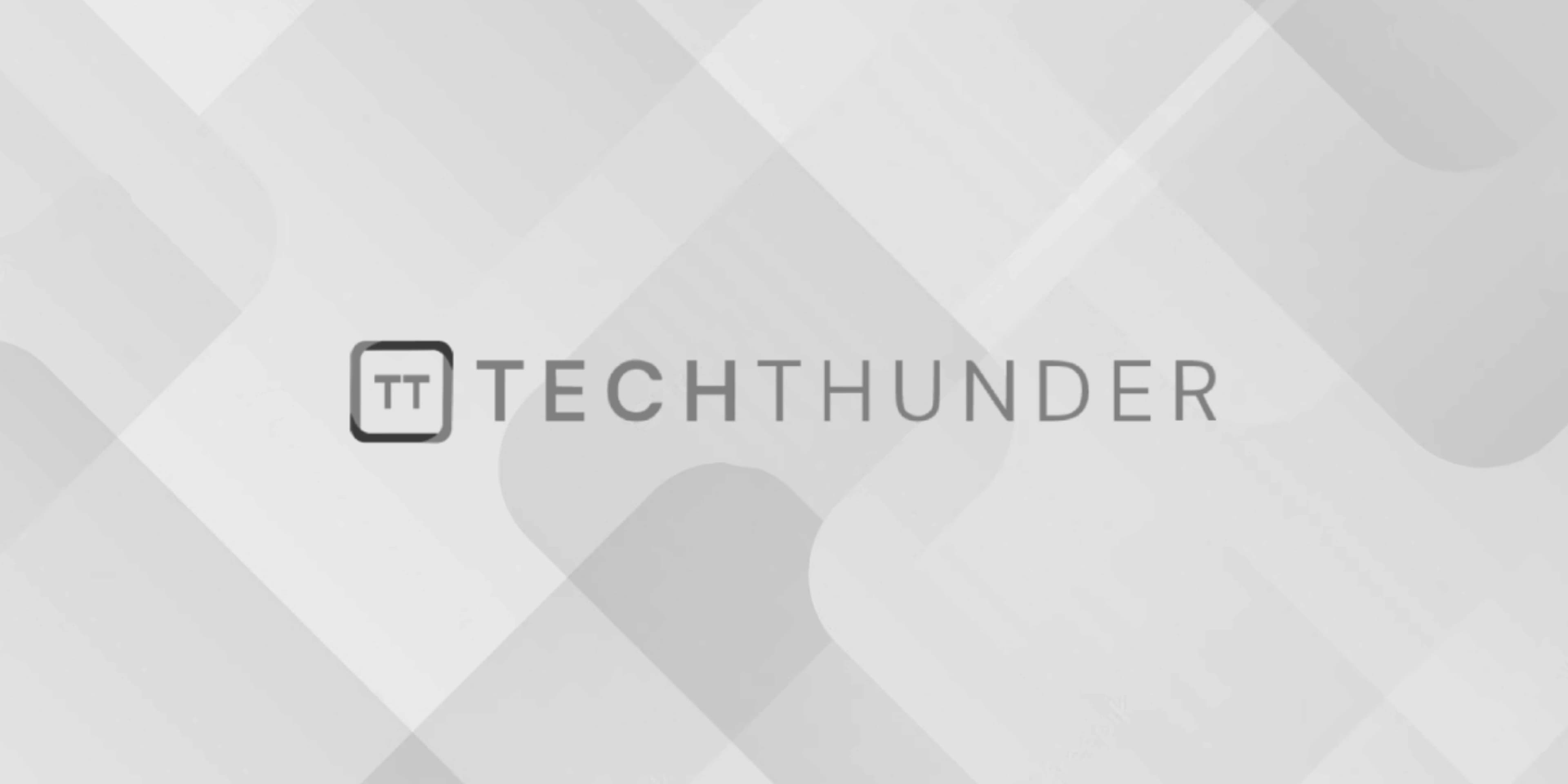
208 views
Java Conversion
It seems you’re referring to data type conversion in Java. Data type conversion, also known as type casting or type conversion, involves changing the data type of a value from one type to another. Java supports two types of data type conversion: implicit (automatic) conversion and explicit (manual) conversion.
- Implicit (Automatic) Conversion:
Implicit conversion, also known as widening conversion, occurs when a smaller data type is automatically converted to a larger data type without any loss of information. For example, converting anint
to adouble
.
int intValue = 42;
double doubleValue = intValue; // Implicit conversion from int to double
- Explicit (Manual) Conversion:
Explicit conversion, also known as type casting, involves manually converting a data type to another data type that might have a smaller range or precision. This might result in loss of information or potential errors.
double doubleValue = 3.14159;
int intValue = (int) doubleValue; // Explicit conversion from double to int
Be cautious when using explicit conversion, as it can lead to loss of precision or unexpected results.
- String Conversion:
Converting data types to and from strings is common in Java. You can use methods likevalueOf()
andtoString()
for conversions.
int intValue = 42;
String strValue = String.valueOf(intValue); // Convert int to String
String strNumber = "123";
int parsedInt = Integer.parseInt(strNumber); // Convert String to int
- Wrapper Class Conversion:
Primitive data types in Java have corresponding wrapper classes that can be used for conversion. These classes provide methods for converting between primitives and objects.
int intValue = 42;
Integer integerValue = Integer.valueOf(intValue); // Convert int to Integer
double doubleValue = integerValue.doubleValue(); // Convert Integer to double
Remember:
- Implicit conversion usually occurs between compatible types, like widening conversions.
- Explicit conversion requires type casting, but be aware of potential loss of information.
- String conversion is common for input/output and interaction with user interfaces.
- Wrapper classes provide methods for conversion and can be useful when working with objects.
Choose the appropriate type conversion based on your requirements and the compatibility of the data types involved.