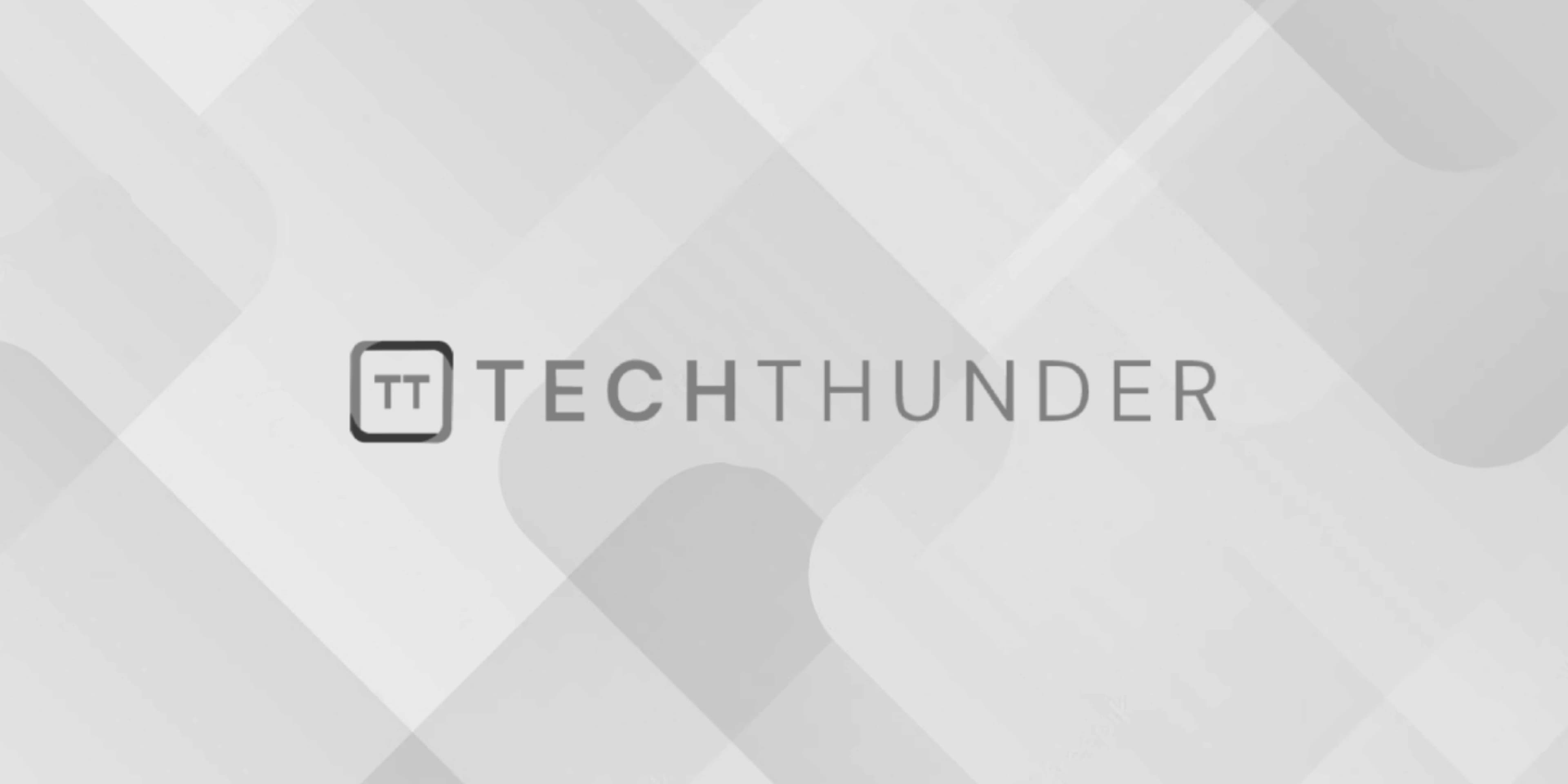
Java Call By Value
The Java method arguments are passed by value. This means that when you pass an argument to a method, you are actually passing a copy of the value, not the original variable. This can sometimes lead to confusion, especially when you’re working with objects and references.
Here’s how call by value works in Java:
- Primitive Data Types: When you pass a primitive data type (e.g.,
int
,double
,char
) as an argument to a method, you are passing a copy of the actual value. Any changes made to the parameter within the method do not affect the original variable outside of the method. Here’s an example:
public class CallByValueExample {
public static void main(String[] args) {
int x = 10;
modifyValue(x);
System.out.println(x); // Output: 10
}
public static void modifyValue(int value) {
value = 20; // Changes made to 'value' do not affect 'x'
}
}
- Objects and References: When you pass an object as an argument, you are passing the reference to the object. The reference is copied, not the object itself. This means that changes made to the object’s properties within the method will affect the original object outside the method. However, reassigning the reference within the method will not change the original reference outside the method. Here’s an example:
public class CallByValueExample {
public static void main(String[] args) {
Person person = new Person("Alice");
modifyPersonName(person);
System.out.println(person.getName()); // Output: Bob
}
public static void modifyPersonName(Person p) {
p.setName("Bob"); // Changes made to the object via the reference 'p' affect the original object
p = new Person("Charlie"); // Reassigning 'p' does not affect the original reference
}
}
The modifyPersonName
method can modify the properties of the Person
object through the reference, but reassigning p
to a new object does not affect the original person
reference.
Java uses call by value for both primitive data types and object references. When you pass a primitive data type, you’re working with a copy of the value, and changes don’t affect the original variable. When you pass an object reference, you’re working with a copy of the reference, and changes to the object’s properties do affect the original object, but reassigning the reference itself does not.