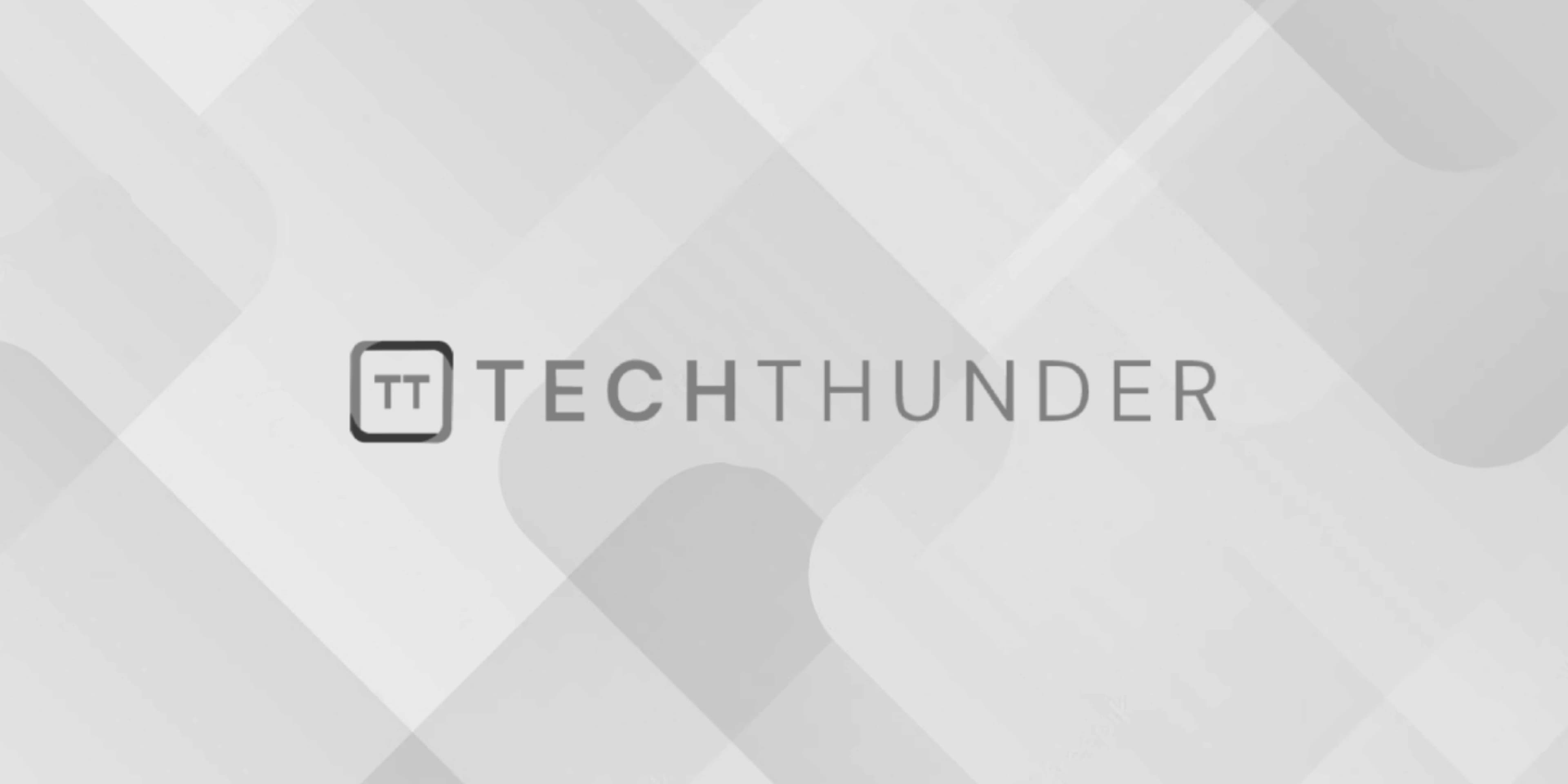
Java Command Line Arg
The Java can pass command-line arguments to a Java program when you run it from the command line. Command-line arguments are values that are supplied as parameters to your program when you execute it. These values can be used to customize the behavior of your program at runtime. Here’s how you can work with command-line arguments in Java:
- Accessing Command-Line Arguments: Command-line arguments are passed to the
main
method of your Java program as an array of strings. You can access these arguments as follows:
public class CommandLineArguments {
public static void main(String[] args) {
// args is an array of command-line arguments
// args[0] is the first argument, args[1] is the second, and so on
if (args.length > 0) {
System.out.println("The first argument is: " + args[0]);
} else {
System.out.println("No arguments provided.");
}
}
}
- Running a Java Program with Command-Line Arguments: To run a Java program with command-line arguments, you use the
java
command followed by the name of the Java class and the arguments you want to pass. For example:
java CommandLineArguments arg1 arg2 arg3
In this example, “arg1,” “arg2,” and “arg3” are the command-line arguments passed to the CommandLineArguments
program.
- Number of Command-Line Arguments: You can determine the number of command-line arguments passed by accessing the
length
property of theargs
array. For example,args.length
will give you the number of arguments passed to your program. - Converting Command-Line Arguments: The
args
array contains strings, so if you need to use the arguments as other data types (e.g., integers, doubles), you will need to convert them using appropriate parsing methods (e.g.,Integer.parseInt()
for integers).
Here’s an example of running a Java program with command-line arguments and converting one of the arguments to an integer:
public class CommandLineArguments {
public static void main(String[] args) {
if (args.length >= 2) {
String name = args[0];
int age = Integer.parseInt(args[1]);
System.out.println("Name: " + name);
System.out.println("Age: " + age);
} else {
System.out.println("Please provide a name and age as command-line arguments.");
}
}
}
To run this program, you might use the following command:
java CommandLineArguments Alice 30
In this example, “Alice” and “30” are the command-line arguments. The program extracts these arguments, converts “30” to an integer, and displays the values.
Command-line arguments are a useful way to customize and parameterize your Java programs, making them more versatile and adaptable to different use cases.