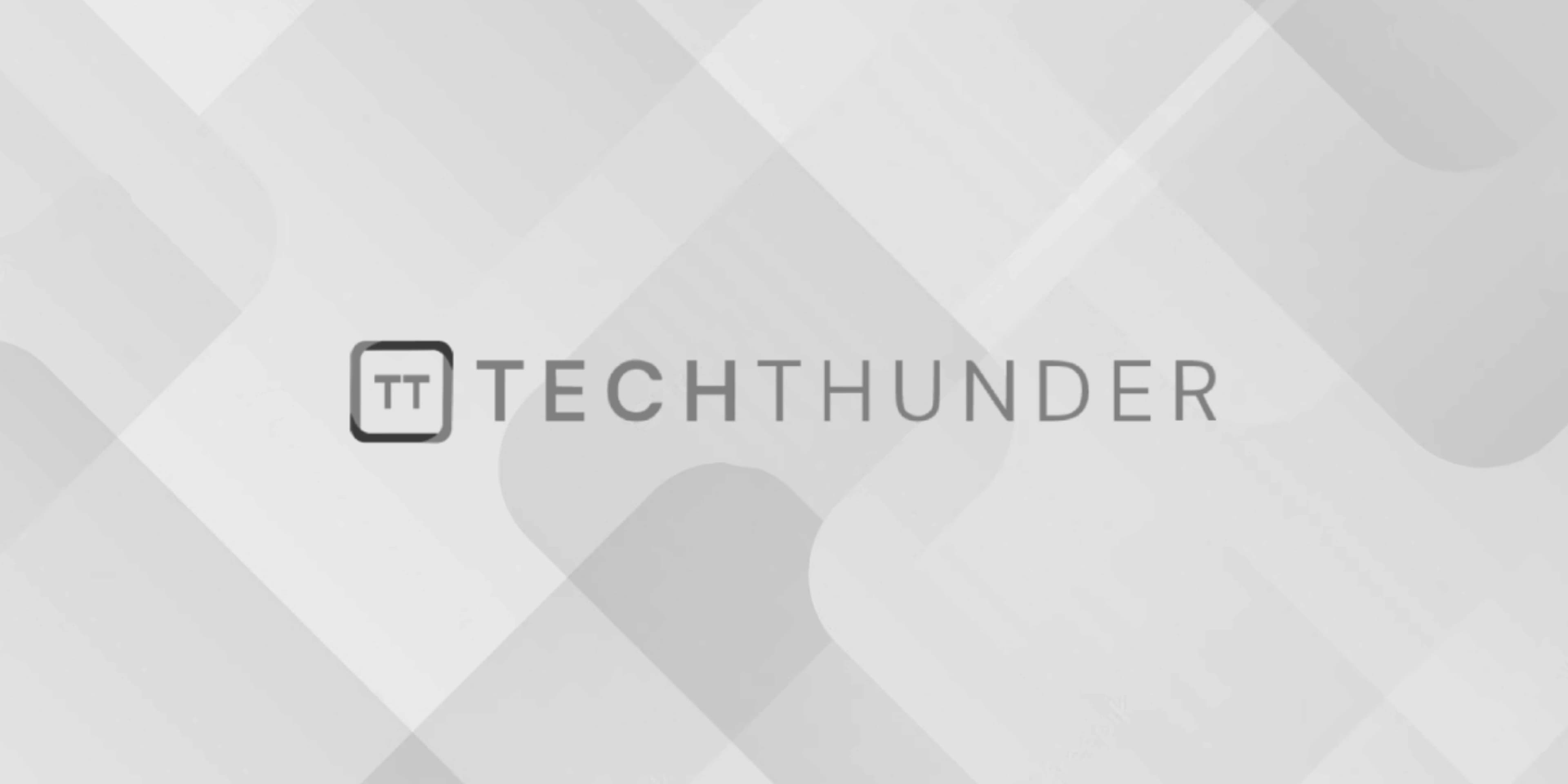
304 views
Java Inheritance
Inheritance is a fundamental concept in object-oriented programming (OOP), and it plays a crucial role in Java. Inheritance allows you to create a new class by inheriting the attributes and behaviors (fields and methods) of an existing class. The existing class is referred to as the superclass or base class, and the new class is called the subclass or derived class. Here’s an overview of inheritance in Java:
- Defining a Superclass:
- A superclass is defined as a regular class in Java, with attributes and methods.
- The superclass serves as a template for the attributes and methods that will be inherited by subclasses.
public class Animal {
String name;
public void eat() {
System.out.println(name + " is eating.");
}
}
- Defining a Subclass:
- A subclass is defined using the
extends
keyword, followed by the name of the superclass. - The subclass inherits the attributes and methods of the superclass and can add its own attributes and methods.
public class Dog extends Animal {
public void bark() {
System.out.println(name + " is barking.");
}
}
- Inherited Members:
- Subclasses inherit all
public
andprotected
attributes and methods of the superclass. - The
private
members of the superclass are not inherited by the subclass. - The subclass can access inherited members as if they were defined in the subclass itself.
- Method Overriding:
- Subclasses can provide their own implementations of methods inherited from the superclass. This is known as method overriding.
- To override a method, the subclass’s method should have the same method signature (name, return type, and parameters).
public class Dog extends Animal {
public void eat() {
System.out.println(name + " the dog is eating.");
}
}
- Access Modifiers:
- In Java, access modifiers control the visibility of members. Subclasses can access
public
andprotected
members of the superclass. - If a subclass is in a different package, it can access
protected
members through inheritance, but notpackage-private
(default) members.
- Constructors in Inheritance:
- Constructors are not inherited. However, a subclass constructor can call a constructor of the superclass using the
super
keyword.
public class Dog extends Animal {
public Dog(String name) {
super(name); // Calls the constructor of the superclass
}
}
- Method Overloading:
- Subclasses can overload methods inherited from the superclass by providing additional methods with different parameter lists.
- “is-a” Relationship:
- Inheritance represents an “is-a” relationship. For example, a
Dog
is-a type ofAnimal
.
Inheritance in Java promotes code reuse, allowing you to build on existing classes and create more specialized classes. It’s a powerful mechanism for modeling relationships between classes and implementing polymorphism (the ability to use different objects through a common interface).