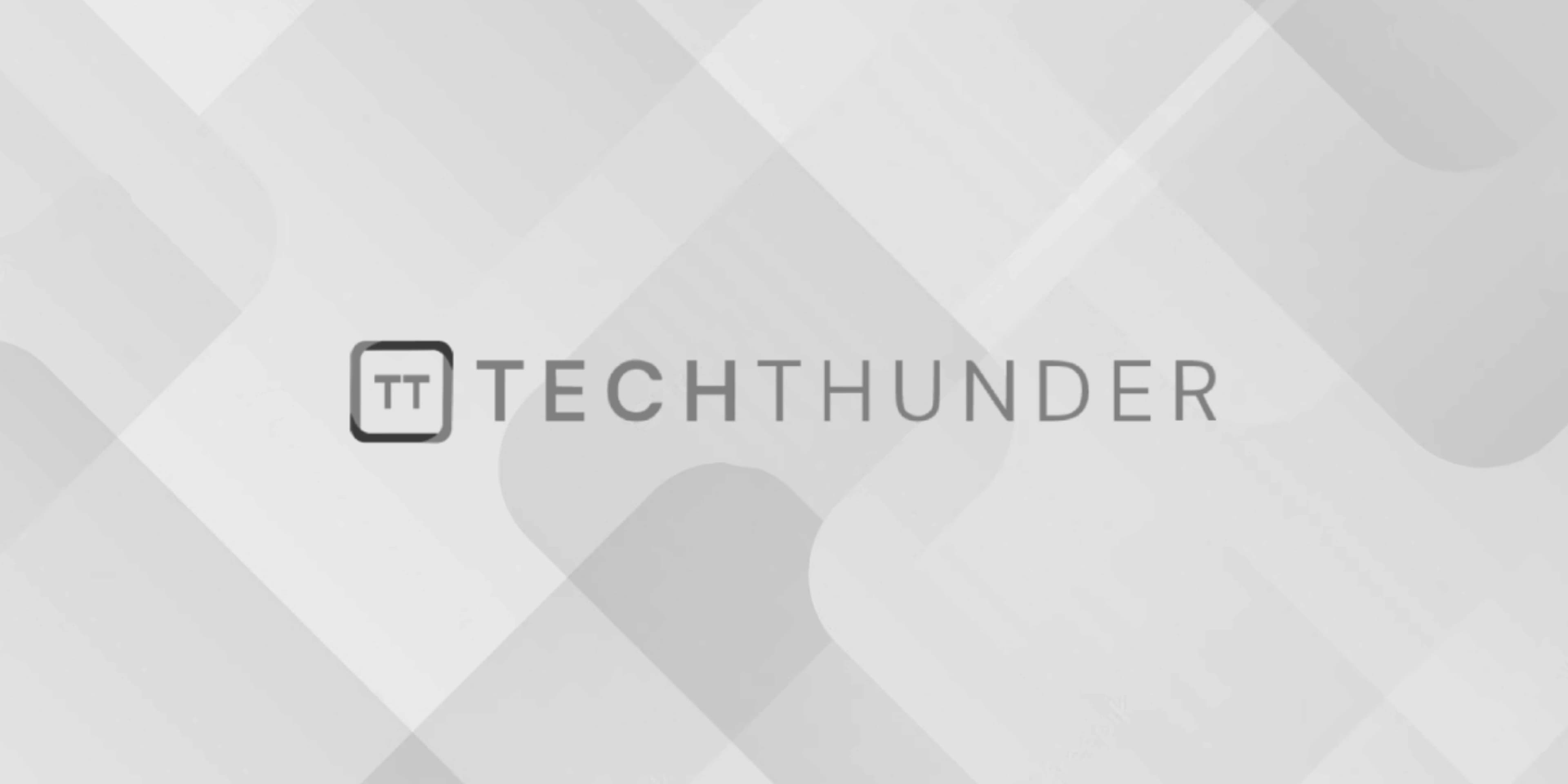
Java Object vs Class
The Java has both “objects” and “classes” are fundamental concepts in object-oriented programming, but they serve different purposes and have distinct roles.
- Class:
- A class in Java is a blueprint or a template for creating objects.
- It defines the structure and behavior of objects of a particular type.
- A class can have fields (variables) and methods (functions) that describe the properties and actions of objects of that class.
- Classes are used to create objects, and they encapsulate the state and behavior of those objects.
- Classes do not exist at runtime but are used to create objects during runtime. Example of a simple Java class:
public class Person {
String name;
int age;
public void speak() {
System.out.println("Hello, my name is " + name + " and I am " + age + " years old.");
}
}
- Object:
- An object is an instance of a class.
- It is created from a class, and it represents a real-world entity that can be manipulated and interacted with in your program.
- Objects have their own unique states (values of fields) and can execute methods defined in their class.
- You can create multiple objects from the same class, each with its own state and behavior. Example of creating and using objects:
public class ObjectExample {
public static void main(String[] args) {
// Create objects of the 'Person' class
Person person1 = new Person();
person1.name = "Alice";
person1.age = 30;
Person person2 = new Person();
person2.name = "Bob";
person2.age = 25;
// Call methods on objects
person1.speak();
person2.speak();
}
}
In the above code, Person
is a class that defines the structure and behavior of a person, and person1
and person2
are objects created from that class. Each object has its own name
and age
fields, and they can execute the speak()
method defined in the Person
class.
The “class” is a blueprint for creating objects, defining their structure and behavior, while an “object” is an instance of a class, representing a specific entity with its own state and behavior. Objects are created from classes and are the primary entities you work with when writing Java programs. Classes are used to define the types of objects you need in your program.