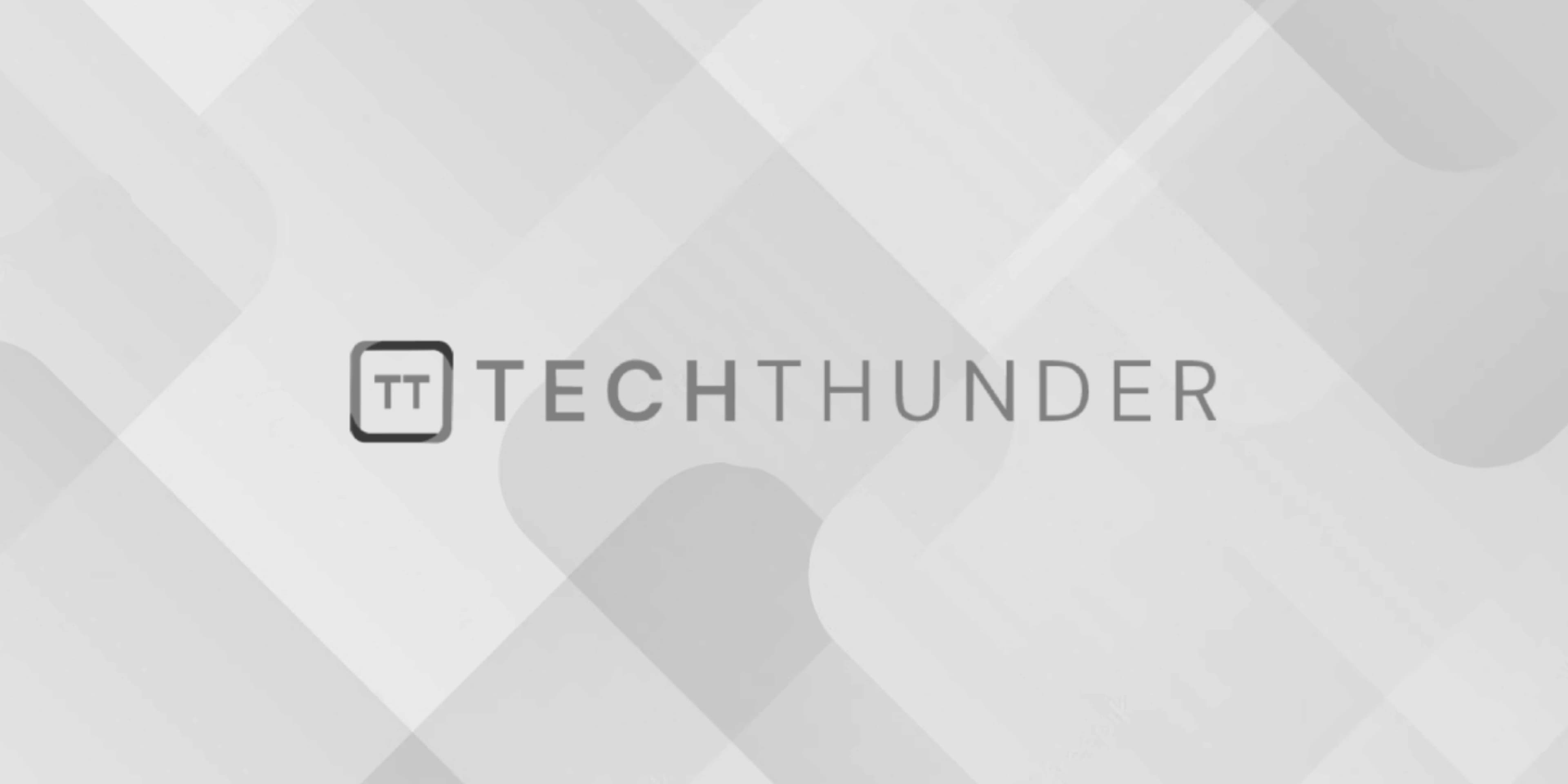
Java Encapsulation
Encapsulation is one of the four fundamental concepts of object-oriented programming (OOP), and it’s a key principle in Java. Encapsulation is the practice of hiding the internal details and state of an object while providing a well-defined public interface for interacting with that object. In Java, encapsulation is achieved through the use of access modifiers (e.g., private
, protected
, public
, and default) and getter and setter methods. Here are the main aspects of encapsulation in Java:
- Private Data Members:
- In encapsulation, class data members (fields) are typically declared as
private
. This means that the internal state of the class is not directly accessible from outside the class.
public class Person {
private String name;
private int age;
}
- Public Accessor Methods (Getters):
- To provide controlled access to the private data members, public methods, often called “getters,” are used to retrieve the values. Getters are used to read the state of an object.
public String getName() {
return name;
}
public int getAge() {
return age;
}
- Public Mutator Methods (Setters):
- To modify the state of the object in a controlled way, public methods, often called “setters,” are used to update the values. Setters are used to write or change the state of an object.
public void setName(String name) {
this.name = name;
}
public void setAge(int age) {
if (age >= 0) {
this.age = age;
}
}
- Data Validation:
- Encapsulation allows you to perform data validation and enforce rules before modifying the internal state of an object. For example, you can ensure that an age is non-negative before setting it.
public void setAge(int age) {
if (age >= 0) {
this.age = age;
} else {
// Handle invalid input, throw an exception, or log an error.
}
}
- Controlled Access:
- Encapsulation provides control over who can access and modify the internal state of an object. By making fields
private
and using getters and setters, you can ensure that data is accessed and modified in a predictable and controlled manner.
- Information Hiding:
- Encapsulation hides the implementation details and complexity of an object, making it easier to understand and use.
- Flexibility and Maintainability:
- Encapsulation allows you to change the internal implementation of a class without affecting the external code that uses the class. This promotes maintainability and reduces the risk of introducing bugs when modifying a class.
The encapsulation in Java is a fundamental concept that promotes data hiding, controlled access to object state, and the separation of implementation details from the public interface. It is a key aspect of writing clean, maintainable, and secure Java code.