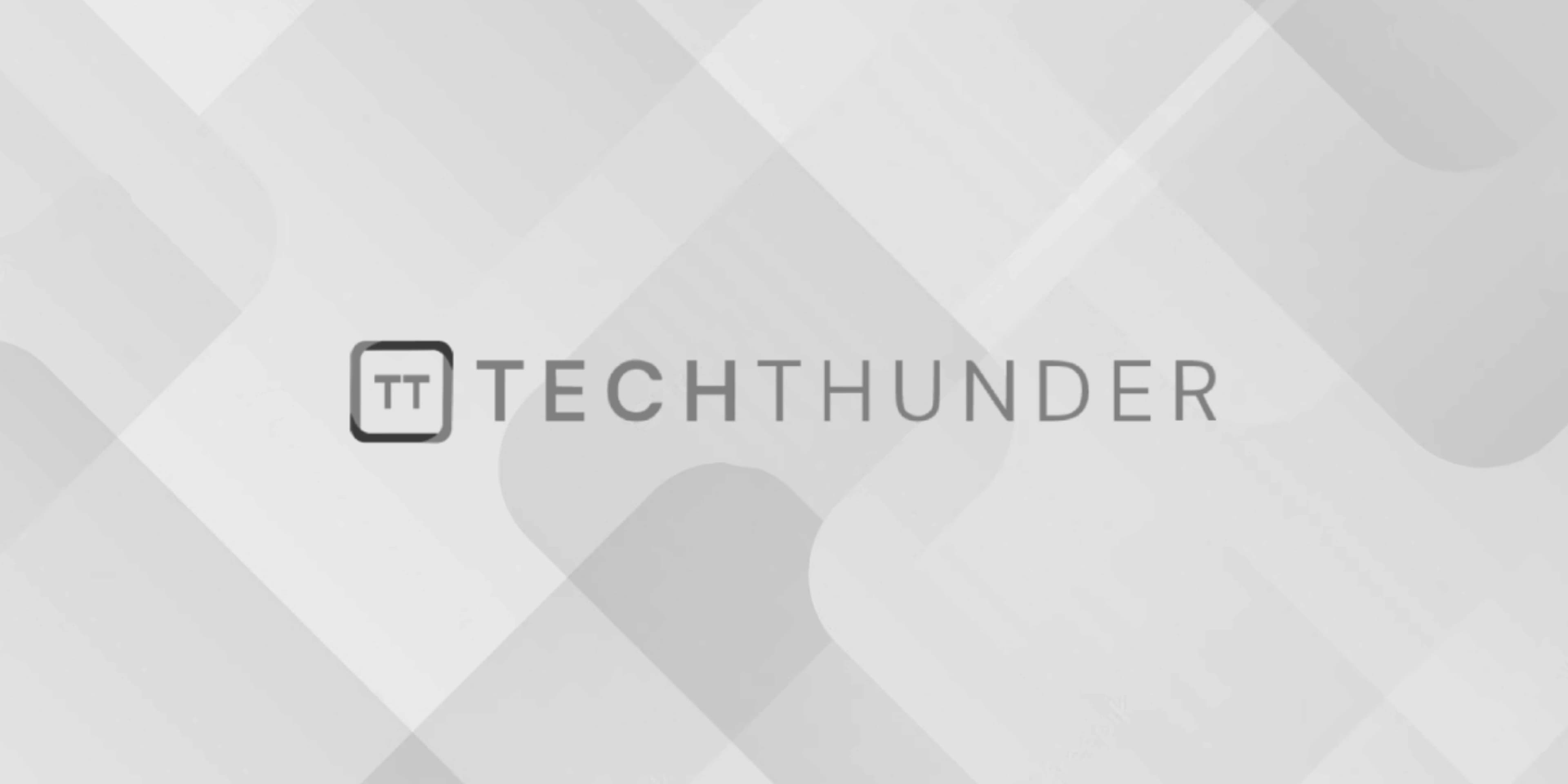
Java instanceof operator
The Java instanceof
operator is used to test whether an object is an instance of a particular class, interface, or a subclass thereof. It is a binary operator that returns a boolean value, indicating whether the left-hand operand is an instance of the right-hand operand.
The syntax of the instanceof
operator is as follows:
objectReference instanceof Type
objectReference
is the reference to the object you want to test.Type
is the class or interface you want to check for.
The instanceof
operator can be used in conditional statements and expressions to determine whether an object is of a particular type before performing certain operations. It helps you avoid type-casting errors and ensures that operations are only performed when they are valid for the given object type.
Here’s an example of how the instanceof
operator can be used:
class Animal {
// Common properties and methods for animals
}
class Dog extends Animal {
void bark() {
System.out.println("Woof!");
}
}
class Cat extends Animal {
void meow() {
System.out.println("Meow!");
}
}
public class InstanceOfExample {
public static void main(String[] args) {
Animal myPet = new Dog();
if (myPet instanceof Dog) {
Dog dog = (Dog) myPet; // Type casting to access specific methods
dog.bark();
} else if (myPet instanceof Cat) {
Cat cat = (Cat) myPet;
cat.meow();
} else if (myPet instanceof Animal) {
System.out.println("It's a generic animal.");
} else {
System.out.println("Unknown type");
}
}
}
The myPet
is an instance of the Dog
class. We use the instanceof
operator to check whether it’s a Dog
, a Cat
, or a generic Animal
. Depending on the result of the check, we can safely perform actions specific to that type, like calling bark()
for a Dog
.
It’s important to use the instanceof
operator along with appropriate type casting (if needed) to ensure type safety and prevent ClassCastExceptions. Using instanceof
allows you to make informed decisions about the object’s type before interacting with it.