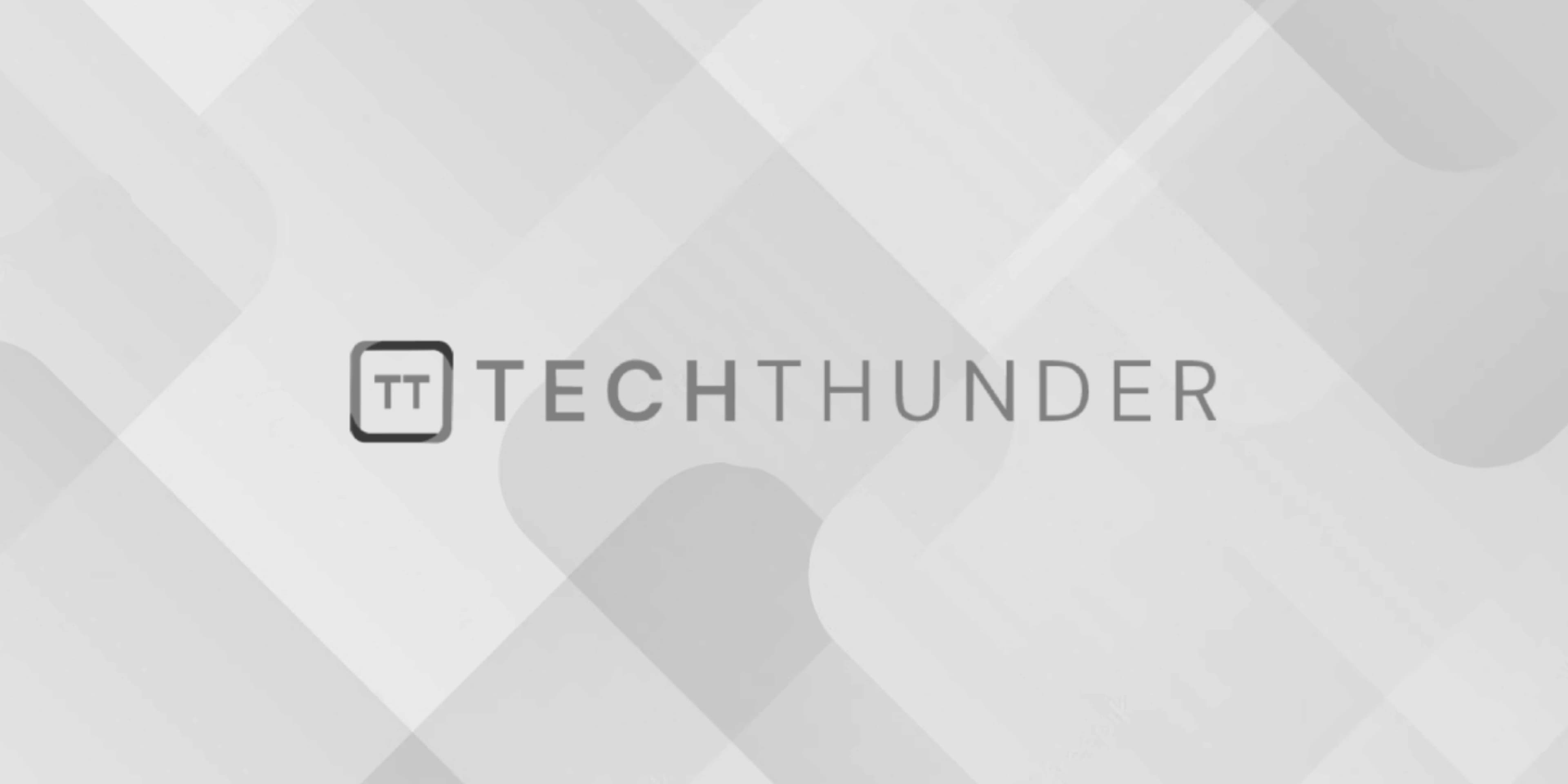
192 views
Java Naming Convention
Naming conventions in Java are established guidelines for naming various program elements, such as classes, variables, methods, and packages. Adhering to these conventions makes your code more readable and understandable by other developers, and it helps maintain consistency in Java projects. Here are the commonly followed Java naming conventions:
- Class Names:
- Class names should be written in CamelCase (or PascalCase) style.
- Names should start with an uppercase letter.
- Class names should be nouns and should represent objects or concepts.
class MyClass {
// Class members and methods here
}
- Interface Names:
- Interface names should also be in CamelCase.
- Interface names often start with an uppercase ‘I’ (e.g.,
Serializable
,Runnable
).
interface MyInterface {
// Interface methods here
}
- Method Names:
- Method names should be in camelCase.
- Names should start with a lowercase letter.
- Method names should be verbs or action-oriented, indicating what the method does.
void calculateTotalPrice() {
// Method implementation
}
- Variable Names:
- Variable names should be in camelCase.
- Names should start with a lowercase letter.
- Variable names should be descriptive and meaningful.
int itemCount;
String customerName;
- Constant Names:
- Constant names should be in uppercase.
- Words are separated by underscores.
- Constants should be declared as
static final
variables.
static final int MAX_VALUE = 100;
- Package Names:
- Package names should be in lowercase.
- Package names should be unique and should follow the reverse domain name convention.
package com.example.myapp;
- Enum Names:
- Enum names should be in CamelCase.
- Enum types should represent a group of related constants.
enum DaysOfWeek {
MONDAY, TUESDAY, WEDNESDAY, // ...
}
- Parameter Names:
- Parameter names should be in camelCase.
- Names should be meaningful and self-explanatory.
void printDetails(String firstName, String lastName) {
// Method implementation
}
- Local Variable Names:
- Local variable names should be in camelCase.
- Names should be concise yet descriptive.
int result = calculateSum();
- Class Member Prefixes:
- Some developers use prefixes for class members to indicate their visibility, such as
m_
for private members ors_
for static members. This is not a standard convention but can be a matter of personal or team preference.
- Some developers use prefixes for class members to indicate their visibility, such as
private int m_privateField;
public static String s_staticField;
Adhering to these naming conventions is not only a best practice but also essential for writing clean and maintainable Java code. It ensures that your code is consistent and easily comprehensible by both you and other developers who may work on the project.