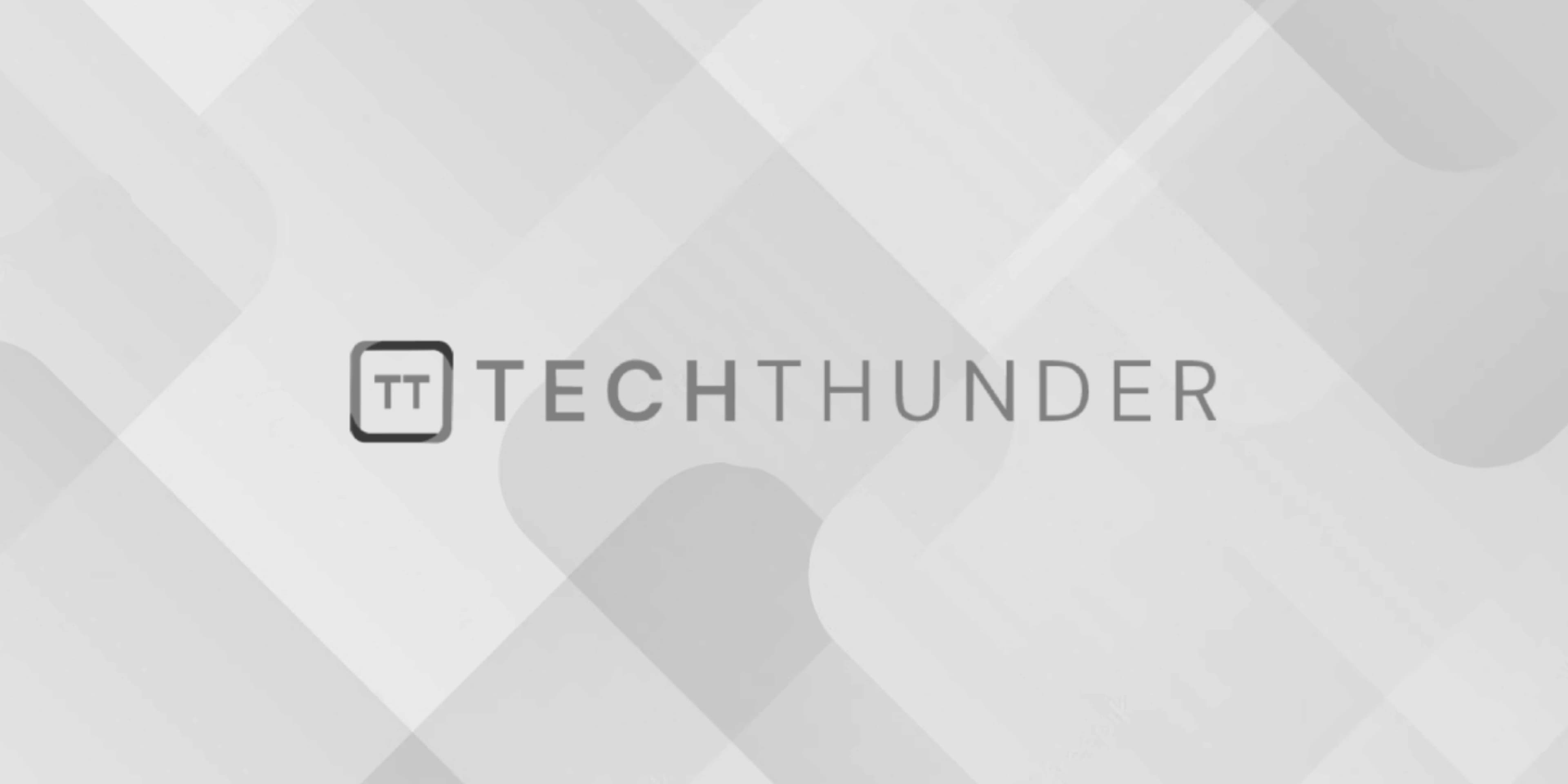
164 views
Java Method Overriding
Method overriding is a fundamental concept in Java’s object-oriented programming that allows a subclass to provide a specific implementation of a method that is already defined in its superclass. This is a way to achieve runtime polymorphism and enable the subclass to customize or extend the behavior of the inherited method.
Key points about method overriding in Java:
- Method Signature:
- To override a method, the subclass method must have the same method signature as the superclass method. This includes the method name, return type, and parameter types and order.
- Access Modifier:
- The access modifier for the overriding method cannot be more restrictive than the access modifier of the overridden method. In other words, you can’t reduce the visibility of the method in the subclass.
@Override
Annotation (Optional):
- While not required, it’s a good practice to use the
@Override
annotation when overriding a method. This annotation helps the compiler catch errors in case you mistakenly change the method signature.
- Exception Handling:
- If the overridden method throws a checked exception, the overriding method can throw the same, a subclass exception, or no exception (subclasses of the exception). However, it cannot throw a broader exception.
super
Keyword:
- Inside the overriding method, you can use the
super
keyword to call the overridden method from the superclass. This can be useful if you want to extend the behavior of the superclass method in the subclass.
- Return Type:
- In Java, method overriding is not based on the return type (except for covariant return types introduced in Java 5). The return type of the overriding method must be a subtype (subclass) of the return type of the overridden method.
Here’s an example demonstrating method overriding:
class Animal {
void makeSound() {
System.out.println("Some generic animal sound");
}
}
class Dog extends Animal {
@Override
void makeSound() {
System.out.println("Woof! Woof!");
}
void playFetch() {
System.out.println("Fetching the ball");
}
}
public class Main {
public static void main(String[] args) {
Animal animal = new Dog(); // Polymorphism: A Dog is treated as an Animal.
animal.makeSound(); // Calls the Dog's overridden method.
// animal.playFetch(); // This is not allowed because the reference type (Animal) does not have the playFetch method.
}
}
In this example, the Dog
class overrides the makeSound
method from the Animal
class. When an instance of Dog
is treated as an Animal
, it calls the overridden method from the Dog
class. Method overriding allows you to extend and specialize the behavior of a class in its subclasses, promoting code reusability and polymorphism.