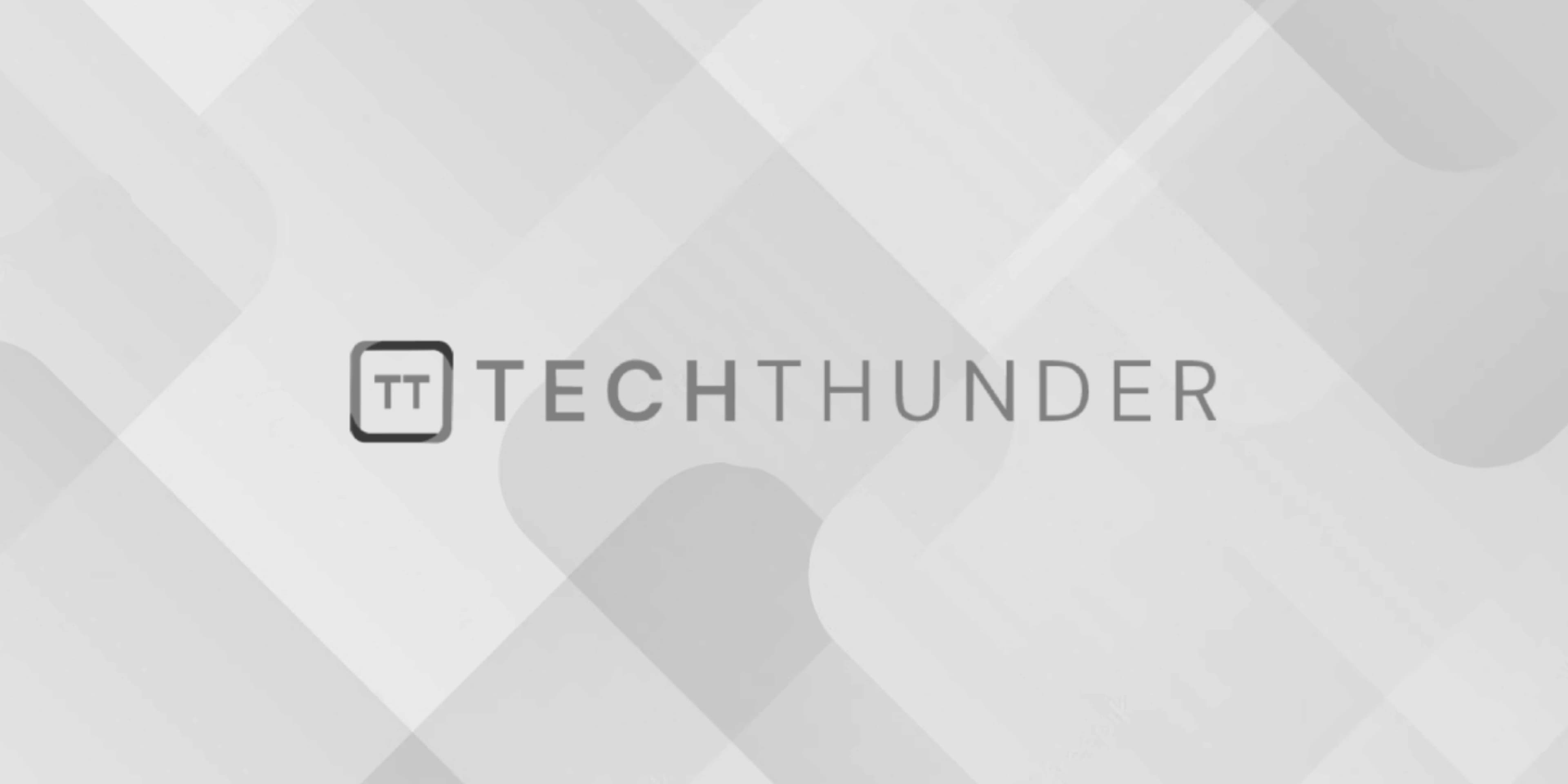
306 views
Java OOPs Concepts
The Object-Oriented Programming (OOP) is a fundamental programming paradigm that is widely used in Java and many other modern programming languages. Java is known for its strong support of OOP principles. Here are the core OOP concepts in Java:
- Classes and Objects:
- A class is a blueprint or template for creating objects. An object is an instance of a class. Classes define the structure (attributes and methods) of objects.
class Car {
String make;
String model;
int year;
void start() {
System.out.println("Car started.");
}
}
Car myCar = new Car(); // Creating an object
myCar.make = "Toyota";
myCar.model = "Camry";
myCar.year = 2022;
myCar.start();
- Encapsulation:
- Encapsulation is the concept of bundling data (attributes) and methods (functions) that operate on that data within a single unit, known as a class. Access to the internal data is controlled through access modifiers.
public class Student {
private String name;
private int age;
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
- Inheritance:
- Inheritance allows a new class (subclass or derived class) to inherit properties and behaviors from an existing class (superclass or base class). This promotes code reuse and establishes an “is-a” relationship.
class Animal {
void eat() {
System.out.println("Animal is eating.");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog is barking.");
}
}
- Polymorphism:
- Polymorphism means “many forms.” It allows objects of different classes to be treated as objects of a common superclass. It’s achieved through method overriding and method overloading.
class Shape {
void draw() {
System.out.println("Drawing a shape.");
}
}
class Circle extends Shape {
void draw() {
System.out.println("Drawing a circle.");
}
}
Shape myShape = new Circle(); // Polymorphism
myShape.draw(); // Calls the overridden method in the Circle class.
- Abstraction:
- Abstraction is the process of simplifying complex reality by modeling classes based on the essential attributes and behaviors. It hides the complex details and shows only the necessary features of an object.
abstract class Vehicle {
abstract void start(); // Abstract method
}
class Car extends Vehicle {
void start() {
System.out.println("Car started.");
}
}
- Association, Aggregation, and Composition:
- These are relationship types between classes.
- Association represents a simple relationship where one class is related to another.
- Aggregation represents a “whole-part” relationship, where one class is composed of multiple other classes.
- Composition is a strong “whole-part” relationship, implying that the part cannot exist without the whole.
- Interfaces:
- An interface defines a contract that a class must adhere to by implementing its methods. A class can implement multiple interfaces, achieving multiple inheritance of behavior.
interface Drawable {
void draw();
}
class Circle implements Drawable {
void draw() {
System.out.println("Drawing a circle.");
}
}
These OOP principles promote code organization, reusability, and maintainability. Java’s OOP features help structure and design programs effectively, making it a powerful language for developing complex software systems.