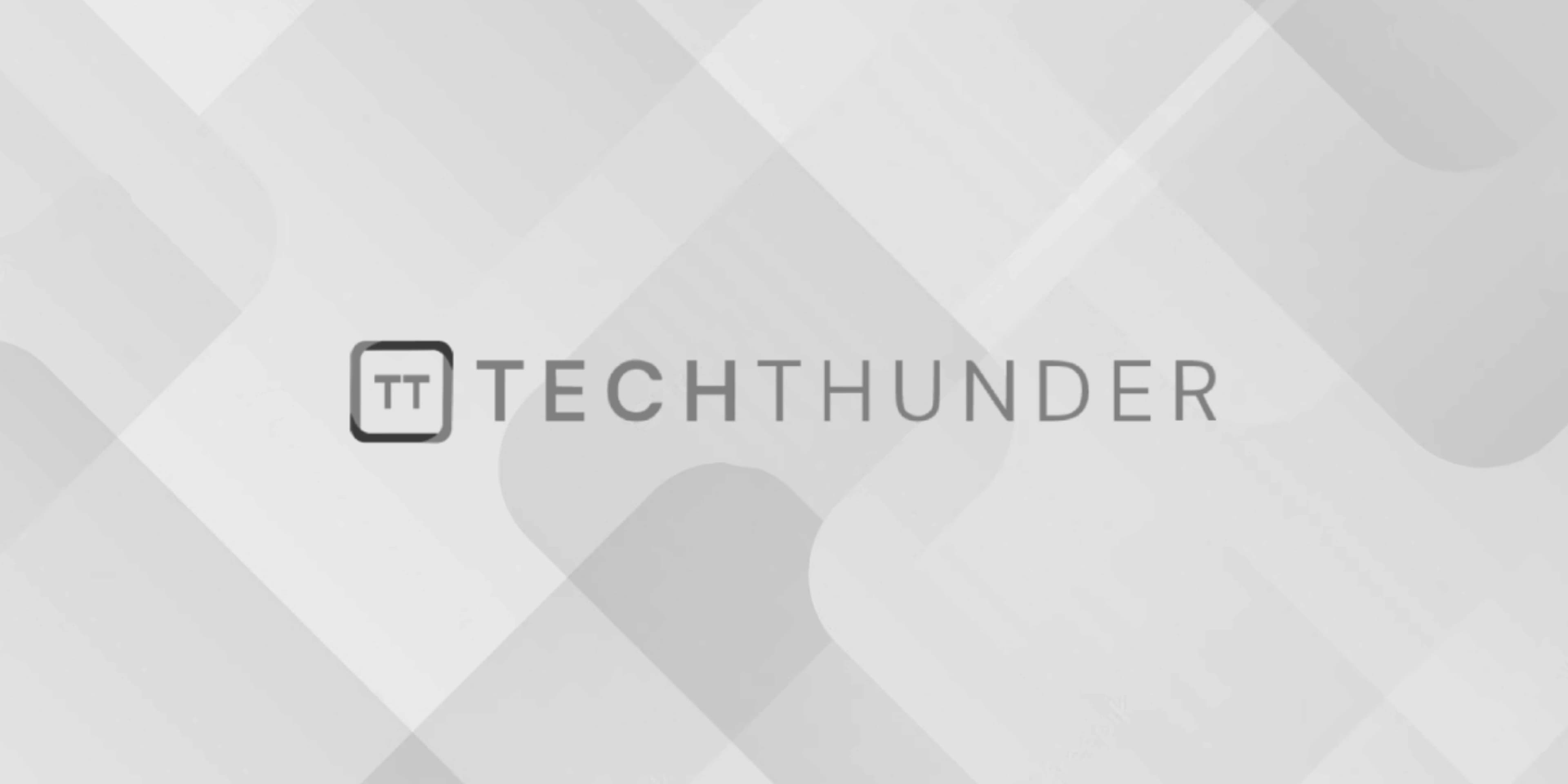
JavaFX
JavaFX is a modern, rich-client platform for building and deploying interactive, expressive, and high-performance desktop applications. It provides a set of APIs for creating a wide range of desktop and mobile applications, including multimedia, 2D and 3D graphics, web views, and more. JavaFX is a replacement for the older Swing toolkit and offers features that make it a compelling choice for developing cross-platform desktop applications.
Here are some key features and concepts of JavaFX:
- Rich User Interfaces: JavaFX enables the creation of visually appealing and interactive user interfaces with support for styles, themes, animations, and multimedia elements.
- FXML: FXML is a markup language for designing user interfaces in a declarative manner. It allows developers and designers to work on the user interface separately. FXML files can be loaded and processed at runtime.
- Scene Graph: JavaFX applications are built around a hierarchical scene graph. The scene graph represents the structure of the user interface, and it is rendered by the JavaFX runtime. Each node in the scene graph is a graphical element or a container.
- CSS Styling: JavaFX supports Cascading Style Sheets (CSS) for styling the user interface components. You can use CSS to define the appearance and layout of your application.
- 2D and 3D Graphics: JavaFX provides a powerful graphics API for creating 2D and 3D graphics. You can draw shapes, images, and complex scenes using JavaFX’s graphics capabilities.
- Event Handling: JavaFX applications use an event-driven model for handling user interactions, similar to Swing. Event listeners can be added to nodes to respond to user actions.
- Media and Multimedia: JavaFX has built-in support for media playback, including audio and video. You can play and control media content within your applications.
- WebView: JavaFX includes a WebView component, which is a lightweight, fully functional web browser component. It allows you to display web content within your application.
- Layout Managers: JavaFX provides various layout managers for arranging nodes in a scene, including
VBox
,HBox
,BorderPane
, and others. - Concurrency: JavaFX includes support for concurrent programming and multithreading, making it easier to create responsive applications.
- Integration: JavaFX can be integrated with other Java technologies, such as Java 2D, Swing, and third-party libraries.
- Cross-Platform: JavaFX applications are cross-platform and can be run on Windows, macOS, and Linux.
- Deployment: JavaFX applications can be deployed as standalone executables, JAR files, or web applets.
Here’s a simple example of creating a JavaFX application with a basic user interface:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class JavaFXHelloWorld extends Application {
@Override
public void start(Stage primaryStage) {
Label helloLabel = new Label("Hello, JavaFX!");
StackPane root = new StackPane(helloLabel);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("JavaFX Hello World");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
To run a JavaFX application, you typically extend the Application
class and implement the start
method. JavaFX applications begin by launching a JavaFX application thread, and you can build your user interface within the start
method.
JavaFX offers a modern and powerful toolkit for developing rich desktop applications. It is a versatile choice for creating cross-platform applications with compelling user interfaces. If you are considering Java for desktop development, JavaFX is worth exploring.