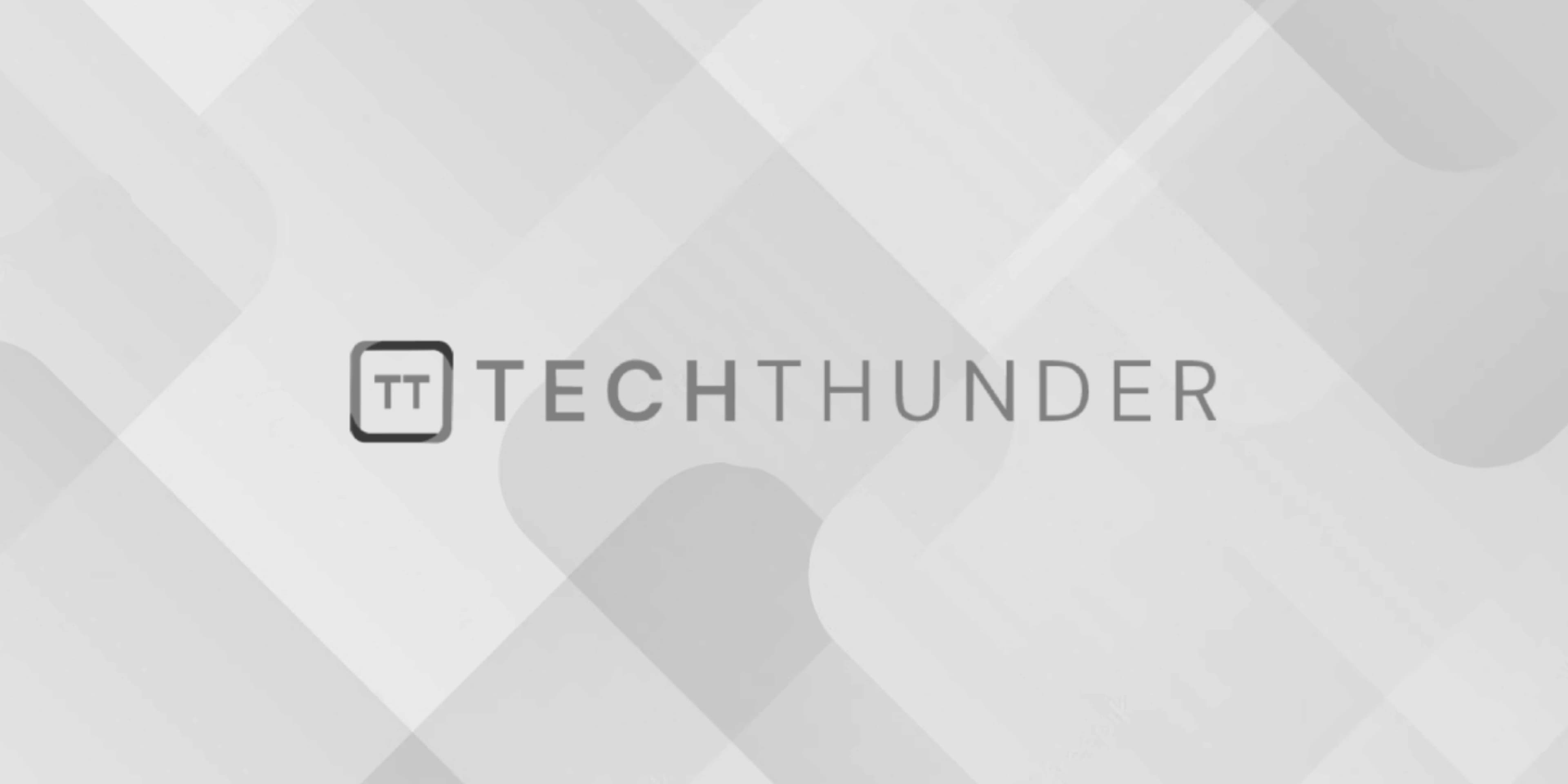
Java Instance Initializer block
The Java instance initializer block is a block of code that is defined within a class but outside of any method or constructor. It is used to initialize instance variables or perform other tasks that need to be executed when an instance of the class is created. Instance initializer blocks are executed when an object is created before any constructors are called.
Here’s the basic syntax for an instance initializer block:
class MyClass {
// Instance variables
int x;
int y;
// Instance initializer block
{
// Code to initialize instance variables or perform other tasks
x = 10;
y = 20;
// Additional code here
}
// Constructors and other methods can follow
}
Key points about instance initializer blocks:
- Execution Order: Instance initializer blocks are executed in the order they appear in the class, just before the constructor of the class is invoked. If there are multiple initializer blocks in a class, they are executed in the order of declaration.
- Initialization: Instance initializer blocks are often used to initialize instance variables or perform complex initialization tasks that can’t be accomplished in a single line within a constructor.
- Common Use Cases:
- Performing complex initialization that involves multiple statements.
- Handling exceptions that might occur during object creation.
- Initializing non-final instance variables that are not known until runtime.
- Benefits:
- Instance initializer blocks help centralize initialization logic for instance variables, making code more organized and easier to maintain.
- They are especially useful when multiple constructors need to share the same initialization logic.
Here’s an example of an instance initializer block in a class:
class Circle {
double radius;
double area;
// Instance initializer block
{
// Initialize area based on the radius
area = Math.PI * radius * radius;
}
// Constructors and other methods can follow
public Circle(double radius) {
this.radius = radius;
}
public void displayArea() {
System.out.println("Area of the circle: " + area);
}
}
public class Main {
public static void main(String[] args) {
Circle circle = new Circle(5.0);
circle.displayArea(); // Output: Area of the circle: 78.53981633974483
}
}
In this example, the instance initializer block is used to calculate the area of a circle based on its radius. When an instance of the Circle
class is created, the initializer block is executed, and the area
variable is initialized before the displayArea
method is called.
Instance initializer blocks are a useful tool for encapsulating complex initialization logic within a class, improving code readability and maintainability.