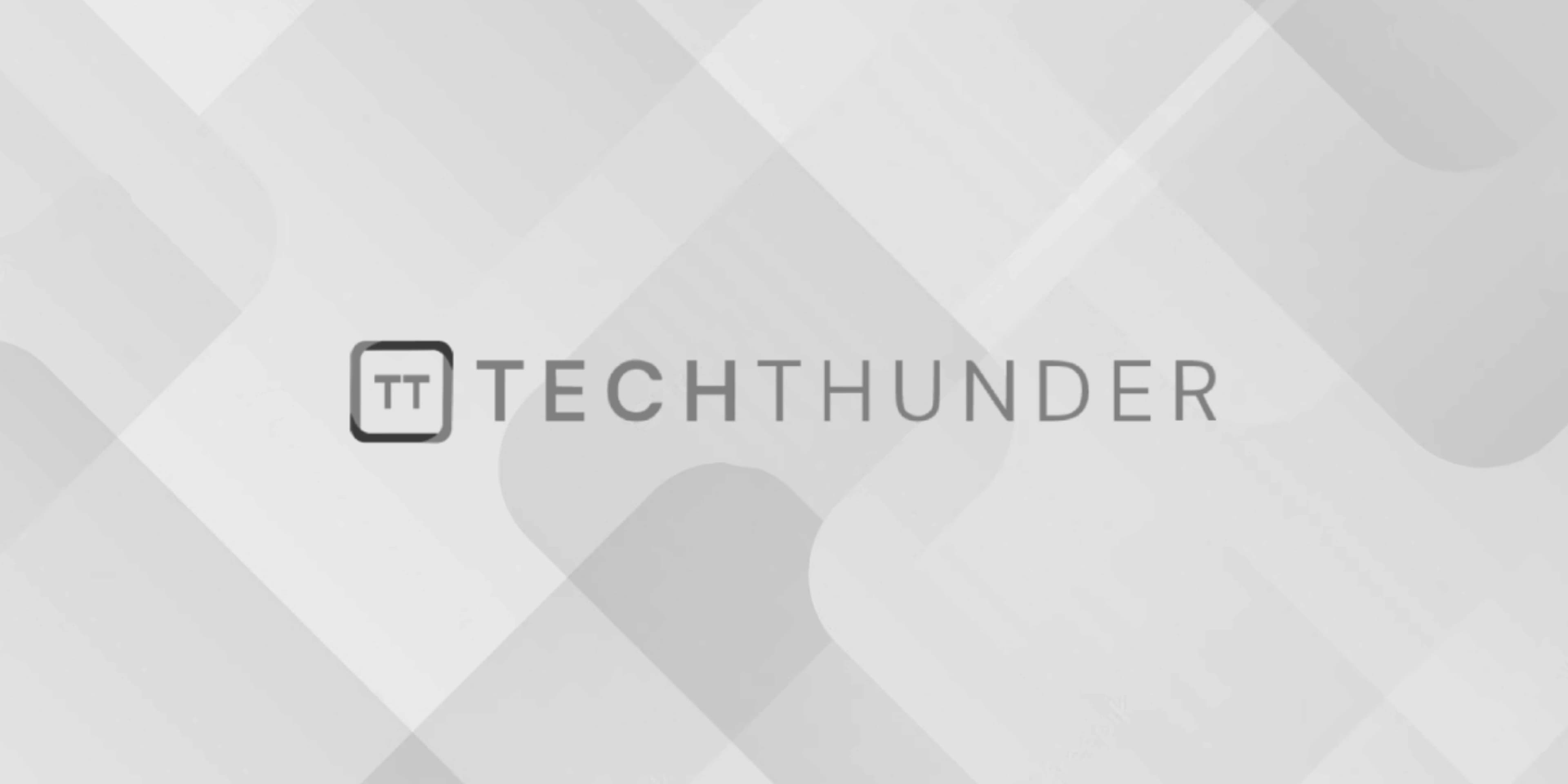
220 views
Java Operators
Operators in Java are symbols or special tokens that perform operations on variables and values. Java provides a variety of operators that can be categorized into several groups based on their functionality. Here are the primary categories of operators in Java:
- Arithmetic Operators:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder)
- Assignment Operators:
=
: Assignment+=
: Addition assignment-=
: Subtraction assignment*=
: Multiplication assignment/=
: Division assignment%=
: Modulus assignment
- Increment/Decrement Operators:
++
: Increment by 1--
: Decrement by 1
- Comparison Operators (Relational Operators):
==
: Equal to!=
: Not equal to>
: Greater than<
: Less than>=
: Greater than or equal to<=
: Less than or equal to
- Logical Operators:
&&
: Logical AND||
: Logical OR!
: Logical NOT
- Bitwise Operators:
&
: Bitwise AND|
: Bitwise OR^
: Bitwise XOR (exclusive OR)~
: Bitwise NOT (complement)<<
: Left shift>>
: Right shift (signed)>>>
: Right shift (unsigned)
- Conditional Operator (Ternary Operator):
? :
: Conditional (Ternary) Operatorcondition ? expression1 : expression2
- If the condition is true, it evaluates to
expression1
; otherwise, it evaluates toexpression2
.
- Instanceof Operator:
instanceof
: Tests if an object is an instance of a particular class or interface.
- Type Cast Operator:
(type)
: Used to cast or convert a value from one data type to another.
- Other Operators:
[]
: Array access (used to access elements in an array).
: Member access (used to access members of a class or object)new
: Object creation::
: Method reference::
: Method reference to an instance method of an object
Here’s an example that demonstrates the use of various operators in Java:
public class OperatorExample {
public static void main(String[] args) {
int a = 10, b = 5;
boolean condition = true;
// Arithmetic operators
int sum = a + b;
int difference = a - b;
int product = a * b;
int division = a / b;
int remainder = a % b;
// Assignment operators
a += 5; // Equivalent to a = a + 5
// Increment and decrement operators
a++;
b--;
// Comparison operators
boolean isEqual = (a == b);
boolean isGreaterThan = (a > b);
// Logical operators
boolean andResult = condition && (a > b);
boolean orResult = condition || (a > b);
boolean notResult = !condition;
System.out.println("Sum: " + sum);
System.out.println("Difference: " + difference);
System.out.println("Product: " + product);
System.out.println("Division: " + division);
System.out.println("Remainder: " + remainder);
System.out.println("Incremented a: " + a);
System.out.println("Is a equal to b? " + isEqual);
System.out.println("Is a greater than b? " + isGreaterThan);
System.out.println("Logical AND result: " + andResult);
System.out.println("Logical OR result: " + orResult);
System.out.println("Logical NOT result: " + notResult);
}
}
Operators play a crucial role in Java programming for performing a wide range of operations, from simple arithmetic calculations to complex logical and bitwise manipulations.