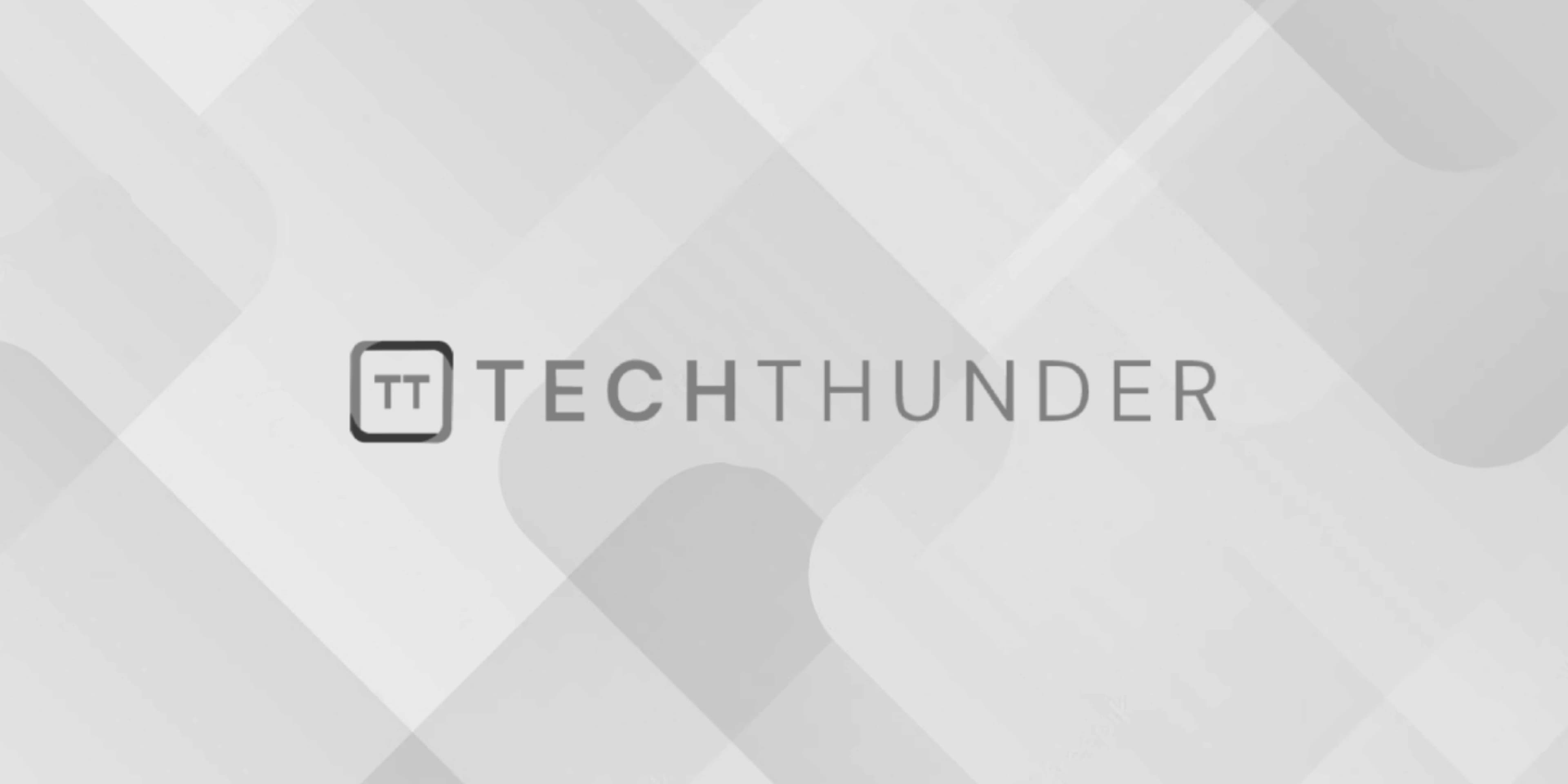
Java Math class
The java.lang.Math
class in Java is a built-in class that provides a set of methods for performing various mathematical operations. These methods are implemented as static methods, meaning they can be invoked directly on the Math
class without the need to create an instance of it. The Math
class is part of the Java standard library and is often used for performing common mathematical calculations. Here are some of the commonly used methods from the Math
class:
- Trigonometric Functions:
Math.sin(double a)
: Returns the sine of an angle in radians.Math.cos(double a)
: Returns the cosine of an angle in radians.Math.tan(double a)
: Returns the tangent of an angle in radians.Math.asin(double a)
: Returns the arcsine (inverse sine) in radians.Math.acos(double a)
: Returns the arccosine (inverse cosine) in radians.Math.atan(double a)
: Returns the arctangent (inverse tangent) in radians.Math.atan2(double y, double x)
: Returns the angle theta from the conversion of rectangular coordinates (x, y) to polar coordinates (r, theta).
- Exponential and Logarithmic Functions:
Math.exp(double a)
: Returns the base of the natural logarithm (e) raised to the power of a.Math.log(double a)
: Returns the natural logarithm (base e) of a.Math.log10(double a)
: Returns the base-10 logarithm of a.Math.pow(double a, double b)
: Returns a raised to the power of b.
- Rounding and Absolute Values:
Math.abs(double a)
: Returns the absolute value of a number.Math.ceil(double a)
: Returns the smallest integer greater than or equal to a.Math.floor(double a)
: Returns the largest integer less than or equal to a.Math.round(double a)
: Rounds a floating-point value to the nearest integer.
- Square Root and Exponentiation:
Math.sqrt(double a)
: Returns the square root of a.Math.cbrt(double a)
: Returns the cube root of a.
- Minimum and Maximum:
Math.min(double a, double b)
: Returns the smaller of two values.Math.max(double a, double b)
: Returns the larger of two values.
- Random Number Generation:
Math.random()
: Returns a pseudo-random double value between 0.0 (inclusive) and 1.0 (exclusive).
- Constants:
Math.PI
: A constant representing the value of π (pi).Math.E
: A constant representing the base of the natural logarithm (e).
- Hypotenuse Length:
Math.hypot(double x, double y)
: Returns the length of the hypotenuse of a right triangle with legs of length x and y.
These are some of the common mathematical operations provided by the Math
class. It’s a useful class for performing mathematical calculations in Java programs. Note that the Math
class methods are static, so you can call them directly using the class name, for example, Math.sqrt(25)
to calculate the square root of 25.