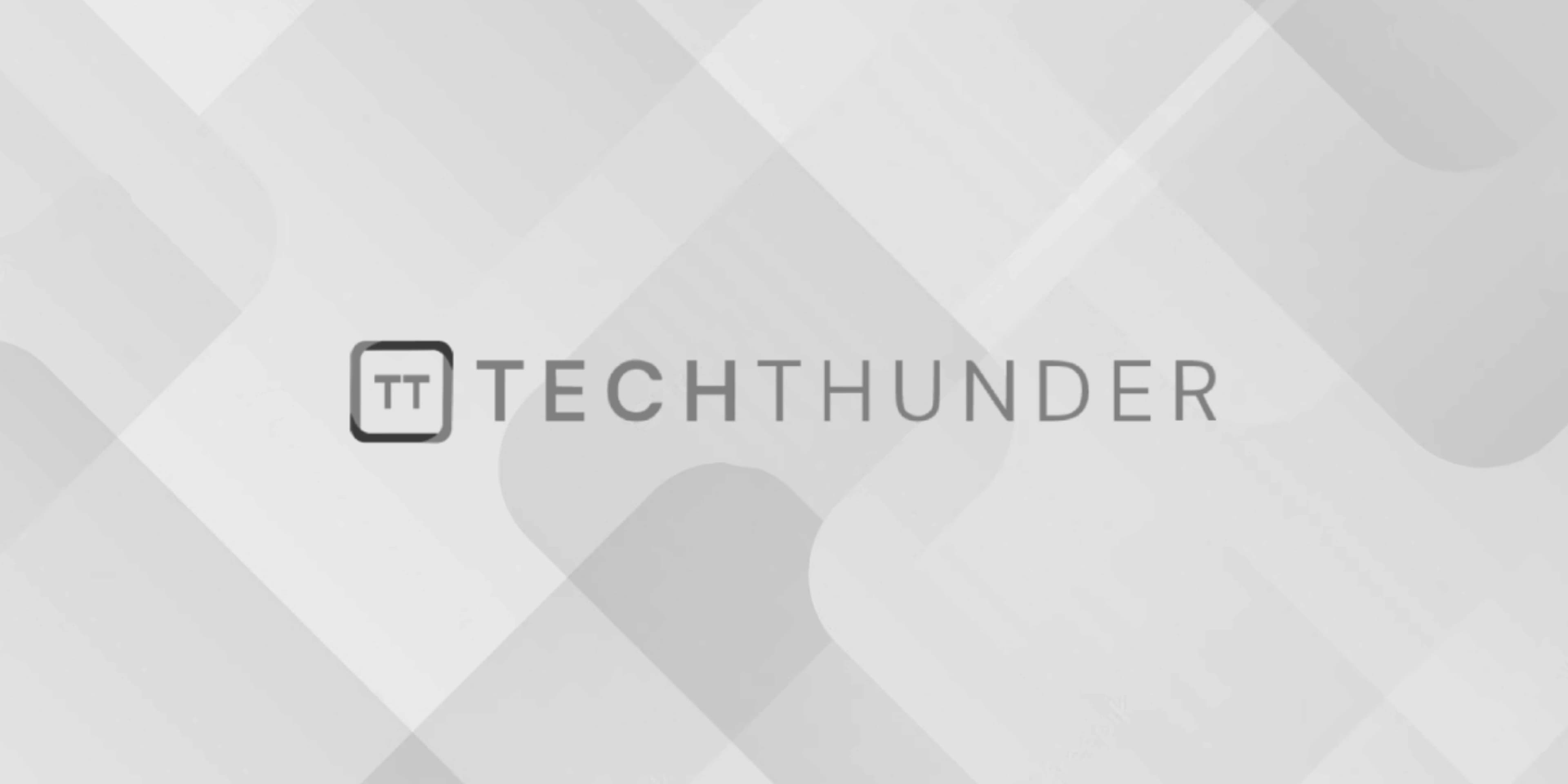
Java I/O
Java Input/Output (I/O) refers to the process of reading data from and writing data to external sources such as files, streams, networks, and other devices. The Java I/O system provides classes and APIs to handle various I/O operations efficiently and effectively. Java’s I/O operations are categorized into two main types: byte-based I/O and character-based I/O.
Byte-Based I/O:
Byte-based I/O is used to handle raw binary data. It’s suitable for reading and writing non-text files, images, audio, video, and other binary data.
InputStream
andOutputStream
: The base classes for byte-based input and output operations. Common subclasses includeFileInputStream
,FileOutputStream
, andByteArrayInputStream
.DataInputStream
andDataOutputStream
: Wrappers aroundInputStream
andOutputStream
that allow you to read and write primitive data types (e.g.,int
,double
) in binary format.BufferedInputStream
andBufferedOutputStream
: Buffered versions of byte-based I/O classes, providing improved performance by reducing the number of actual I/O operations.
Character-Based I/O:
Character-based I/O is used for reading and writing text data, and it deals with characters rather than raw binary data.
Reader
andWriter
: The base classes for character-based input and output operations. Subclasses includeFileReader
,FileWriter
, andStringReader
.BufferedReader
andBufferedWriter
: Buffered versions of character-based I/O classes, offering improved performance by reducing the number of I/O operations.InputStreamReader
andOutputStreamWriter
: Classes that bridge byte-based and character-based I/O. They allow you to convert between byte streams and character streams using specified character encodings.
File I/O:
Java provides classes to read from and write to files.
import java.io.*;
public class FileIOExample {
public static void main(String[] args) {
try {
// Writing to a file
FileWriter writer = new FileWriter("output.txt");
writer.write("Hello, Java I/O!");
writer.close();
// Reading from a file
FileReader reader = new FileReader("output.txt");
int character;
while ((character = reader.read()) != -1) {
System.out.print((char) character);
}
reader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
Standard I/O:
Java also provides access to standard input, output, and error streams using System.in
, System.out
, and System.err
.
import java.util.Scanner;
public class StandardIOExample {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "!");
scanner.close();
}
}
The Java I/O system is powerful and versatile, allowing you to interact with various types of data sources and destinations. It’s important to handle exceptions properly when working with I/O operations and to close resources to prevent resource leaks.