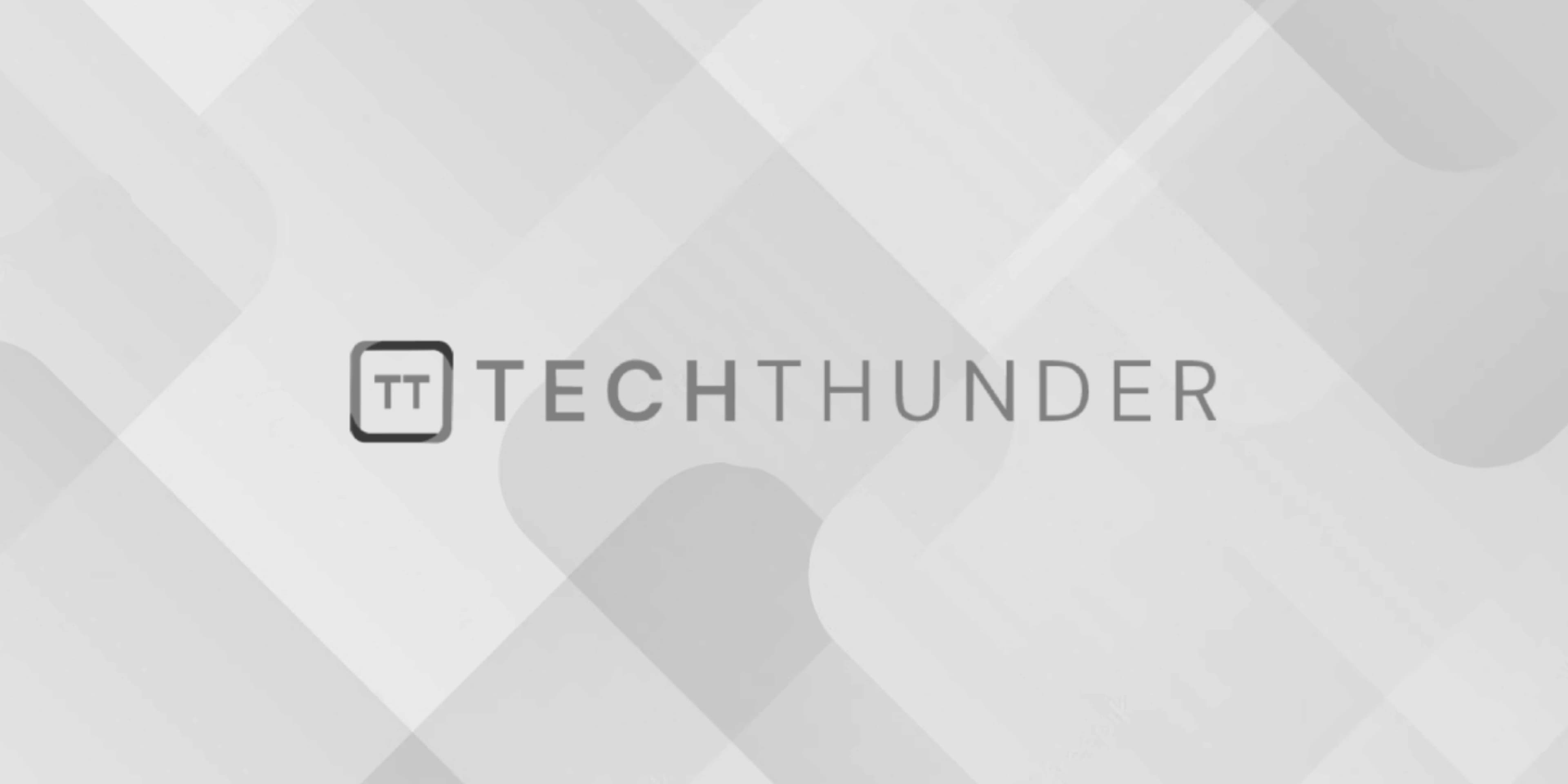
258 views
Java Access Modifiers
The Java access modifiers are keywords that control the visibility and accessibility of classes, fields, methods, and other members within your code. They play a crucial role in maintaining encapsulation, controlling who can access or modify elements in your code, and ensuring data privacy and security. Java provides four main access modifiers:
public
:
- Members (classes, fields, methods, etc.) marked as
public
are accessible from any class or package. - There are no access restrictions, and public members are part of the API for the class.
- For example, a
public
method in a class can be called from any other class.
public class MyClass {
public int myPublicField;
public void myPublicMethod() {
// Code here
}
}
protected
:
- Members marked as
protected
are accessible within the same package and by subclasses, even if they are in different packages. - This modifier is often used to implement inheritance and provide a degree of encapsulation.
- For example, a
protected
field is accessible in subclasses but not by classes in unrelated packages.
class MyBaseClass {
protected int myProtectedField;
}
public class MySubClass extends MyBaseClass {
void accessProtectedField() {
int x = myProtectedField; // Access to the protected field
}
}
default
(Package-Private):
- When no access modifier is specified (i.e., no
public
,protected
, orprivate
), the default access modifier is used. - Members marked as default are accessible only within the same package. They are not accessible from outside the package.
class MyClass {
int myDefaultField; // Package-private access
}
private
:
- Members marked as
private
are the most restrictive. They are only accessible within the same class. - This provides the highest level of encapsulation and data hiding.
- Typically used to hide the internal implementation details of a class.
public class MyClass {
private int myPrivateField;
private void myPrivateMethod() {
// Code here
}
}
Here’s a summary of the access modifiers and their visibility:
public | Accessible from anywhere, including other packages. |
protected | Accessible within the same package and by subclasses. |
default | Accessible only within the same package. |
private | Accessible only within the same class. |
It’s important to use access modifiers appropriately to ensure that your code is secure, maintainable, and follows the principles of encapsulation. Good use of access modifiers helps create clear, well-structured code and promotes code reusability.