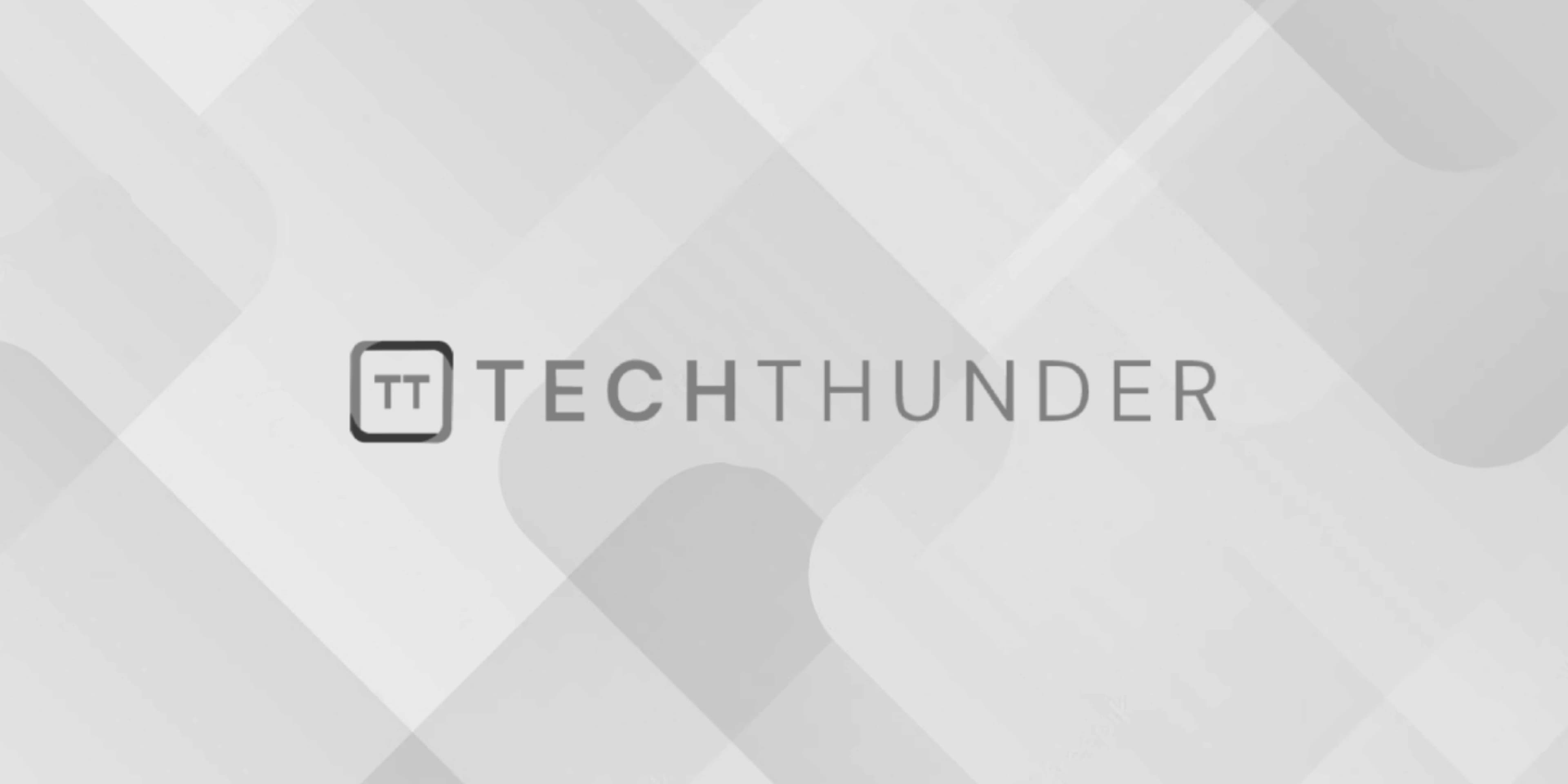
217 views
Java Exception Handling
Exception handling is an essential part of Java programming, enabling you to manage and respond to unexpected or exceptional situations that can occur during program execution. Java provides a robust exception-handling mechanism through the use of the try
, catch
, finally
, and throw
statements.
Here’s an overview of how exception handling works in Java:
- Types of Exceptions:
- Checked Exceptions: These are exceptions that must be explicitly handled using try-catch blocks or declared in the method signature using the
throws
keyword. Common examples includeIOException
andSQLException
. - Unchecked Exceptions (Runtime Exceptions): These are exceptions that don’t need to be declared explicitly. They can be caught or left unhandled. Common examples include
NullPointerException
andArrayIndexOutOfBoundsException
. - Errors: These represent exceptional situations that typically cannot be recovered, and it’s not appropriate to handle them programmatically. Common examples include
OutOfMemoryError
andStackOverflowError
.
try-catch
Blocks:
- The
try
block is used to enclose the code that might throw an exception. - The
catch
block follows thetry
block and contains code to handle the exception. You can have multiplecatch
blocks to handle different types of exceptions. - Here’s a simple example:
try { // Code that may throw an exception int result = 10 / 0; } catch (ArithmeticException e) { // Handle the exception System.out.println("An error occurred: " + e.getMessage()); }
finally
Block:
- The
finally
block, if used, is executed regardless of whether an exception occurred or not. It’s commonly used for cleanup operations, such as closing resources (e.g., files or database connections). - Example:
FileInputStream file = null; try { file = new FileInputStream("example.txt"); // Read from the file } catch (IOException e) { // Handle the exception } finally { // Close the file, even if an exception occurred if (file != null) { try { file.close(); } catch (IOException e) { // Handle the closing exception } } }
throw
Statement:
- The
throw
statement is used to explicitly throw an exception. You can use it to create custom exceptions or to rethrow caught exceptions. - Example:
if (condition) { throw new CustomException("This is a custom exception message"); }
- Custom Exceptions:
- You can create your own custom exceptions by extending the
Exception
orRuntimeException
classes. Custom exceptions can provide more meaningful information about errors specific to your application. - Example of creating a custom exception:
class CustomException extends Exception { public CustomException(String message) { super(message); } }
try-with-resources
:
- Java introduced the try-with-resources statement, available since Java 7, to simplify resource management, such as closing files, sockets, or database connections. It automatically closes resources when they are no longer needed.
- Example:
try (FileInputStream file = new FileInputStream("example.txt")) { // Use the file } catch (IOException e) { // Handle the exception }
Java’s exception handling mechanism allows you to gracefully handle errors and exceptional situations in your code, improving the robustness and reliability of your applications. Proper exception handling is a critical aspect of Java programming, and it ensures that your application can recover gracefully from unexpected issues.