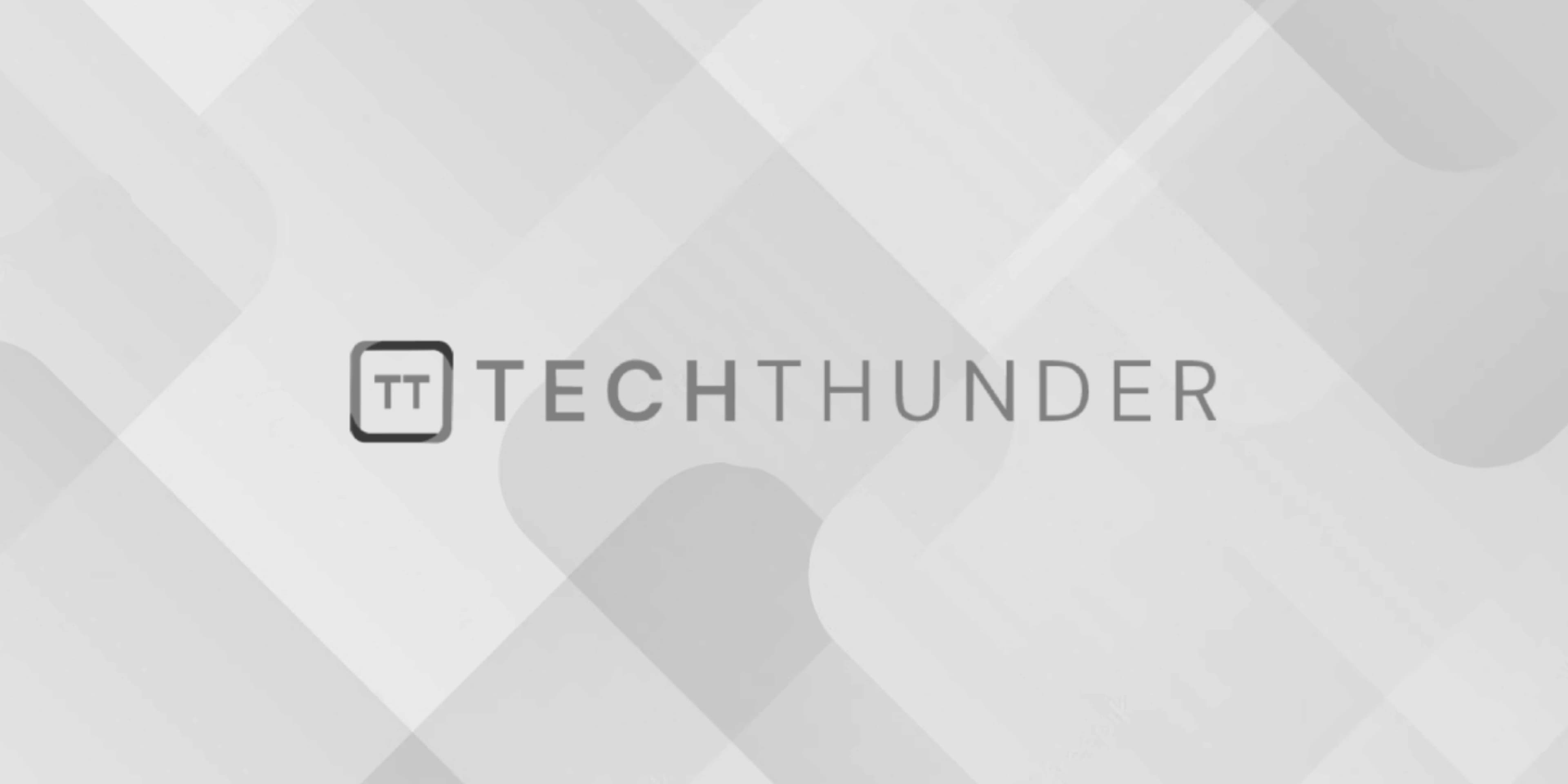
Java Object and Class
The Java object is an instance of a class. The class is a blueprint or template that defines the structure and behavior of objects. Objects are used to model real-world entities and their interactions in a program. Let’s explore the concepts of classes and objects in Java:
1. Class:
- A class is a user-defined data type that defines the properties (attributes) and behaviors (methods) of objects. It serves as a blueprint for creating objects.
- Classes are defined using the
class
keyword followed by the class name. Class names should follow Java naming conventions. - Attributes (instance variables) represent the state of an object, and methods define its behavior.
- Here’s an example of a simple Java class:
public class Car {
// Attributes
String make;
String model;
int year;
// Method
void start() {
System.out.println("The car is starting.");
}
}
2. Object:
- An object is an instance of a class, created based on the blueprint defined by the class. It represents a specific entity or occurrence.
- To create an object, you use the
new
keyword followed by the class constructor (the class name). - You can access the attributes and methods of an object using the dot notation.
- Here’s how you create and use objects:
public class Main {
public static void main(String[] args) {
// Creating objects
Car myCar = new Car();
Car anotherCar = new Car();
// Accessing attributes and methods of objects
myCar.make = "Toyota";
myCar.model = "Camry";
myCar.year = 2022;
myCar.start();
System.out.println("Make: " + myCar.make);
System.out.println("Model: " + myCar.model);
}
}
In this example, we created two objects of the Car
class: myCar
and anotherCar
. We assigned values to the attributes of myCar
and called its start
method. Each object maintains its own state (attribute values), and methods can be called on individual objects.
Java’s object-oriented nature allows you to model complex systems by defining classes that represent different components, and you can create instances of these classes as needed. Classes and objects are fundamental concepts that facilitate code organization, reusability, and the modeling of real-world entities in your Java programs.