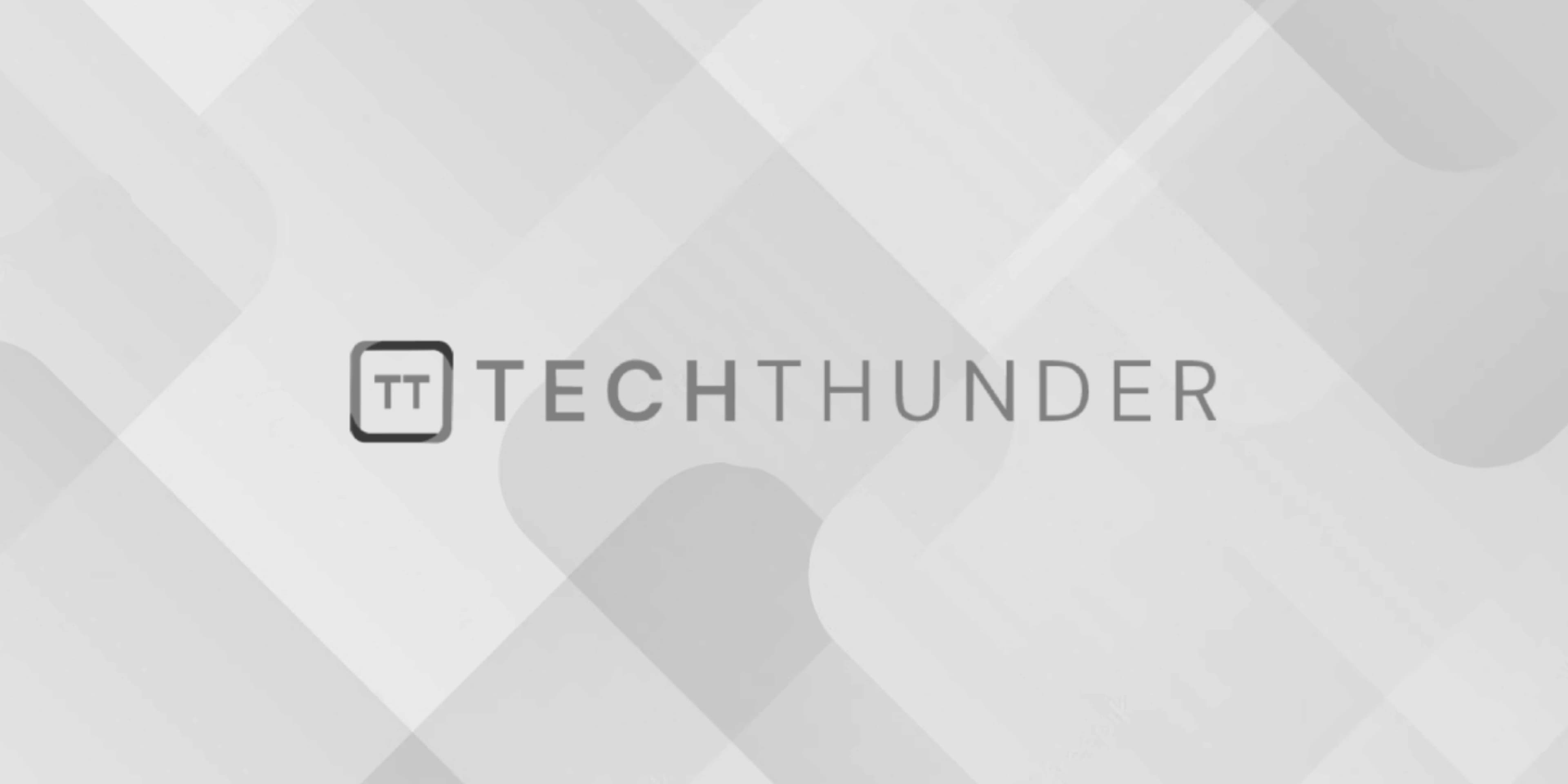
Java Wrapper Class
The Java wrapper classes are a set of classes provided by the Java standard library to represent primitive data types as objects. They allow you to work with primitive data types in situations where objects are required, such as when using collections, generics, or when you need to call methods that expect objects. The Java wrapper classes are part of the java.lang
package, and there is a one-to-one correspondence between primitive types and their corresponding wrapper classes. Here are the Java wrapper classes for common primitive types:
Integer
– Wrapper forint
Long
– Wrapper forlong
Double
– Wrapper fordouble
Float
– Wrapper forfloat
Short
– Wrapper forshort
Byte
– Wrapper forbyte
Character
– Wrapper forchar
Boolean
– Wrapper forboolean
These wrapper classes provide useful methods for converting, parsing, and manipulating primitive data types, and they allow you to work with them in an object-oriented way. Here’s an example of using wrapper classes:
Integer intObj = new Integer(42); // Wrapping an int
int intValue = intObj.intValue(); // Unwrapping to get the int value
String intStr = "123";
int parsedInt = Integer.parseInt(intStr); // Parsing a string to an int using the wrapper class
List<Integer> integerList = new ArrayList<>();
integerList.add(1); // Autoboxing - primitive int is automatically wrapped in Integer
int sum = 0;
for (Integer num : integerList) {
sum += num; // Unboxing - Integer objects are automatically unwrapped to int
}
System.out.println("Sum: " + sum);
In the example above, we use the Integer
wrapper class to wrap an int
, unwrap it to get the primitive value, parse a string to an integer, and demonstrate autoboxing and unboxing within a list.
Autoboxing and unboxing are features in Java that allow automatic conversion between primitive types and their corresponding wrapper classes. These features make it easier to work with both primitive types and objects when needed.
The use of wrapper classes is particularly common when working with collections such as List
, Set
, and Map
, which store objects, not primitive types. Additionally, they are useful when you need to represent null
values, as the wrapper classes can be null
, unlike primitive types.