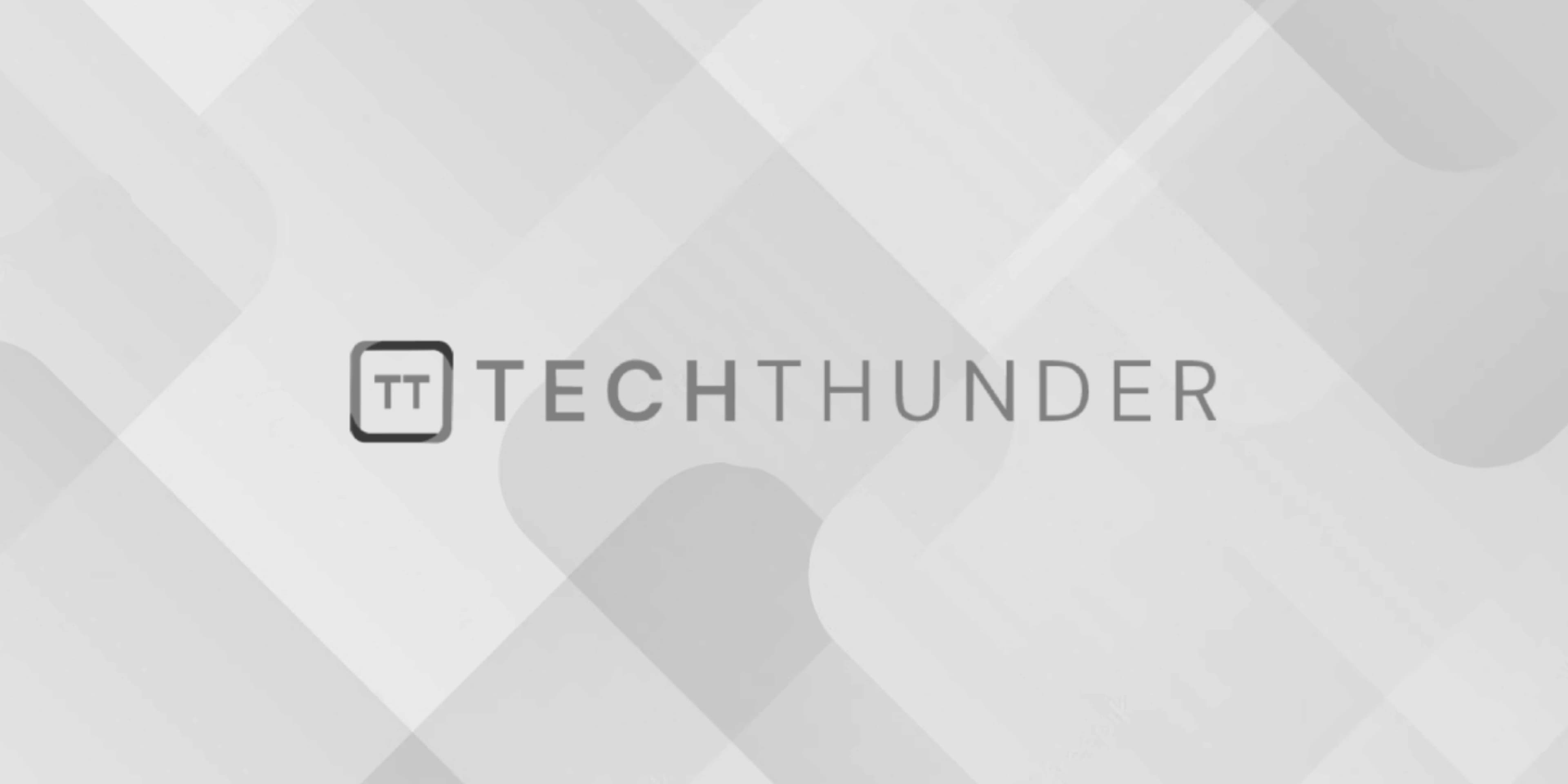
215 views
Java Unicode System
The Java uses Unicode to represent characters in its character set. Unicode is a standardized character encoding that assigns a unique code point (an integer value) to each character, symbol, or ideograph from almost every writing system in the world. Java uses Unicode to ensure that text can be represented and manipulated in a consistent and international manner.
Here are some key points related to Unicode and its usage in Java:
- Character Encoding: Unicode provides a standardized way to encode characters using unique numeric values (code points). Java uses the UTF-16 (16-bit Unicode Transformation Format) character encoding, which means that each character in a Java string is represented as a 16-bit code unit.
- Internationalization: Unicode support in Java is a fundamental aspect of internationalization (i18n). It allows Java applications to work with text and data in various languages and scripts, ensuring compatibility and correctness across different locales.
- Characters and Strings: In Java, characters are represented using the
char
data type, which is a 16-bit data type capable of representing Unicode characters. Java strings are sequences ofchar
values. - Unicode Escape Sequences: Unicode characters can be represented in Java source code using Unicode escape sequences. For example,
\u0041
represents the Unicode character ‘A’. - Character Literals: Java allows you to use Unicode characters directly in character literals. For example,
char ch = 'Ω';
assigns the Greek letter Omega (Ω) to thech
variable. - String Comparison: When comparing strings in Java, be aware of potential issues related to Unicode normalization and case sensitivity, especially when working with characters from different scripts.
- Normalization: Java provides classes like
java.text.Normalizer
to handle text normalization, which ensures that equivalent Unicode sequences are considered equal. - Character Encoding and I/O: When reading or writing text from or to external sources (e.g., files, network), you need to consider character encoding. Java provides classes like
java.io.Reader
andjava.io.Writer
for reading and writing text in specific character encodings.
Here’s a simple example that demonstrates the use of Unicode characters in Java:
public class UnicodeExample {
public static void main(String[] args) {
char omega = 'Ω'; // Greek letter Omega
char smiley = '\u263A'; // Unicode code point for a smiley face
System.out.println("Greek Omega: " + omega);
System.out.println("Smiley Face: " + smiley);
}
}
We assign and display characters ‘Ω’ (Greek Omega) and a smiley face using Unicode literals. Java’s support for Unicode makes it a powerful tool for working with text in a global and multilingual context.