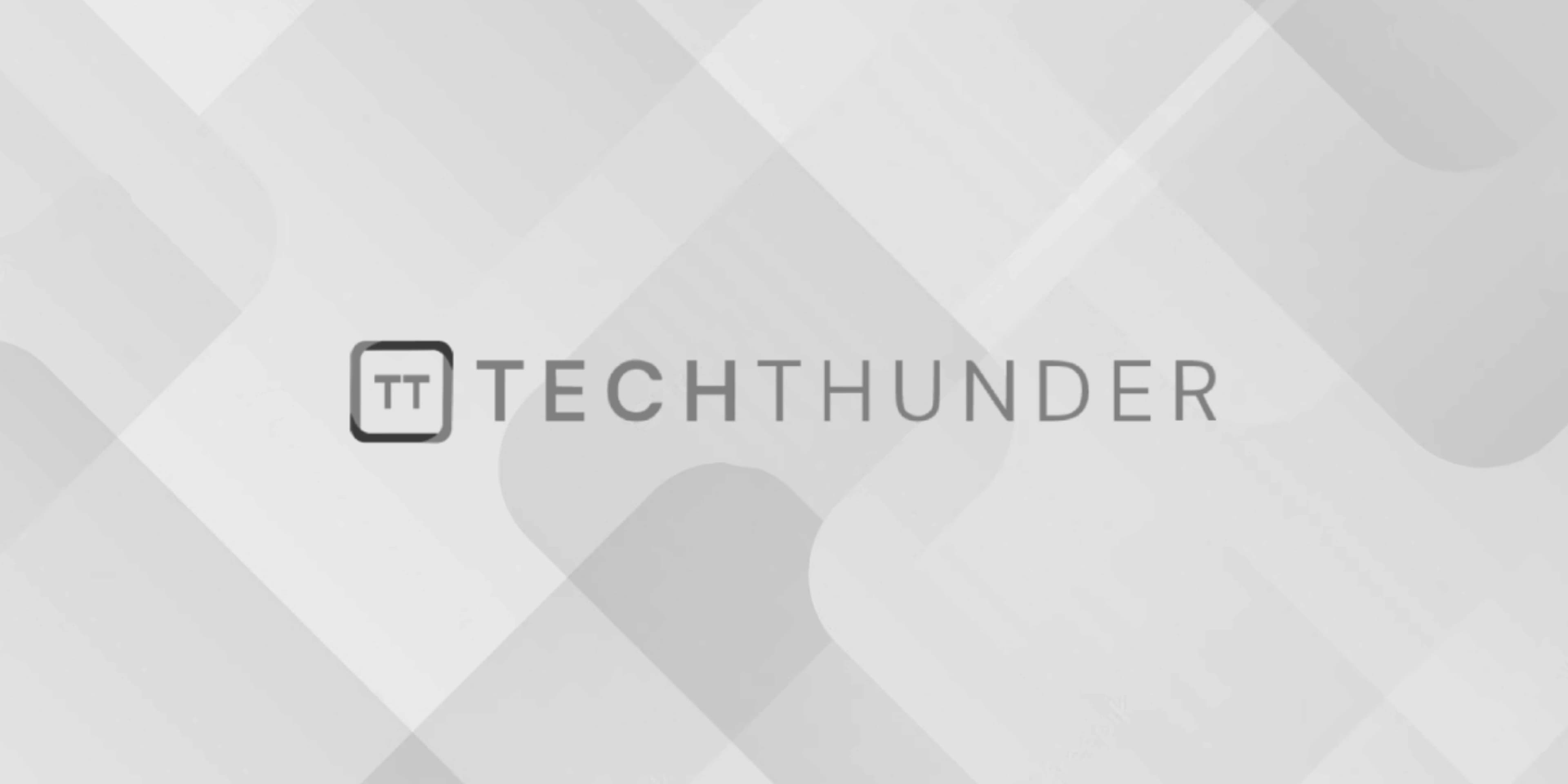
209 views
Java Continue
The Java continue
statement is used inside loops (such as for
, while
, and do-while
) to skip the current iteration and move on to the next one. It’s mainly used to control the flow of a loop by skipping the remaining code within the current iteration and proceeding to the next iteration of the loop. Here’s how the continue
statement works:
for (int i = 1; i <= 5; i++) {
if (i == 3) {
continue; // Skip the current iteration when i is 3
}
System.out.println("Iteration " + i);
}
In this example, when the continue
statement is encountered inside the loop, the current iteration (when i
is 3) is terminated, and the loop moves on to the next iteration. As a result, the output will be:
Iteration 1
Iteration 2
Iteration 4
Iteration 5
Key points to note about the continue
statement:
- Usage in Loops: The
continue
statement is used within loops and cannot be used outside of them. It allows you to control the flow of the loop while the loop is still in progress. - Skips Code: When a
continue
statement is executed, the remaining code in the current iteration is skipped, and the loop proceeds to the next iteration. - Use Cases: Common use cases for
continue
include skipping specific iterations based on certain conditions or implementing a form of filtering within a loop. - Labeling Loops: In nested loops, you can use labels to specify which loop the
continue
statement should affect. This is known as a labeledcontinue
. For example,outerLoop:
is a label for the outer loop.
outerLoop: for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
if (i == 2 && j == 2) {
continue outerLoop; // Continue the outer loop when i is 2 and j is 2
}
System.out.println("i = " + i + ", j = " + j);
}
}
- Avoid Infinite Loops: Be cautious when using the
continue
statement, as it can lead to infinite loops if not used correctly. Ensure that there is a way to make progress toward the loop’s exit condition.
The continue
statement is a valuable tool for fine-tuning the behavior of loops by allowing you to skip specific iterations when necessary.