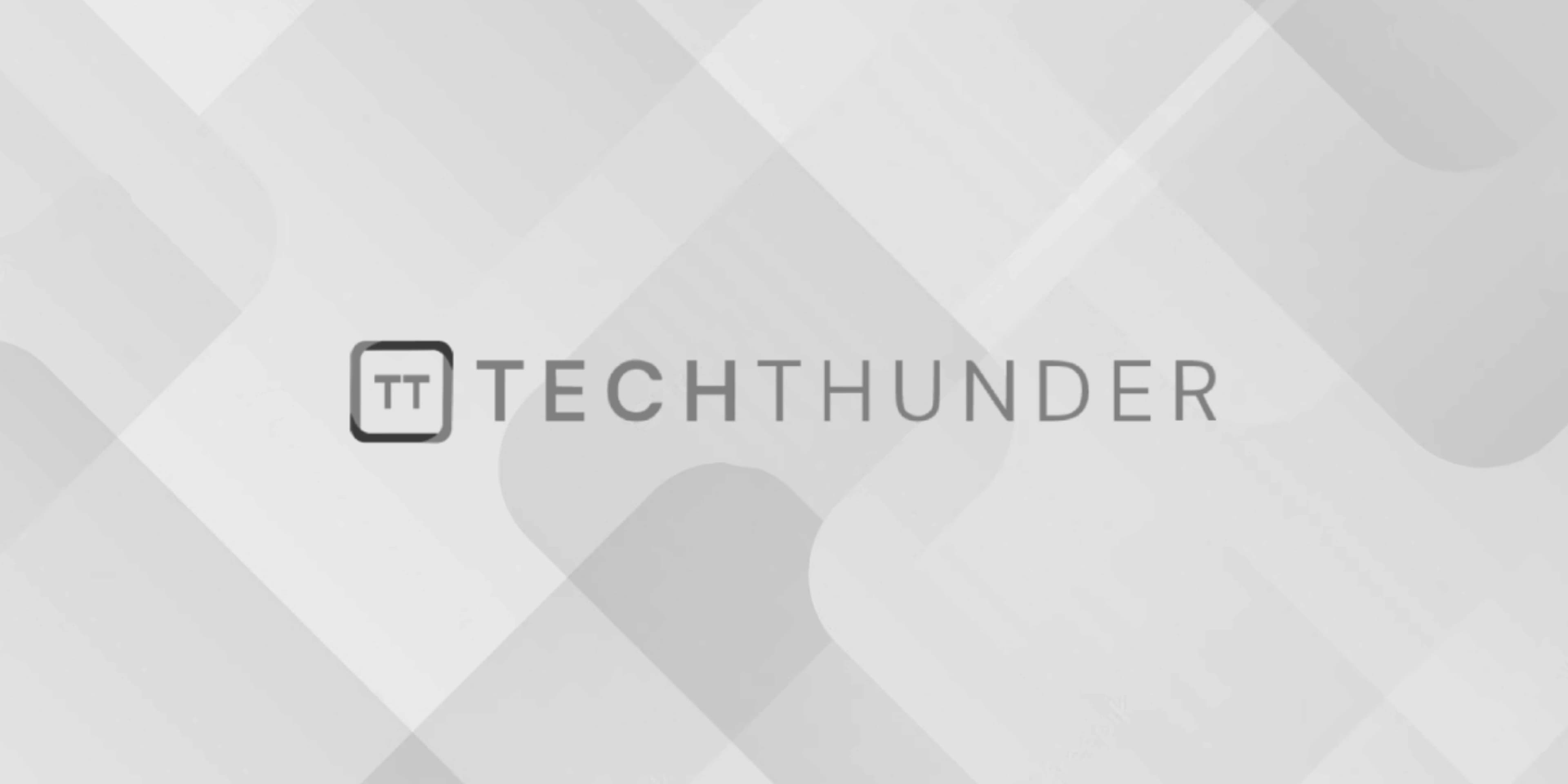
Java If-else
The if-else
statement in Java is used for decision-making in your code. It allows you to execute different blocks of code based on whether a specified condition is true or false. Here’s the basic syntax of the if-else
statement in Java:
if (condition) {
// Code to execute if the condition is true
} else {
// Code to execute if the condition is false
}
- The
condition
is an expression that is evaluated to eithertrue
orfalse
. - If the
condition
istrue
, the code block inside the first set of curly braces{}
is executed. - If the
condition
isfalse
, the code block inside the second set of curly braces{}
(theelse
block) is executed.
Here’s an example of how to use the if-else
statement in Java:
public class Main {
public static void main(String[] args) {
int age = 18;
if (age >= 18) {
System.out.println("You are an adult.");
} else {
System.out.println("You are not yet an adult.");
}
}
}
In this example, the age
variable is checked, and if it is greater than or equal to 18, the code inside the first block is executed, which prints “You are an adult.” If the age
is less than 18, the code inside the else
block is executed, which prints “You are not yet an adult.”
You can also use nested if-else
statements to handle multiple conditions. Here’s an example:
public class Main {
public static void main(String[] args) {
int score = 75;
if (score >= 90) {
System.out.println("A");
} else if (score >= 80) {
System.out.println("B");
} else if (score >= 70) {
System.out.println("C");
} else {
System.out.println("D");
}
}
}
In this example, the program evaluates the score
variable and prints the corresponding grade based on the conditions in the if-else if-else
ladder.
The if-else
statement is a fundamental building block for creating decision-making logic in Java programs. It allows you to control the flow of your program based on the evaluation of conditions.