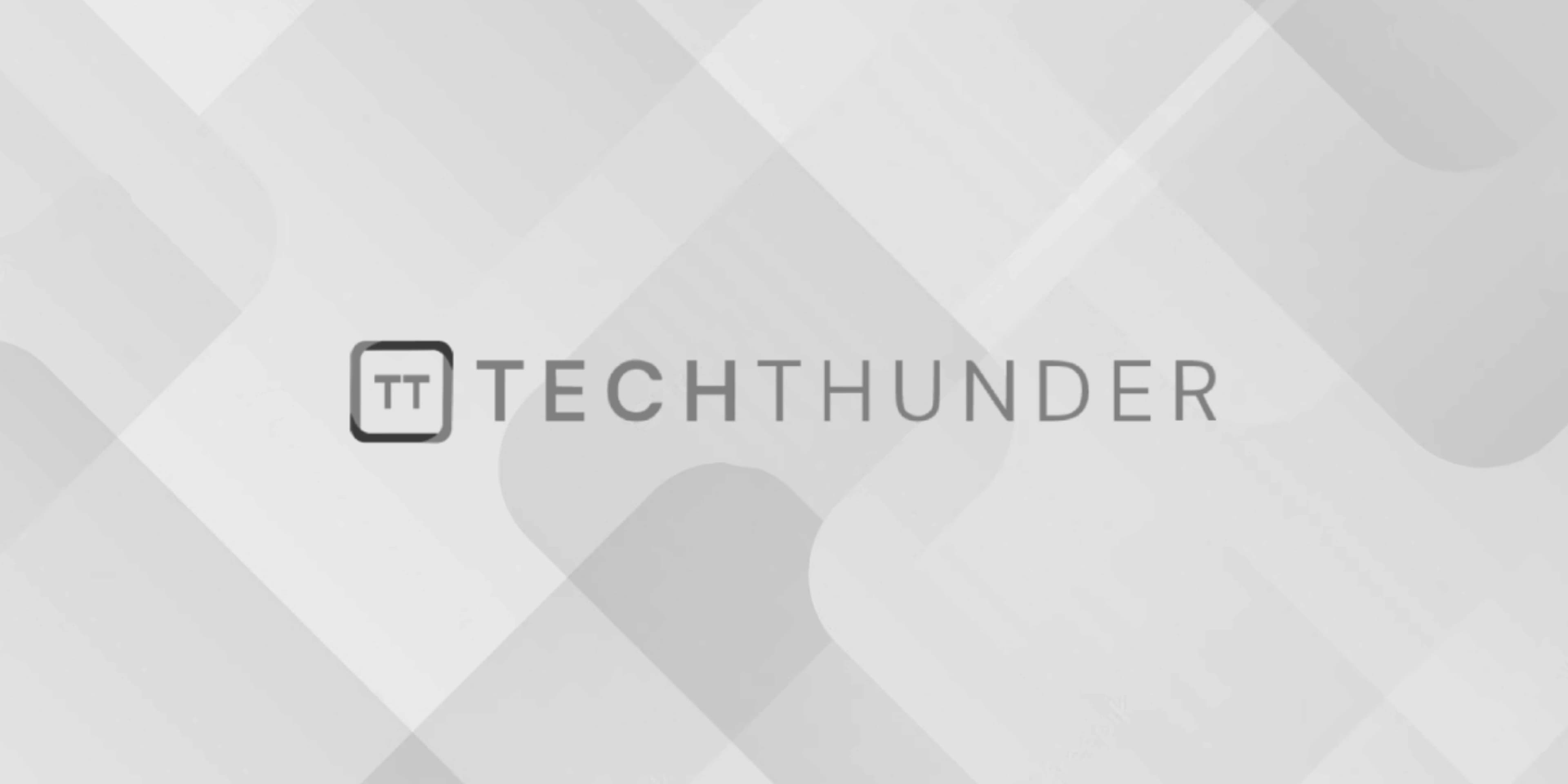
Java Collection
The Java Collections Framework provides a set of classes and interfaces for working with collections of objects. Collections are used to store, manipulate, and manage groups of related objects. The framework offers a wide range of data structures and algorithms for various collection types, making it easier to work with data in a structured manner. The Java Collections Framework is part of the java.util
package.
Some key interfaces and classes in the Java Collections Framework include:
- Collection: The root interface for all collection types. It defines the basic methods common to all collections, such as adding, removing, and iterating over elements.
- List: An ordered collection that allows duplicate elements. Common implementations include
ArrayList
andLinkedList
. - Set: A collection that does not allow duplicate elements. Common implementations include
HashSet
andTreeSet
. - Map: A collection of key-value pairs. It associates keys with values, and each key must be unique. Common implementations include
HashMap
andTreeMap
. - Queue: A collection that holds elements prior to processing. Common implementations include
LinkedList
(used as a queue) andPriorityQueue
. - Deque: A double-ended queue that allows efficient insertion and removal at both ends. Common implementations include
LinkedList
andArrayDeque
. - Iterator: An interface used to traverse elements in a collection.
- Iterable: An interface that defines an iteration over a collection. It’s implemented by classes whose instances can be iterated.
Here’s a simple example of using the Java Collections Framework:
import java.util.ArrayList;
import java.util.List;
public class CollectionExample {
public static void main(String[] args) {
// Creating a list
List<String> names = new ArrayList<>();
// Adding elements
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Accessing elements
System.out.println("Names: " + names);
// Iterating over elements
for (String name : names) {
System.out.println(name);
}
// Removing an element
names.remove("Bob");
System.out.println("Names after removal: " + names);
}
}
The Java Collections Framework provides a consistent and flexible way to work with collections of data. Depending on your needs, you can choose the appropriate collection type that suits your use case, and the framework provides methods to efficiently manipulate and access the data.